The lowest common ancestor (LCA) of two nodes U and V in a tree is the deepest node that has both U and V as descendants.
A binary search tree (BST) is recursively defined as a binary tree which has the following properties:
- The left subtree of a node contains only nodes with keys less than the node's key.
- The right subtree of a node contains only nodes with keys greater than or equal to the node's key.
- Both the left and right subtrees must also be binary search trees.
Given any two nodes in a BST, you are supposed to find their LCA.
Input Specification:
Each input file contains one test case. For each case, the first line gives two positive integers: M (≤ 1,000), the number of pairs of nodes to be tested; and N (≤ 10,000), the number of keys in the BST, respectively. In the second line, N distinct integers are given as the preorder traversal sequence of the BST. Then M lines follow, each contains a pair of integer keys U and V. All the keys are in the range of int.
Output Specification:
For each given pair of U and V, print in a line LCA of U and V is A.
if the LCA is found and A
is the key. But if A
is one of U and V, print X is an ancestor of Y.
where X
is A
and Y
is the other node. If U or V is not found in the BST, print in a line ERROR: U is not found.
or ERROR: V is not found.
or ERROR: U and V are not found.
.
Sample Input:
6 8
6 3 1 2 5 4 8 7
2 5
8 7
1 9
12 -3
0 8
99 99
Sample Output:
LCA of 2 and 5 is 3.
8 is an ancestor of 7.
ERROR: 9 is not found.
ERROR: 12 and -3 are not found.
ERROR: 0 is not found.
ERROR: 99 and 99 are not found.
Solution:
这道题给出一个重大的提示,就是SBT,题目说明是SBT不是让你自己去兴奋的去重建这棵树【我当时就是这么想的,也这样做了】,而是让你从中发现根节点与左右孩子节点的大小关系,然后从中找到突破口
我开始是重建了二叉树,然后DFS来找到两个节点的最低公共节点,然而。。。。。超时了
聪明的做法就是从前序遍历中找到突破口【我当时想了,但没有找到规律】
首先,使用map来记录哪些节点是存在的,用来判断不存在的节点
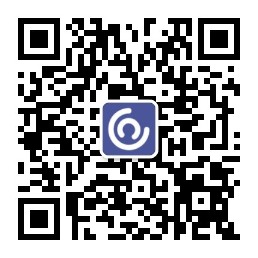
然后,遍历前序数组,当节点a ,与查询节点 u,v存在关系:(U<=a && a>=v)||(v<=a && u>=a), 那么u,v的最低公共节点就是a!!!!!
~~~~~~~~~~~
1 #include <iostream> 2 #include <vector> 3 #include <unordered_map> 4 using namespace std; 5 int n, m; 6 vector<int>pre; 7 unordered_map<int, bool>map; 8 int main() 9 { 10 cin >> m >> n; 11 pre.resize(n); 12 for (int i = 0; i < n; ++i) 13 { 14 cin >> pre[i]; 15 map[pre[i]] = true; 16 } 17 while (m--) 18 { 19 int a, b; 20 cin >> a >> b; 21 if (map[a] != true && map[b] != true) 22 printf("ERROR: %d and %d are not found.\n", a, b); 23 else if (map[a] != true) 24 printf("ERROR: %d is not found.\n", a); 25 else if (map[b] != true) 26 printf("ERROR: %d is not found.\n", b); 27 else 28 { 29 int k = 0; 30 for (k = 0; k < n; ++k) 31 if (a <= pre[k] && pre[k] <= b || b <= pre[k] && pre[k] <= a) 32 break; 33 if (pre[k] == a) 34 printf("%d is an ancestor of %d.\n", a, b); 35 else if (pre[k] == b) 36 printf("%d is an ancestor of %d.\n", b, a); 37 else 38 printf("LCA of %d and %d is %d.\n", a, b, pre[k]); 39 } 40 } 41 return 0; 42 }