Tensor类的成员函数dim()可以返回张量的维度,shape属性与成员函数size()返回张量的具体维度分量,如下代码定义了一个两行三列的张量:
f = torch.randn(2, 3)
print(f.dim())
print(f.size())
print(f.shape)
输出结果:
2
torch.Size([2, 3])
torch.Size([2, 3])
dim=0的标量
维度为0的Tensor为标量,标量一般用在Loss这种地方。如下代码定义了一个标量:
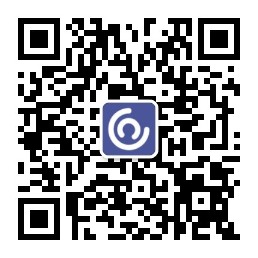
import torch
a = torch.tensor(1.6) # dimension为0就是标量了
print(a, a.type())
# 一些检验维度的方法
print(a.dim())
print(a.shape, a.size())
print(len(a.shape), len(a.size()))
输出结果:
tensor(1.6000) torch.FloatTensor
0
torch.Size([]) torch.Size([])
0 0
定义标量的方式很简单,只要在tensor函数中传入一个标量初始化的值即可,注意是具体的数据。
注意:
Pytorch中的torch.tensor()是一个函数,函数的原形如下:
torch.tensor(data, dtype=None, device=None, requires_grad=False)
其中data可以是:list、tuple、NumPy ndarray、scalar和其他类型。torch.tensor会从data中的数据部分做拷贝(而不是直接引用),根据原始数据类型生成相应的torch.LongTensor、torch.FloatTensor和torch.DoubleTensor。
而torch.Tensor()是Pytorch中的一个类,是默认张量类型torch.FloatTensor()的别名。
Tensor与tensor的区别,具体可参考https://blog.csdn.net/tfcy694/article/details/85338745
注意一点,torch.tensor()参数接收的是具体的数据,而torch.Tensor()参数既可以接收数据也可以接收维度分量也就是shape。
dim=1的张量
dim=1的Tensor一般用在Bais这种地方,或者神经网络线性层的输入Linear Input,例如MINST数据集的一张图片用shape=[784]的Tensor来表示。
dim=1相当于只有一个维度,但是这个维度上可以有多个分量(就像一维数组一样),一维的张量实现方法有很多,下面是三种实现:
import torch
import numpy as np
def printMsg(k):
"""输出Tensor的信息,维度,shape"""
print(k, k.dim(), k.size(), k.shape)
# 1.通过torch.tensor(), 参数传入一个list构造dim=1的Tensor
a = torch.tensor([1.1])
printMsg(a)
b = torch.tensor([1.1, 2.2])
printMsg(b)
print("-" * 20)
# 2.通过torch.Tensor(), 随机构造dim=1的Tensor
# 这里传入的是shape=1,有1个元素
c = torch.FloatTensor(1)
printMsg(c)
# 这里传入的是shape=2,有2个元素
d = torch.FloatTensor(2)
printMsg(d)
print("-" * 20)
# 3.从numpy构造dim=1的Tensor
e = np.ones(2)
print(e)
e = torch.from_numpy(e)
printMsg(e)
输出结果:
tensor([1.1000]) 1 torch.Size([1]) torch.Size([1])
tensor([1.1000, 2.2000]) 1 torch.Size([2]) torch.Size([2])
--------------------
tensor([1.5056e-38]) 1 torch.Size([1]) torch.Size([1])
tensor([0., 0.]) 1 torch.Size([2]) torch.Size([2])
--------------------
[1. 1.]
tensor([1., 1.], dtype=torch.float64) 1 torch.Size([2]) torch.Size([2])
dim=2的张量
dim=2的张量一般用在带有batch的Linear Input,例如MNIST数据集的k张图片如果放再一个Tensor里,那么shape=[k,784]。
如下示例:
import torch
# dim=2,shape=[2,3],随机生成Tensor
a = torch.FloatTensor(2, 3)
print(a.shape)
print(a.shape[0])
print(a.shape[1])
print(a.size())
print(a.size(0))
print(a.size(1))
输出结果:
torch.Size([2, 3])
2
3
torch.Size([2, 3])
2
3
从上面可以看出,可以取到Tensor每一维度的分量 。
dim=3的张量
dim=3的张量很适合用于RNN和NLP,如20句话,每句话10个单词,每个单词用100个分量的向量表示,得到的Tensor就是shape=[20,10,100]。
示例代码:
import torch
# dim=3,shape=[1,2,3],随机取0~1之间的数
a = torch.rand(1, 2, 3)
print(a)
print(a.dim())
print(a.shape)
print(a.size())
b = a[0] # 在第一个维度上取,得到的就是shape=[2,3]的dim=2的Tensor
print(b.dim())
print(b.shape)
print(b.size())
# 将torch.Size()转换为python列表的形式
list(a.shape)
输出结果:
tensor([[[0.3503, 0.2163, 0.7880],
[0.0933, 0.0372, 0.9077]]])
3
torch.Size([1, 2, 3])
torch.Size([1, 2, 3])
2
torch.Size([2, 3])
torch.Size([2, 3])
[1, 2, 3]
dim=4的张量
dim=4的张量适合用于CNN表示图像,例如100张MNIST手写数据集的灰度图(通道数为1,如果是RGB图像通道数就是3),每张图高=28像素,宽=28像素,所以这个Tensor的shape=[100,1,28,28],也就是一个batch的数据维度:[batch_size,channel,height,width] 。
如下构建一个shape=[2, 3, 28, 28]的Tensor:
import torch
a = torch.rand(2, 3, 28, 28)
print(a)
print(a.shape)
输出结果:
tensor([[[[0.0788, 0.1869, 0.2921, ..., 0.0653, 0.3229, 0.1024],
[0.5636, 0.4804, 0.6982, ..., 0.3832, 0.9984, 0.5522],
[0.5650, 0.8758, 0.2636, ..., 0.5024, 0.5592, 0.2962],
...,无锡看男科医院哪家好 https://yyk.familydoctor.com.cn/20612/
[0.6311, 0.8914, 0.1481, ..., 0.9255, 0.4388, 0.6771],
[0.1691, 0.6803, 0.0111, ..., 0.8962, 0.4907, 0.7369],
[0.7360, 0.3643, 0.9757, ..., 0.6954, 0.5979, 0.2019]],
[[0.1387, 0.1379, 0.7730, ..., 0.3476, 0.6346, 0.5387],
[0.2940, 0.2296, 0.5864, ..., 0.0558, 0.4093, 0.4563],
[0.1498, 0.1705, 0.4542, ..., 0.0370, 0.5867, 0.4178],
...,
[0.6814, 0.3024, 0.8031, ..., 0.0425, 0.4695, 0.3253],
[0.5721, 0.9202, 0.1709, ..., 0.7571, 0.6944, 0.7455],
[0.4426, 0.3546, 0.9652, ..., 0.2672, 0.0161, 0.3755]],
[[0.1455, 0.2020, 0.2242, ..., 0.5136, 0.5918, 0.9750],
[0.1039, 0.1641, 0.1945, ..., 0.1314, 0.6887, 0.2439],
[0.0275, 0.1987, 0.5682, ..., 0.0732, 0.7392, 0.8445],
...,
[0.0180, 0.7265, 0.0541, ..., 0.0939, 0.4592, 0.7898],
[0.2253, 0.2115, 0.6485, ..., 0.0314, 0.0185, 0.5788],
[0.4660, 0.9765, 0.1458, ..., 0.3520, 0.7162, 0.4895]]],
[[[0.3837, 0.1531, 0.9617, ..., 0.3220, 0.3959, 0.6391],
[0.4072, 0.0711, 0.5841, ..., 0.9417, 0.5799, 0.9794],
[0.0307, 0.1862, 0.0766, ..., 0.8336, 0.6978, 0.4468],
...,
[0.8934, 0.0480, 0.9964, ..., 0.8817, 0.3746, 0.6780],
[0.7201, 0.5030, 0.2582, ..., 0.9542, 0.8955, 0.0750],
[0.5892, 0.8647, 0.3251, ..., 0.5489, 0.5575, 0.3004]],
[[0.0630, 0.1473, 0.7858, ..., 0.3695, 0.2419, 0.4843],
[0.7736, 0.6974, 0.8900, ..., 0.3137, 0.4747, 0.9298],
[0.8021, 0.3490, 0.8568, ..., 0.5600, 0.2382, 0.0054],
...,
[0.4725, 0.7952, 0.5935, ..., 0.3612, 0.9396, 0.2763],
[0.3715, 0.8464, 0.0258, ..., 0.2218, 0.0808, 0.9323],
[0.2634, 0.8852, 0.7021, ..., 0.4925, 0.6998, 0.0013]],
[[0.7005, 0.2593, 0.1891, ..., 0.2242, 0.9181, 0.4466],
[0.2316, 0.1313, 0.6365, ..., 0.2096, 0.4597, 0.0361],
[0.2662, 0.8333, 0.4361, ..., 0.5952, 0.0086, 0.8168],
...,
[0.0641, 0.5702, 0.9628, ..., 0.0303, 0.9381, 0.9755],
[0.6629, 0.5515, 0.5912, ..., 0.5070, 0.1345, 0.2626],
[0.6316, 0.1251, 0.1719, ..., 0.6973, 0.2206, 0.2461]]]])
torch.Size([2, 3, 28, 28])
计算Tensor中元素的数目
示例代码:
import torch
a = torch.rand(10, 1, 28, 28)
print(a.numel()) # number of element 10*1*28*28=7840
输出结果:
7840