Best Time to Buy and Sell Stock with Cooldown
题目等级:Medium
题目描述:
Say you have an array for which the ith element is the price of a given stock on day i.
Design an algorithm to find the maximum profit. You may complete as many transactions as you like (ie, buy one and sell one share of the stock multiple times) with the following restrictions:
- You may not engage in multiple transactions at the same time (ie, you must sell the stock before you buy again).
- After you sell your stock, you cannot buy stock on next day. (ie, cooldown 1 day)
Example:
Input: [1,2,3,0,2]
Output: 3
Explanation: transactions = [buy, sell, cooldown, buy, sell]
题意:给定一个整数数组,其中第 i 个元素代表了第 i 天的股票价格 。设计一个算法计算出最大利润。在满足以下约束条件下,你可以尽可能地完成更多的交易,但是有两个条件:再次买入之前必须卖出、卖出后第二天无法买入,有一天的冷冻期。
解题思路(动态规划):
又开始买卖股票了。。。
前面我们依次处理了买卖股票的四道题目:分别限制交易一次、交易无数次、交易两次、交易k次。这里是无数次情况的一个变形,加入了一个冷冻期。
解题方法同样还是动态规划,这里没有交易次数的限制,所以我们定义状态比较方便,对于动态规划,这里每一天是一个阶段,每一阶段的决策就是:每天决定买、卖还是冷冻,而每一天有两个状态:手里持有股票、手里没有股票(包括了冷冻期这种情况)。
所以可以定义两个状态变量:
sell[i]:表示在第i天结束后手里没有股票的情况下,获得的最大收益。
hold[i]:表示在第i天结束后手里仍然持有股票的情况下获得的最大收益。
那么,对于这两个状态,如果当天结束时仍然持有股票,那么有两种可能:(1)今天刚买的,那么说明前一天必须冷冻,再往前一天结束时手里没有股票,即hold[i]=sell[i-2]-prices[i]
. (2)以前就买了,今天啥也没干,也没买也没卖,换句话说就是前一天结束的时候手里已经有了,即:hold[i]=hold[i-1]
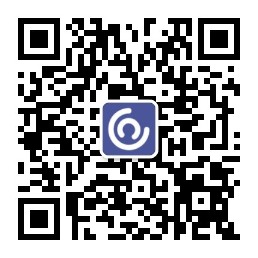
如果当天结束的时候手里没有股票,那么说明也有两种可能:(1)今天刚卖了, 也就是说前一天结束的时候手里还是持有股票的,所以:sell[i]=hold[i-1]+prices[i]
.(2)以前就卖了,今天啥也没干,那说明前一天结束的时候手里已经没有股票了,所以:sell[i]=sell[i-1]
.
综合起来,就可以得到递推关系式:
hold[i]=max(sell[i-2]-prices[i],hold[i-1])
sell[i]=max(hold[i-1]+prices[i],sell[i-1])
另外还有一点需要注意的是:初始条件,第一天的时候不可能卖出,也不可能冷冻,第一天一定会买入,这实际上还是贪心思想的一种体现,第一天可以买一定是会买的,在处理不限次数的交易时,已经证明了这种贪心思想的正确性,所以sell[0]=0
,而hold[0]=-prices[0]
.
最后,根据以上分析给出以下代码:
class Solution {
public int maxProfit(int[] prices) {
if(prices==null || prices.length==0)
return 0;
int len=prices.length;
int[] sell=new int[len];
int[] hold=new int[len];
sell[0]=0;
hold[0]=-prices[0];
for(int i=1;i<len;i++){
sell[i]=Math.max(sell[i-1],hold[i-1]+prices[i]);
hold[i]=Math.max(hold[i-1],(i>1?sell[i-2]:0)-prices[i]); //这里i-2注意防止越界
}
return sell[len-1];
}
}
时间复杂度:O(n),空间复杂度:O(2n)