Poj 1094 拓扑排序Kahn
Sorting It All Out
Time Limit: 1000MS | Memory Limit: 10000K | |
---|---|---|
Total Submissions: 41332 | Accepted: 14478 |
Description
An ascending sorted sequence of distinct values is one in which some form of a less-than operator is used to order the elements from smallest to largest. For example, the sorted sequence A, B, C, D implies that A < B, B < C and C < D. in this problem, we will give you a set of relations of the form A < B and ask you to determine whether a sorted order has been specified or not.
Input
Input consists of multiple problem instances. Each instance starts with a line containing two positive integers n and m. the first value indicated the number of objects to sort, where 2 <= n <= 26. The objects to be sorted will be the first n characters of the uppercase alphabet. The second value m indicates the number of relations of the form A < B which will be given in this problem instance. Next will be m lines, each containing one such relation consisting of three characters: an uppercase letter, the character "<" and a second uppercase letter. No letter will be outside the range of the first n letters of the alphabet. Values of n = m = 0 indicate end of input.
Output
For each problem instance, output consists of one line. This line should be one of the following three:
Sorted sequence determined after xxx relations: yyy...y.
Sorted sequence cannot be determined.
Inconsistency found after xxx relations.
where xxx is the number of relations processed at the time either a sorted sequence is determined or an inconsistency is found, whichever comes first, and yyy...y is the sorted, ascending sequence.
Sample Input
4 6
A<B
A<C
B<C
C<D
B<D
A<B
3 2
A<B
B<A
26 1
A<Z
0 0
Sample Output
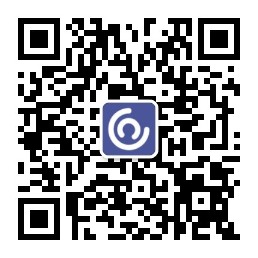
Sorted sequence determined after 4 relations: ABCD.
Inconsistency found after 2 relations.
Sorted sequence cannot be determined.
题目大意:给你多组边让你判断是否能够确定一个拓扑排序,这也就意味着我们的排序只能有唯一的一种,不能有多种方案,也就是说这里并不保证给我们的是一个DAG图。而要求我们自己判断
这里就要用到拓扑排序的一个经典算法Kahn:
摘一段维基百科上关于Kahn算法的伪码描述:
L← Empty list that will contain the sorted elements
S ← Set of all nodes with no incoming edges
while S is non-empty do
remove a node n from S
insert n into L
foreach node m with an edge e from nto m do
remove edge e from thegraph
ifm has no other incoming edges then
insert m into S
if graph has edges then
return error (graph has at least onecycle)
else
return L (a topologically sortedorder)
不难看出该算法的实现十分直观,关键在于需要维护一个入度为0的顶点的集合:
每次从该集合中取出(没有特殊的取出规则,随机取出也行,使用队列/栈也行,下同)一个顶点,将该顶点放入保存结果的List中。
紧接着循环遍历由该顶点引出的所有边,从图中移除这条边,同时获取该边的另外一个顶点,如果该顶点的入度在减去本条边之后为0,那么也将这个顶点放到入度为0的集合中。然后继续从集合中取出一个顶点…………
当集合为空之后,检查图中是否还存在任何边,如果存在的话,说明图中至少存在一条环路。不存在的话则返回结果List,此List中的顺序就是对图进行拓扑排序的结果。
代码如下:
#include<vector>
#include<queue>
#include<cstdio>
#include<cstring>
using namespace std;
const int maxn = 27;
int n,m;
vector<int> G[maxn];
int in[maxn],deg[maxn];
char ans[maxn];
int cnt;
void init() {
for(int i = 0;i < n;i++) G[i].clear();
memset(in,0,sizeof(in));
}
bool check(int u,int v) {
for(int i = 0;i < G[u].size();i++) if(G[u][i] == v) return true;
return false;
}
//传入当前边数
int topsort(int s) {
for(int i = 0;i < n;i++) deg[i] = in[i];
cnt = 0;
int flag = 1;
memset(ans,0,sizeof(ans));
queue<int> Q;
while(!Q.empty()) Q.pop();
for(int i = 0;i < n;i++) {
if(deg[i] == 0) Q.push(i);
}
//if(Q.size() > 1) flag = 0; !!!判断是否有多种情况
while(!Q.empty()) {
if(Q.size() > 1) flag = 0;
int u = Q.front();Q.pop();
ans[cnt++] = u + 'A';
int len = G[u].size();
for(int i = 0;i < len;i++) {
int v = G[u][i];
deg[v]--;
s--;
if(deg[v] == 0) {
Q.push(v);
}
}
}
if(s != 0) return 2;
else if(cnt == n && flag) return 3;
else return 1;
}
int main() {
while(scanf("%d%d",&n,&m) == 2) {
if(n == 0 && m == 0) break;
init();
char s[5];
int flag,ok = 0,k;
for(int i = 0;i < m;i++) {
scanf("%s",s);
if(ok) continue;
int u = s[0] - 'A',v = s[2] - 'A';
if(check(u,v)) continue;
in[v]++;
G[u].push_back(v);
flag = topsort(i+1);
if(flag == 3) {
ok = 1;
printf("Sorted sequence determined after %d relations: %s.\n",i+1,ans);
}
else if(flag == 2) {
ok = 1;
printf("Inconsistency found after %d relations.\n",i+1);
}
}
if(flag == 1) printf("Sorted sequence cannot be determined.\n");
}
return 0;
}