题目来源:http://poj.org/problem?id=1094
问题描述
Sorting It All Out
Time Limit: 1000MS |
Memory Limit: 10000K |
|
Total Submissions: 39161 |
Accepted: 13808 |
Description
An ascending sorted sequence of distinct values is one in which some form of a less-than operator is used to order the elements from smallest to largest. For example, the sorted sequence A, B, C, D implies that A < B, B < C and C < D. in this problem, we will give you a set of relations of the form A < B and ask you to determine whether a sorted order has been specified or not.
Input
Input consists of multiple problem instances. Each instance starts with a line containing two positive integers n and m. the first value indicated the number of objects to sort, where 2 <= n <= 26. The objects to be sorted will be the first n characters of the uppercase alphabet. The second value m indicates the number of relations of the form A < B which will be given in this problem instance. Next will be m lines, each containing one such relation consisting of three characters: an uppercase letter, the character "<" and a second uppercase letter. No letter will be outside the range of the first n letters of the alphabet. Values of n = m = 0 indicate end of input.
Output
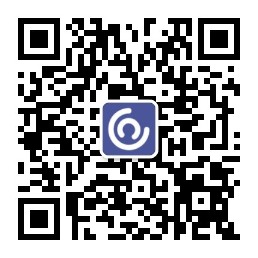
For each problem instance, output consists of one line. This line should be one of the following three:
Sorted sequence determined after xxx relations: yyy...y.
Sorted sequence cannot be determined.
Inconsistency found after xxx relations.
where xxx is the number of relations processed at the time either a sorted sequence is determined or an inconsistency is found, whichever comes first, and yyy...y is the sorted, ascending sequence.
Sample Input
4 6
A<B
A<C
B<C
C<D
B<D
A<B
3 2
A<B
B<A
26 1
A<Z
0 0
Sample Output
Sorted sequence determined after 4 relations: ABCD.
Inconsistency found after 2 relations.
Sorted sequence cannot be determined.
Source
East Central North America 2001
------------------------------------------------------------
题意
给定一组字母间两两顺序关系,问是否能确定这些字母的唯一顺序。如果能确定,输出前几条关系就能确定;如果矛盾,输出前几条关系处发生矛盾;如果不能确定,输出不能确定。
一些题目中没有说但很重要的约定:
1. 如果前x条关系就能确定唯一顺序,但第y条(y>x)关系开始又产生了矛盾,此时按“前x条关系能确定唯一顺序”处理;
2. 如果既发生矛盾又不能确定关系,优先输出矛盾。
------------------------------------------------------------
思路
本题需要判断是否成环,用基于入度的拓扑排序算法。
1. 找到图中入度为0的点作为拓扑排序的起点(忽略孤立点,若有孤立点则一定不能确定唯一顺序,但仍有可能是有环)
2. 将该点的所有后继的入度-1(相当于删除该节点)
3. 循环“步骤1-步骤2”n次,某次循环如果不存在入度为0的点则说明有环(“矛盾”),直接退出循环;如果存在不止一个入度为0且不孤立的点,则一定不能确定唯一顺序,但仍有可能有环
------------------------------------------------------------
代码
// 拓扑排序:基于入度的实现
// 找到图中入度为0的点作为拓扑排序的起点
// 将该点的后继节点的入度-1(相当于删除该点),再遍历全图找入度为0的点
// 如此循环
// 注意:
// 1. 优先判断是否成环,再判断是否可以确定拓扑顺序
// 2. 如果已经可以确定拓扑顺序,即使后面新增的语句的加入使得图成环,也不管了
#include<cstdio>
#include<vector>
#include<cstring>
const int NMAX = 30;
std::vector<int> E[NMAX]; // 邻接表
int indegree[NMAX]; // 入度数组
bool vis[NMAX]; // 是否访问数组
void clear_case() // 每算完一个测试用例清空邻接表和入度数组
{
for (int i = 0; i < NMAX; i++)
{
E[i].clear();
}
memset(indegree, 0, sizeof(indegree));
memset(vis, 0, sizeof(vis));
}
int TopoSort(int n, std::vector<int> &res) // res: 拓扑排序结果数组
{
res.clear(); // 清空结果数组
memset(indegree, 0, sizeof(indegree)); // 清空入度数组
memset(vis, 0, sizeof(vis)); // 清空访问标记
int i, j, k, u, ucnt = 0, ans = 0;
for (i=0; i<n; i++) // 遍历每条边计算每个节点的入度
{
for (j=0; j<E[i].size(); j++)
{
indegree[E[i].at(j)]++;
}
}
for (k=0; k<n; k++)
{
ucnt = 0;
for (i=0; i<n; i++)
{
if (indegree[i] == 0 && !vis[i])
{
u = i;
ucnt ++;
}
}
if (ucnt == 0) // 找不到入度为0的节点
{
return -1; // 有环
}
else if (ucnt > 1) // 入度为0的节点多于1个
{
ans = 1; // 不能确定拓扑关系,但可能有环,不能返回,要继续计算
}
vis[u] = 1;
res.push_back(u);
if (E[u].empty())
{
continue;
}
for (j=0; j<E[u].size(); j++)
{
if (!vis[E[u].at(j)])
{
indegree[E[u].at(j)]--;
}
}
}
return ans;
}
int main()
{
#ifndef ONLINE_JUDGE
freopen("1094.txt", "r", stdin);
#endif
int n, k, i, ans;
char str[4];
bool has_print = false;
std::vector<int> res;
std::vector<int>::iterator it;
while (scanf("%d%d", &n, &k))
{
if (n==0 && k==0)
{
break;
}
has_print = false;
clear_case(); // 清空全局变量
for (i=0; i<k; i++)
{
scanf("%s", str);
E[str[0]-'A'].push_back(str[2]-'A');
ans = TopoSort(n, res);
if (ans == 0 && !has_print)
{
printf("Sorted sequence determined after %d relations: ", i+1);
for (it = res.begin(); it != res.end(); it++)
{
printf("%c", (*it) + 'A');
}
printf(".\n");
has_print = true;
}
else if (ans == -1 && !has_print)
{
printf("Inconsistency found after %d relations.\n", i+1);
has_print = true;
}
}
if (ans == 1 && !has_print)
{
printf("Sorted sequence cannot be determined.\n");
}
}
return 0;
}