Time Limit: 1000MS |
|
Memory Limit: 65536K |
Description
Due to recent rains, water has pooled in various places in Farmer John's field, which is represented by a rectangle of N x M (1 <= N <= 100; 1 <= M <= 100) squares. Each square contains either water ('W') or dry land ('.'). Farmer John would like to figure out how many ponds have formed in his field. A pond is a connected set of squares with water in them, where a square is considered adjacent to all eight of its neighbors.
Given a diagram of Farmer John's field, determine how many ponds he has.
Input
* Line 1: Two space-separated integers: N and M
* Lines 2..N+1: M characters per line representing one row of Farmer John's field. Each character is either 'W' or '.'. The characters do not have spaces between them.
Output
* Line 1: The number of ponds in Farmer John's field.
Sample Input
10 12 W........WW. .WWW.....WWW ....WW...WW. .........WW. .........W.. ..W......W.. .W.W.....WW. W.W.W.....W. .W.W......W. ..W.......W.
Sample Output
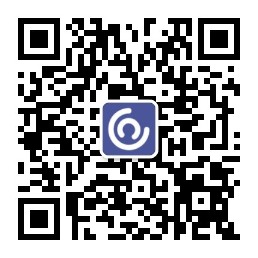
3
Hint
OUTPUT DETAILS: There are three ponds: one in the upper left, one in the lower left,and one along the right side.
解题思路
简单的DFS入门,写好之后一直WA,然后发现scanf的一个注意事项之前一直没注意,cin读入字符会自动忽略换行符和空格,但是scanf不会,所以涉及到换行和空格的时候要用getchar()跳过换行符和空格。
AC代码
#include<cstdio> #include<cstring> using namespace std; const int N = 105; char map[N][N]; int vis[N][N];//访问标记 int n, m; int dx[8] = { -1,0,1,-1,1,-1,0,1 }; int dy[8] = { 1,1,1,0,0,-1,-1,-1 };//结点周边从上到下,从左到右八个点 int ans = 0;//湖数 bool valid(int x, int y) { return (x >= 0 && y >= 0 && x < n && y < m); } void DFS(int x, int y) { vis[x][y] = 1;//已访问 for (int i = 0; i < 8; i++) { int newx = x + dx[i]; int newy = y + dy[i]; if (valid(newx, newy)) { if (map[newx][newy] == 'W'&&vis[newx][newy] == -1) DFS(newx, newy); } } } int main() { memset(vis, -1, sizeof(vis)); scanf("%d%d", &n, &m); getchar(); for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { scanf("%c", &map[i][j]); } getchar(); } for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { if (map[i][j] == 'W'&&vis[i][j] == -1) { ans++; DFS(i, j); } } } printf("%d", ans); return 0; }
更简单的解决:不用vis数组,直接将访问过的“W”变成“.”。