优先级队列
代码实现
class PrioLink{
class Entry{
int data;
Entry next;
int prio;
public Entry(){
data = -1;
next = null;
prio = -1;
}
public Entry(int data,int prio){
this.data = data;
this.prio = prio;
this.next = null;
}
}
private Entry head = null;
public PrioLink(){
this.head = new Entry();
}
/**
* 按照优先级进行插入
*/
public void insert(int data,int prio){
Entry entry = new Entry(data,prio);
Entry cur = this.head;
if(cur.next == null){
cur.next = entry;
}else{
while(cur.next.prio>prio){
cur = cur.next;
}
entry.next = cur.next;
cur.next = entry;
}
}
/**
* 出队
*/
public void pop(){
Entry cur = this.head;
if(cur.next == null){
return;
}
Entry del = cur.next;
cur.next = del.next;
del = null;
}
public void show(){
Entry cur = this.head.next;
while(cur!=null){
System.out.println(cur.data+" ");
cur = cur.next;
}
System.out.println();
}
}
public class Test2 {
public static void main(String[] args) {
PrioLink p1 = new PrioLink();
p1.insert(10, 1);
p1.insert(30, 3);
p1.insert(50, 2);
p1.show();
p1.pop();
p1.show();
}
}
运行结果
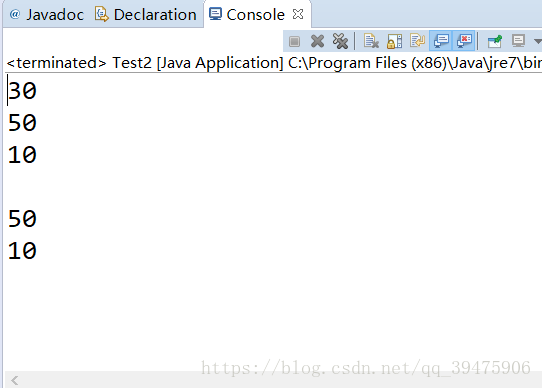