1 题目
Given a binary tree, determine if it is height-balanced.
For this problem, a height-balanced binary tree is defined as:
a binary tree in which the depth of the two subtrees of everynode never differ by more than 1.
Example 1:
Given the following tree [3,9,20,null,null,15,7]
:
3
/ \
9 20
/ \
15 7
Return true.
Example 2:
Given the following tree [1,2,2,3,3,null,null,4,4]
:
1
/ \
2 2
/ \
3 3
/ \
4 4
Return false.
2 尝试解
扫描二维码关注公众号,回复:
6150029 查看本文章
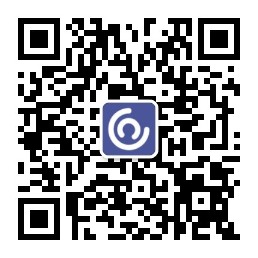
2.1 分析
判断是否为平衡二叉树,即任意节点左右子树高度相差不差过2。可以让每一个节点的为以节点为根节点的树的高度,然后遍历每个节点,看其是否平衡。
2.2 代码
class Solution {
public:
bool isBalanced(TreeNode* root) {
if(root == NULL) return true;
depth(root);
queue<TreeNode*> traverse;
traverse.push(root);
while(!traverse.empty()){
TreeNode* cur = traverse.front();
traverse.pop();
if(abs((cur->left?cur->left->val:0)-(cur->right?cur->right->val:0))>=2) return false;
if(cur->left != NULL) traverse.push(cur->left);
if(cur->right != NULL) traverse.push(cur->right);
}
return true;
}
int depth(TreeNode* root){
if(root == NULL) return 0;
if(root->left == NULL && root->right == NULL) {
root->val = 1;
return 1;
}
root->val = 1 + max(depth(root->left),depth(root->right));
return root->val;
}
};
3 标准解
class solution {
public:
int depth (TreeNode *root) {
if (root == NULL) return 0;
return max (depth(root -> left), depth (root -> right)) + 1;
}
bool isBalanced (TreeNode *root) {
if (root == NULL) return true;
int left=depth(root->left);
int right=depth(root->right);
return abs(left - right) <= 1 && isBalanced(root->left) && isBalanced(root->right);
}
};