题目:
Given a binary tree, determine if it is height-balanced.
For this problem, a height-balanced binary tree is defined as:
a binary tree in which the depth of the two subtrees of every node never differ by more than 1.
Example 1:
Given the following tree [3,9,20,null,null,15,7]
:
3 / \ 9 20 / \ 15 7
Return true.
Example 2:
Given the following tree [1,2,2,3,3,null,null,4,4]
:
1 / \ 2 2 / \ 3 3 / \ 4 4
Return false.
扫描二维码关注公众号,回复:
2591433 查看本文章
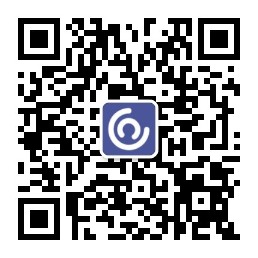
题意:
当输入的二叉树节点的左右子树的高度差超过1(>=2)时,则该二叉树是不平衡的,反之,则平衡。我同样使用回溯法进行求解,每次需要比较的是当前节点出发的左子树和右子树的高度差,如果超过1则将信号量与false,若没有则与true。
值得一提的是,当没有任何剪枝条件时,代码提交后的效率只击败10%的用户,但是我加上条件“一旦信号量变为false,则返回”后,效率击败了96+%的用户^_______^
代码:
int backtrack(TreeNode* root, int cur, bool& s) {
if(!s)
return 0;
int left = cur, right = cur;
if(root->left != NULL)
{
left = backtrack(root->left, cur + 1, s);
}
if(root->right != NULL)
{
right = backtrack(root->right, cur + 1, s);
}
s &= abs(left - right) < 2 ? true : false;
return max(left, right);
}
bool isBalanced(TreeNode* root) {
if(root == NULL)
return true;
int cur = 1;
bool s = true;
int tmp = backtrack(root, cur, s);
return s;
}
代码应该还有一些优化的地方>.< 准备睡了,晚安世界。