Sudoku
Time Limit: 2000MS | Memory Limit: 65536K | |||
Total Submissions: 25420 | Accepted: 11869 | Special Judge |
Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
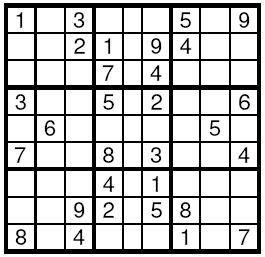
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1 103000509 002109400 000704000 300502006 060000050 700803004 000401000 009205800 804000107
Sample Output
143628579 572139468 986754231 391542786 468917352 725863914 237481695 619275843 854396127
Source
拿到这道题的时候我的想法是先记录有多少个待填数,在待填数填满之前,进行不断地循环,每次找到待填数能填的数字只有一个的去填。
对于合格的数独题来说这样是正确的,并且是唯一的答案,但是仔细观察题目,有说到若有多种答案输出其中一种即可,说明这不是严格的数独题,所以这个方法错误。
那么就只好将每次数据都填进去尝试,这里用到的方法是dfs+剪枝。
row记录每一行填过的数,col记录每一列填过的数,grid记录每一格填过的数,不难发现(x/3)*3+y/3可以代表x,y所在格子的编号。
1.一开始写完的时候忘记了dfs里面改回row col grid的值,只改了a的值,所以以后回溯注意要彻底。
2.要注意设置碰到对的答案的时候就停止回溯。
3.不可以a[x][y]!=0时就不继续递归,因为一轮完整的dfs是从左到右从上到下每一格都进行访问从而验证某种方案到底可不可。
以下是正确代码(自带测试用例):
#include <iostream> #include <cstdio> #include <cstring> using namespace std; bool row[9][10]; bool col[9][10]; bool grid[9][10]; int getgrid(int x,int y){ return (x/3)*3+y/3; } bool dfs(int x,int y,int a[9][9]){ if(x==9) return true; bool flag=false; if(a[x][y]!=0){ if(y==8) flag=dfs(x+1,0,a); else flag=dfs(x,y+1,a); } else{ int g=getgrid(x,y); for(int i=1;i<10;i++){ if(row[x][i]&&col[y][i]&&grid[g][i]){ a[x][y]=i; bool rt=row[x][i]; bool ct=col[y][i]; bool gt=grid[g][i]; row[x][i]=false; col[y][i]=false; grid[g][i]=false; if(y==8) flag=dfs(x+1,0,a); else flag=dfs(x,y+1,a); if(flag) return flag; a[x][y]=0; row[x][i]=rt; col[y][i]=ct; grid[g][i]=gt; } } } return flag; } int main() { int T; scanf("%d",&T); while(T--){ memset(row,true,sizeof(bool)*90); memset(col,true,sizeof(bool)*90); memset(grid,true,sizeof(bool)*90); int a[9][9]={{1,0,3,0,0,0,5,0,9}, {0,0,2,1,0,9,4,0,0}, {0,0,0,7,0,4,0,0,0}, {3,0,0,5,0,2,0,0,6}, {0,6,0,0,0,0,0,5,0}, {7,0,0,8,0,3,0,0,4}, {0,0,0,4,0,1,0,0,0}, {0,0,9,2,0,5,8,0,0}, {8,0,4,0,0,0,1,0,7}}; /*for(int i=0;i<9;i++){ string s; cin>>s; for(int k=0;k<9;k++){ a[i][k]=(int)s[k]-48; } }*/ for(int i=0;i<9;i++){ for(int j=0;j<9;j++){ if(a[i][j]!=0){ row[i][a[i][j]]=false; col[j][a[i][j]]=false; int g=getgrid(i,j); grid[g][a[i][j]]=false; } } } dfs(0,0,a); for(int i=0;i<9;i++){ for(int j=0;j<9;j++){ printf("%d",a[i][j]); } printf("\n"); } } return 0; }
以下是AC代码:
#include <iostream> #include <cstdio> #include <cstring> using namespace std; bool row[9][10]; bool col[9][10]; bool grid[9][10]; int getgrid(int x,int y){ return (x/3)*3+y/3; } bool dfs(int x,int y,int a[9][9]){ if(x==9) return true; bool flag=false; if(a[x][y]!=0){ if(y==8) flag=dfs(x+1,0,a); else flag=dfs(x,y+1,a); } else{ int g=getgrid(x,y); for(int i=1;i<10;i++){ if(row[x][i]&&col[y][i]&&grid[g][i]){ a[x][y]=i; bool rt=row[x][i]; bool ct=col[y][i]; bool gt=grid[g][i]; row[x][i]=false; col[y][i]=false; grid[g][i]=false; if(y==8) flag=dfs(x+1,0,a); else flag=dfs(x,y+1,a); if(flag) return flag; a[x][y]=0; row[x][i]=rt; col[y][i]=ct; grid[g][i]=gt; } } } return flag; } int main() { int T; scanf("%d",&T); while(T--){ memset(row,true,sizeof(bool)*90); memset(col,true,sizeof(bool)*90); memset(grid,true,sizeof(bool)*90); int a[9][9]; for(int i=0;i<9;i++){ string s; cin>>s; for(int k=0;k<9;k++){ a[i][k]=(int)s[k]-48; } } for(int i=0;i<9;i++){ for(int j=0;j<9;j++){ if(a[i][j]!=0){ row[i][a[i][j]]=false; col[j][a[i][j]]=false; int g=getgrid(i,j); grid[g][a[i][j]]=false; } } } dfs(0,0,a); for(int i=0;i<9;i++){ for(int j=0;j<9;j++){ printf("%d",a[i][j]); } printf("\n"); } } return 0; }
1.学会了memset的使用,虽然说这样可以
memset(row,true,sizeof(bool)*90);
但这样写起来会更方便
memset(row,true,row);
2.学会了字符串输入数字转int数组
for(int i=0;i<9;i++){ string s; cin>>s; for(int k=0;k<9;k++){ a[i][k]=(int)s[k]-48; } }
3.对于dfs有了更进一步的了解
以下是用唯一数法的WA代码:
#include <iostream> #include <cstdio> using namespace std; void afill(int a[9][9],int x,int y){ int num[10]; for(int i=0;i<10;i++) num[i]=1; for(int i=0;i<9;i++){ if(a[x][i]!=0){ num[a[x][i]]=0; } if(a[i][y]!=0){ num[a[i][y]]=0; } } for(int i=(x/3)*3;i<(x/3)*3+3;i++){ for(int j=(y/3)*3;j<(y/3)*3+3;j++){ if(a[i][j]!=0){ num[a[i][j]]=0; } } } int mark=0; int p=0; for(int i=1;i<10;i++){ if(num[i]!=0){ mark++; p=i; if(mark>1) return; } } a[x][y]=p; } int main() { int T; scanf("%d",&T); while(T--){ int a[9][9]={{1,0,3,0,0,0,5,0,9}, {0,0,2,1,0,9,4,0,0}, {0,0,0,7,0,4,0,0,0}, {3,0,0,5,0,2,0,0,6}, {0,6,0,0,0,0,0,5,0}, {7,0,0,8,0,3,0,0,4}, {0,0,0,4,0,1,0,0,0}, {0,0,9,2,0,5,8,0,0}, {8,0,4,0,0,0,1,0,7}}; int z=0; for(int i=0;i<9;i++){ //string s; //cin>>s; for(int k=0;k<9;k++){ //a[i][k]=(int)s[k]-48; if(a[i][k]==0){ z++; } } } int x=0;int y=0;int f=0; for(int k=0;k<z;k++) for(int i=0;i<9;i++){ for(int j=0;j<9;j++){ if(a[i][j]==0){ afill(a,i,j); } } } for(int i=0;i<9;i++){ for(int j=0;j<9;j++){ printf("%d",a[i][j]); } printf("\n"); } } return 0; }