版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/huidev/article/details/80213197
如果安卓自带的对话框不能满足你的需求,而你又迫切需要一个炫酷(哈哈,夸张一下,就是可以加缩放,平移等动画)的自定义对话框,可以看看这篇博客,大神可以绕过。。
先上图
对话框布局代码dialog_test.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="@color/white">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:text="查询结果"
android:textColor="@color/black"
android:textSize="18sp"
android:layout_margin="8dp"
android:layout_centerInParent="true"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
<View
android:layout_width="match_parent"
android:layout_height="0.1dp"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:background="@color/gray_very_light"/>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp">
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/meeting_img_dialog"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_centerVertical="true"
android:layout_marginRight="16dp"
android:src="@mipmap/meeting_img"
android:transitionName="details"
android:layout_marginEnd="16dp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_toEndOf="@id/meeting_img_dialog"
android:layout_toRightOf="@id/meeting_img_dialog"
android:orientation="vertical">
<TextView
android:id="@+id/meeting_name_dialog"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="@string/topic"
android:layout_margin="1dp"/>
<TextView
android:id="@+id/meeting_time_dialog"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="@string/site"
android:layout_margin="1dp"/>
<TextView
android:id="@+id/meeting_site_dialog"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="@string/start_time"
android:layout_margin="1dp"/>
<TextView
android:id="@+id/meeting_creator_dialog"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="@string/creator"
android:layout_margin="1dp"/>
</LinearLayout>
</RelativeLayout>
<View
android:layout_width="match_parent"
android:layout_height="0.1dp"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:background="@color/gray_very_light"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/cancel_dialog"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:background="@drawable/btn_bg"
android:text="@string/not"
android:textSize="16sp" />
<View
android:layout_width="0.1dp"
android:layout_height="match_parent"
android:layout_marginBottom="5dp"
android:layout_marginTop="5dp"
android:background="@color/gray_very_light" />
<Button
android:id="@+id/join_dialog"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:background="@drawable/btn_bg"
android:text="@string/join"
android:textSize="16sp" />
</LinearLayout>
</LinearLayout>
用到的CircleImageView 是一个开源项目,他可以轻松实现图片圆形化,在gradle 里添加依赖
compile 'de.hdodenhof:circleimageview:2.1.0' 就可以使用。然后就是在线性布局,相对布局,其它的也可以,自己可以自由发挥。
btn_bg.xml 用selector 来实现点击的颜色变换
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_pressed="false" android:drawable="@color/transparent"/>
<item android:state_pressed="true" android:drawable="@color/gray_very_light"/>
</selector>
写一个显示对话框的方法
/**
* 弹出对话框,让用户决定加入与否
*/
public void showDialog(){
final Dialog dialog = new Dialog(MainActivity.this);
//去掉title
dialog.requestWindowFeature(Window.FEATURE_NO_TITLE);
dialog.show();
Window window = dialog.getWindow();
// 设置布局
window.setContentView(R.layout.dialog_test);
// 设置宽高
window.setLayout(RadioGroup.LayoutParams.MATCH_PARENT, RadioGroup.LayoutParams.WRAP_CONTENT);
// 设置弹出的动画效果
window.setWindowAnimations(R.style.AnimScale);
window.setGravity(Gravity.CENTER);
ImageView img = (ImageView)window.findViewById(R.id.meeting_img_dialog);
TextView topic = (TextView)window.findViewById(R.id.meeting_name_dialog);
TextView t = (TextView)window.findViewById(R.id.meeting_time_dialog);
TextView site = (TextView)window.findViewById(R.id.meeting_site_dialog);
TextView creator = (TextView)window.findViewById(R.id.meeting_creator_dialog);
Button cancel_btn = (Button) window.findViewById(R.id.cancel_dialog);
Button join_btn = (Button) window.findViewById(R.id.join_dialog);
cancel_btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this,"不了",Toast.LENGTH_SHORT).show();
dialog.cancel();
}
});
join_btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this,"加入",Toast.LENGTH_SHORT).show();
dialog.cancel();
}
});
}
通过来为对话框添加一个缩放的动画window.setWindowAnimations(R.style.AnimScale);
需要注意的是Button cancel_btn = (Button) window.findViewById(R.id.cancel_dialog); 不要忘加window 控件的引用要依托在一个View 上的。就一个简单的Button button = (Button)findViewById(R.id.btn); 也是可以这样写的Button button = (Button)this.findViewById(R.id.btn); 只不过这个this 通常是省略掉的。Window 虽然不同于View ,但是在这里有类似的作用。忘记加window 会报错,是找不到对应的View 的。
在styles.xml 里加入
扫描二维码关注公众号,回复:
5536209 查看本文章
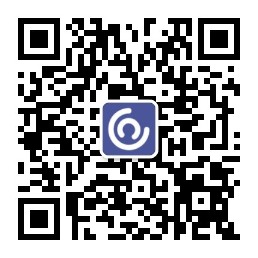
<style name="AnimScale" parent="@android:style/Animation">
<item name="android:windowEnterAnimation">@anim/scale_in</item>
<item name="android:windowExitAnimation">@anim/scale_out</item>
</style>
scale_in.xml 代码
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="100">
<scale
android:fromXScale="50%"
android:fromYScale="50%"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="100%"
android:toYScale="100%"/>
</set>
scale_out.xml 代码
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="160">
<scale
android:fromXScale="100%"
android:fromYScale="100%"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="20%"
android:toYScale="20%"/>
</set>