2019.1.18
题目描述:
Implement int sqrt(int x)
.
Compute and return the square root of x, where x is guaranteed to be a non-negative integer.
Since the return type is an integer, the decimal digits are truncated and only the integer part of the result is returned.
Example 1:
Input: 4 Output: 2
Example 2:
Input: 8 Output: 2 Explanation: The square root of 8 is 2.82842..., and since the decimal part is truncated, 2 is returned.
这道题是求一个int值的数的平方根。
解法一:
由于int开根号最大也就46340,所以在0~46340中找,若i的平方小于等于x,并且i+1的平方大于x,则返回,由于46341的平方超出了int的范围,因此在循环的最后一次是无法进行比较的,所以若是循环结束还无法返回,则在最后返回46340(即特殊情况)。
C++代码:
扫描二维码关注公众号,回复:
4969673 查看本文章
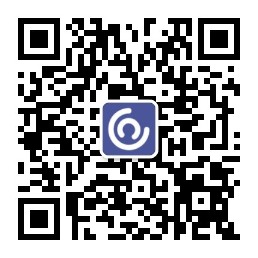
class Solution {
public:
int mySqrt(int x) {
for(int i=0;i<=46340;){
int j=i+1;
if(i*i<=x&&j*j>x)
return i;
else
i++;
}
return 46340;
}
};
执行时间:40ms,有点慢
解法二:
基本思想不变,但是查找用二分法,可以减小时间复杂度
C++代码:
class Solution {
public:
int mySqrt(int x) {
if (x <= 1) return x;
int low = 0, high = x;
while (low < high) {
int mid = low + (high - low) / 2;
if (x / mid >= mid) low = mid + 1;
else high = mid;
}
return high - 1;
}
};
执行时间:16ms,快多了
解法三:
通过查阅讨论与相关博客,得知还有一种牛顿迭代法,可以简单了解一下https://en.wikipedia.org/wiki/Integer_square_root#Using_only_integer_division
https://zh.wikipedia.org/wiki/%E7%89%9B%E9%A1%BF%E6%B3%95
C++代码:
class Solution {
public:
int mySqrt(int x) {
if (x == 0) return 0;
double res = 1, pre = 0;
while (abs(res - pre) > 1e-6) {
pre = res;
res = (res + x / res) / 2;
}
return int(res);
}
};
执行用时16ms