Given an array nums
of n integers where n > 1, return an array output
such that output[i]
is equal to the product of all the elements of nums
except nums[i]
.
Example:
Input:[1,2,3,4]
Output:[24,12,8,6]
Note: Please solve it without division and in O(n).
Follow up:
Could you solve it with constant space complexity? (The output array does not count as extra space for the purpose of space complexity analysis.)
给定长度为 n 的整数数组 nums
,其中 n > 1,返回输出数组 output
,其中 output[i]
等于 nums
中除 nums[i]
之外其余各元素的乘积。
示例:
输入:[1,2,3,4]
输出:[24,12,8,6]
说明: 请不要使用除法,且在 O(n) 时间复杂度内完成此题。
进阶:
你可以在常数空间复杂度内完成这个题目吗?( 出于对空间复杂度分析的目的,输出数组不被视为额外空间。)
116ms
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 4 var result : [Int] = [] 5 var mutiplier = 1 6 7 for num in nums 8 { 9 result.append(mutiplier) 10 mutiplier *= num 11 } 12 13 mutiplier = 1 14 var index = nums.count - 1 15 while index>=0 16 { 17 result[index] *= mutiplier 18 mutiplier *= nums[index] 19 index -= 1 20 } 21 22 return result 23 } 24 }
124ms
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 guard !nums.isEmpty else { return [] } 4 5 var output = [Int](repeating: 1, count: nums.count) 6 for i in nums.indices.dropFirst() { 7 output[i] = output[i - 1] * nums[i - 1] 8 } 9 10 var right = nums[nums.count - 1] 11 for i in nums.indices.dropLast().reversed() { 12 output[i] *= right 13 right *= nums[i] 14 } 15 16 return output 17 } 18 }
124ms
扫描二维码关注公众号,回复:
4736842 查看本文章
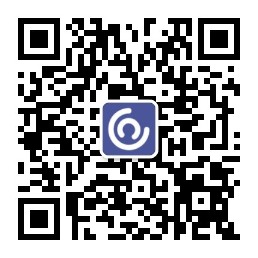
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 let count = nums.count 4 if count == 0 { 5 return [Int]() 6 } 7 if count == 1 { 8 return [1] 9 } 10 var result = nums 11 for i in 1..<count - 1 { 12 result[i] *= result[i - 1] 13 } 14 15 var temp = 1 16 for i in (1..<count).reversed() { 17 result[i] = temp * result[i - 1] 18 temp *= nums[i] 19 } 20 result[0] = temp 21 return result 22 } 23 }
125ms
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 let total = nums.filter({ $0 != 0 }).reduce(1, { $0 * $1 }) 4 let zero_count = nums.filter({ $0 == 0}).count 5 return nums.map { number -> Int in 6 if zero_count == 0 { 7 return total / number 8 } else if zero_count == 1 { 9 return number == 0 ? total : 0 10 } else { 11 return 0 12 } 13 } 14 } 15 }
136ms
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 guard nums.count > 1 else { return [0]} 4 let letfProduct = nums.reduce(into:[]) { $0.append(($0.last ?? 1) * $1) } 5 let rightProduct = Array(Array(nums.reversed().reduce(into:[]) { $0.append(($0.last ?? 1) * $1) }).reversed()) 6 var result = [Int](repeating:0, count:nums.count) 7 for i in 0..<nums.count { 8 if i == 0 { 9 result[i] = rightProduct[1] 10 } else if i == nums.count-1 { 11 result[i] = letfProduct[nums.count-2] 12 } else { 13 result[i] = letfProduct[i-1] * rightProduct[i+1] 14 } 15 } 16 return result 17 } 18 }
160ms
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 let n = nums.count 4 var result = [Int](repeating: 1, count: n) 5 guard n > 0 else { return result } 6 //设left[i]为nums[i]左边的所有元素的乘积 7 var left = [Int](repeating: 1, count: n) 8 //设right[i]为nums[i]右边的所有元素的乘积 9 var right = [Int](repeating: 1, count: n) 10 for i in 1 ..< n { 11 left[i] = left[i - 1] * nums[i - 1] 12 } 13 for i in (0 ..< n - 1).reversed() { 14 right[i] = right[i + 1] * nums[i + 1] 15 } 16 for i in 0 ..< n { 17 result[i] = left[i] * right[i] 18 } 19 return result 20 } 21 }
188ms
1 class Solution { 2 func productExceptSelf(_ nums: [Int]) -> [Int] { 3 var res = [Int]() 4 var leftValue = 1 5 for i in 0 ..< nums.count { 6 res.append(leftValue) 7 leftValue *= nums[i] 8 } 9 var rightValue = 1 10 for i in stride(from: nums.count - 1, to: -1, by: -1) { 11 res[i] = res[i] * rightValue 12 rightValue *= nums[i] 13 } 14 return res 15 } 16 }