AmazonDynamoDB被设计成用来托管的NoSQL数据库服务、可预期的性能、可实现无缝扩展性和可靠性等核心问题
DynamoDB没有Cassandra和MongoDB强大
MongoDB是文档型的数据库,可以理解为底层存储的是JSON,DynamoDB不支持JSON的
开发应用上MongoDB远胜于DynamoDB
运维的角度来讲,DynamoDB省去开发者部署/监控/维护数据库环节,给开发者节约了大量时间,
强大的扩展能力又减轻了后续运维的压力,这正是DynamoDB最大的价值所在
亚马逊网络服务(AWS)
创建表格:
import boto3
dynamodb = boto3.resource('dynamodb')
# 在 create_table 调用中,您需要指定表名称、主键属性及其数据类型,ProvisionedThroughput 参数是必填项
table = dynamodb.create_table(
TableName = 'users',
KeySchema = [
{
'AttributeName': 'username',
'KeyType': 'HASH'
}, {
'AttributeName': 'last_name',
'KeyType': 'RANGE'
},
],
AttributeDefinitions = [
{
'AttributeName': 'username',
'AttributeType': 'S'
},
{
'AttributeName': 'last_name',
'AttributeType': 'S'
},
],
ProvisionedThroughput={
'ReadCapacityUnits': 5,
'WriteCapacityUnits': 5
}
)
table.meta.client.get_waiter('table_exists').wait( TableName = 'users',)
print(table.item_count)
基本的操作:
import boto3
from boto3.dynamodb.conditions import Key, Attr
使用存在的表Table = users
dynamodb = boto3.resource(‘dynamodb’)
table = dynamodb.Table(‘users’)
print(table.creation_date_time)
创建一个新的Item
table.put_item(
Item={
‘username’: ‘janedoe’,
‘first_name’: ‘Jane’,
‘last_name’: ‘Doe’,
‘age’: 25,
‘account_type’: ‘standard_user’,
}
)
获取一个Item
response = table.get_item(
Key={
‘username’: ‘janedoe’,
‘last_name’: ‘Doe’
}
)
item = response[‘Item’]
print(item)
更新数据
table.update_item(
Key={
‘username’: ‘janedoe’,
‘last_name’: ‘Doe’
},
UpdateExpression=‘SET age = :val1’,
ExpressionAttributeValues={
‘:val1’: 26
}
)
再获取更新之后的信息
response = table.get_item(
Key={
‘username’: ‘janedoe’,
‘last_name’: ‘Doe’
}
)
item = response[‘Item’]
print(item)
删除数据
table.delete_item(
Key={
‘username’: ‘janedoe’,
‘last_name’: ‘Doe’
}
)
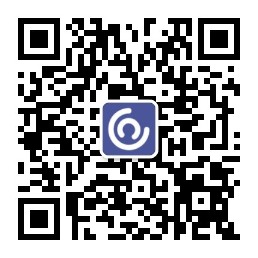
查找数据需要导入 from boto3.dynamodb.conditions import Key, Attr
response = table.query(
KeyConditionExpression=Key(‘username’).eq(‘janedoe’)
)
items = response[‘Items’]
print(items)