声明:
今天是第70道题。给定一个包含 0, 1, 2, ..., n
中 n 个数的序列,找出 0 .. n 中没有出现在序列中的那个数。以下所有代码经过楼主验证都能在LeetCode上执行成功,代码也是借鉴别人的,在文末会附上参考的博客链接,如果侵犯了博主的相关权益,请联系我删除
(手动比心ღ( ´・ᴗ・` ))
正文
题目:给定一个包含 0, 1, 2, ..., n
中 n 个数的序列,找出 0 .. n 中没有出现在序列中的那个数。
示例 1:
输入: [3,0,1] 输出: 2
示例 2:
输入: [9,6,4,2,3,5,7,0,1] 输出: 8
说明:
你的算法应具有线性时间复杂度。你能否仅使用额外常数空间来实现?
解法1。线性时间。先对nums排序,再使用枚举enumerate方法,如果key和value不相等代表缺失了与key相等的数字,代码如下。
执行用时: 52 ms, 在Missing Number的Python提交中击败了35.62% 的用户
class Solution(object):
def missingNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
# 长度为1时只可能有2种情况,就是为1或者0
if len(nums)==1:
if nums[0]==0:
return 1
if nums[0]==1:
return 0
nums.sort()
for key,val in enumerate(nums):
if key != val:
return key
else:
continue
return nums[-1]+1 # 如果当前序列不缺元素,则返回最后1个元素加1
解法2。如果缺失的数字出现在中间,那么可以利用等差数列的求和公式求出它完整时的和,再减去实际的和就是缺失的那个数字,代码如下。
执行用时: 32 ms, 在Missing Number的Python提交中击败了99.08% 的用户
class Solution(object):
def missingNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
nums_sum = sum(nums)
supposed_sum = len(nums)*(len(sum)+1)/2
return supposed_sum-nums_sum
解法3。这种方法真的精妙,如果nums一开始是无序的且肯定缺了1个数,那么假设这个数是i,放在index为len(nums)这个位置上,也就是最后1个,那么就构成了1个完整的序列,该完整序列的值和index都异或,得出结果肯定为0,套用下面的异或法则,因为完整的index和value一定相等嘛,现在把i单独抽出来,[3,1,0,i]全员异或的公式就变为:3⊕0⊕1⊕1⊕0⊕2⊕i⊕len(nums)=3⊕0⊕1⊕1⊕0⊕2⊕len(nums)⊕i=0,i=3⊕0⊕1⊕1⊕0⊕2⊕len(nums),代码实现如下。
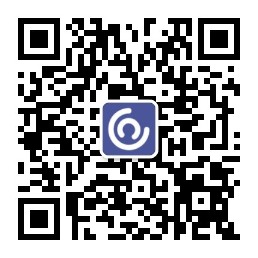
- a^b=1
- a^a=0
- a^b^a=b
执行用时: 40 ms, 在Missing Number的Python提交中击败了64.38% 的用户
class Solution(object):
def missingNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
i_index = len(nums)
for i in range(len(nums)):
i_index ^= (i^nums[i])
return i_index
解法4。用set这个数据结构,根据给定的序列,构造一个对应的完整的序列,再找2者的不同元素,pop出来正好有现成的API,代码如下。
执行用时: 40 ms, 在Missing Number的Python提交中击败了64.38% 的用户
- set(a).difference(b):返回的是集合a、b的不同元素构成的集合
- .pop():就是把返回的集合元素弹出,这里面只有1个元素,就是缺失的那个元素
class Solution(object):
def missingNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
return set(range(len(nums)+1)).difference(set(nums)).pop()
结尾
解法1:https://blog.csdn.net/qq_34364995/article/details/80699747
解法2&解法3:https://blog.csdn.net/u014251967/article/details/52473505
解法4:LeetCode