我之前写过两个接口服务user和student,现在将它完善一下
user,创建一个接口 localhost:12003/getUserList 返回一个json数据,用来做测试
UserApi.java
package com.springcloud.demo.api;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import com.springcloud.demo.domain.BaseJSON;
import com.springcloud.demo.domain.po.UserPO;
import com.springcloud.demo.server.UserService;
@RestController
public class UserApi {
@Autowired
public UserService userService;
@RequestMapping(value="/test",method=RequestMethod.GET)
public String test() {
return "Hello World";
}
@RequestMapping(value="/getUserList",method=RequestMethod.GET)
public BaseJSON getUserList() {
BaseJSON json = new BaseJSON();
List<UserPO> list = userService.getUserList();
if(list.size()<0||list==null) {
json.setCode(11);
json.setMessage("失败");
}else {
json.setResult(list);
}
return json;
}
}
Service层实现
package com.springcloud.demo.server.imp;
import java.util.ArrayList;
import java.util.List;
import org.springframework.stereotype.Service;
import com.springcloud.demo.domain.po.UserPO;
import com.springcloud.demo.server.UserService;
@Service
public class UserServiceImp implements UserService{
@Override
public List<UserPO> getUserList() {
List<UserPO> list = new ArrayList<>();
UserPO user = new UserPO();
user.setId(1);
user.setUsername("lg");
user.setPassword("123456");
list.add(user);
user.setId(2);
user.setUsername("lxy");
user.setPassword("123456");
list.add(user);
return list;
}
}
Student 调用 user的restFul接口,获取数据,然后将数据重新封装
首先我们需要注入RestTemplate,这里我们图方便加载BootMain中了,使用@LoadBalanced注解标明
package com.springcloud.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.cloud.netflix.feign.EnableFeignClients;
import org.springframework.cloud.netflix.hystrix.dashboard.EnableHystrixDashboard;
import org.springframework.context.annotation.Bean;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.client.RestTemplate;
import springfox.documentation.annotations.ApiIgnore;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Controller
@SpringBootApplication
@ApiIgnore
@EnableScheduling
@EnableDiscoveryClient
@EnableFeignClients
@EnableSwagger2
@EnableHystrixDashboard
public class BootMain {
public static void main(String[] args) {
SpringApplication.run(BootMain.class, args);
}
@RequestMapping("/help")
public String help() {
return "redirect:swagger-ui.html";
}
/**
重点看这里
*/
@Bean
@LoadBalanced
RestTemplate restTemplate() {
return new RestTemplate();
}
}
service调用实现
【注】:
1、RestTemplate 访问的URL不能携带ip和端口号,不然会报错,改成你所调用的服务在注册中心注册的名字
扫描二维码关注公众号,回复:
3335968 查看本文章
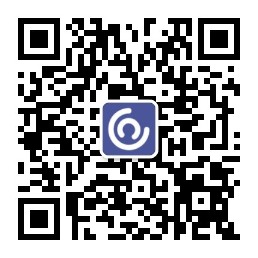
报错
No instances available for 192.168.xx.xx
No instances available for [IP]
2、使用Entity接收RestTemplate获取到的值
@Autowired
private RestTemplate rt;
//ResponseEntity<BaseJSON> re = rt.getForEntity(url, BaseJSON.class);
HttpEntity<BaseJSON> re = rt.getForEntity(url, BaseJSON.class);
BaseJSON json = re.getBody();
获取到的json就是我门的目标json对象(BaseJSON自己封装的),里面拥有从user获取到的全部数据
3、在获取对象时候,我们得到的Object无法直接转为目标对象StudentPO,我们可以先将其转为json字符串,然后再转为我们的目标对象,需要在pom.xml中加入jar包,具体java<——>操作可见:json数据对象操作
<dependency>
<groupId>net.sf.json-lib</groupId>
<artifactId>json-lib</artifactId>
<version>2.4</version>
<classifier>jdk15</classifier>
</dependency>
package com.springcloud.demo.service.imp;
import java.util.ArrayList;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import com.springcloud.demo.domain.BaseJSON;
import com.springcloud.demo.domain.po.StudentPO;
import com.springcloud.demo.domain.po.UserPO;
import com.springcloud.demo.service.StudentService;
import net.sf.json.JSONObject;
@Service
public class StudentServiceImp implements StudentService{
@Autowired
private RestTemplate rt;
@Override
public List<StudentPO> getStudentList() {
//使用RestTemplate
String url = "http://user/getUserList";
/**
* String url = "http://192.168.99.115:12001/user/getUserList";
* 报错:No instances available for [IP]
*/
//ResponseEntity<BaseJSON> re = rt.getForEntity(url, BaseJSON.class);
HttpEntity<BaseJSON> re = rt.getForEntity(url, BaseJSON.class);
BaseJSON json = re.getBody();
List<UserPO> userList = new ArrayList();
List<StudentPO> studentList = new ArrayList();
if(json.getCode()==0) {
userList = (List<UserPO>) json.getResult();
for(Object obj:userList) {
JSONObject jsonObject = JSONObject.fromObject(obj);
UserPO user = (UserPO)jsonObject.toBean(jsonObject,UserPO.class);
StudentPO student = new StudentPO();
student.setId(user.getId());
student.setName(user.getUsername());
student.setPassword(user.getUsername());
student.setGrade(1);
studentList.add(student);
}
}else {
studentList = null;
}
return studentList;
}
}