版权声明:本文为博主原创文章,未经博主允许不得转载。有事联系:[email protected] https://blog.csdn.net/qq_17550379/article/details/82815121
一个机器人位于一个 m x n 网格的左上角 (起始点在下图中标记为“Start” )。
机器人每次只能向下或者向右移动一步。机器人试图达到网格的右下角(在下图中标记为“Finish”)。
问总共有多少条不同的路径?

例如,上图是一个7 x 3 的网格。有多少可能的路径?
说明: m 和 n 的值均不超过 100。
示例 1:
输入: m = 3, n = 2
输出: 3
解释:
从左上角开始,总共有 3 条路径可以到达右下角。
1. 向右 -> 向右 -> 向下
2. 向右 -> 向下 -> 向右
3. 向下 -> 向右 -> 向右
示例 2:
输入: m = 7, n = 3
输出: 28
解题思路
这个问题和Leetcode 64:最小路径和(最详细的解法!!!) 很类似,我们同样可以通过递归解决。我们要知道左上角开始有多少路径,那么我们只需要知道左上角的→有多少路径以及↓有多少路径,然后将两者相加即可。
class Solution:
def uniquePaths(self, m, n):
"""
:type m: int
:type n: int
:rtype: int
"""
return self._uniquePaths(m, n, 0, 0)
def _uniquePaths(self, m, n, row, col):
if row == m - 1 or col == n - 1:
return 1
return self._uniquePaths(m, n, row + 1, col) +\
self._uniquePaths(m, n, row, col + 1)
但是这样做存在着大量的重复运算(在哪呢?)。我们可以通过记忆化搜索的方式来优化上面的问题。
class Solution:
def uniquePaths(self, m, n):
"""
:type m: int
:type n: int
:rtype: int
"""
mem = [[None for i in range(m)] for j in range(n)]
return self._uniquePaths(m, n, 0, 0, mem)
def _uniquePaths(self, m, n, row, col, mem):
if row == n - 1 or col == m - 1:
mem[row][col] = 1
return mem[row][col]
if row < n and col < m and mem[row][col]:
return mem[row][col]
mem[row][col] = self._uniquePaths(m, n, row + 1, col, mem) +\
self._uniquePaths(m, n, row, col + 1, mem)
return mem[row][col]
同样的,我们也可以反过来思考问题,直接从终点出发,而不是从起点出发。
扫描二维码关注公众号,回复:
3303830 查看本文章
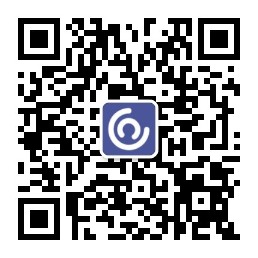
class Solution:
def uniquePaths(self, m, n):
"""
:type m: int
:type n: int
:rtype: int
"""
mem = [[None for i in range(m)] for j in range(n)]
for i in range(m):
mem[n - 1][i] = 1
for i in range(n):
mem[i][m - 1] = 1
for i in range(n - 2, -1, -1):
for j in range(m - 2, -1, -1):
mem[i][j] = mem[i + 1][j] + mem[i][j + 1]
return mem[0][0]
其实这个问题最简单的思路是通过排列组合解决,实际上这是一个组合问题。对于一个mxn
的网格来说,我们要知道有多少种路径,那么只要知道m+n-2
个step
中,向下的n-1
个step
有多少种组合即可,也就是
。
import math
class Solution:
def uniquePaths(self, m, n):
"""
:type m: int
:type n: int
:rtype: int
"""
mole = math.factorial(m)
deno = math.factorial(n - r) * math.factorial(r)
return int(mole/deno)
上述代码在使用c++
写的时候存在数据溢出的风险,最好使用long long
进行数据存储。另外,一个最简单的做法是,使用c++
的库函数lgamma
,并且lgamma(n)=log((n-1)!)
#include <math.h>
class Solution
{
public:
int uniquePaths(int m, int n)
{
return int(exp(lgamma(m+n-1)-lgamma(m)-lgamma(n))+0.5);
}
};
我将该问题的其他语言版本添加到了我的GitHub Leetcode
如有问题,希望大家指出!!!