To Fill or Not to Fill
Time Limit: 2 Seconds Memory Limit: 65536 KB
With highways available, driving a car from Hangzhou to any other city is easy. But since the tank capacity of a car is limited, we have to find gas stations on the way from time to time. Different gas station may give different price. You are asked to carefully design the cheapest route to go.
Input :
Each input file contains one test case. For each case, the first line contains 4 positive numbers: C~max~ (<= 100), the maximum capacity of the tank; D (<=30000), the distance between Hangzhou and the destination city; D~avg~ (<=20), the average distance per unit gas that the car can run; and N (<= 500), the total number of gas stations. Then N lines follow, each contains a pair of non-negative numbers: P~i~, the unit gas price, and D~i~ (<=D), the distance between this station and Hangzhou, for i=1,...N. All the numbers in a line are separated by a space.
Output :
For each test case, print the cheapest price in a line, accurate up to 2 decimal places. It is assumed that the tank is empty at the beginning. If it is impossible to reach the destination, print "The maximum travel distance = X" where X is the maximum possible distance the car can run, accurate up to 2 decimal places.
Sample Input
50 1300 12 8
6.00 1250
7.00 600
7.00 150
7.10 0
7.20 200
7.50 400
7.30 1000
6.85 300
50 1300 12 2
7.10 0
7.00 600
Sample Output
749.17
The maximum travel distance = 1200.00
题意:给你油箱容量Cmax,总路程D,单位油可行驶路程Da,站点数N,计算到达目的地需要的最小花费。油箱最开始是空的
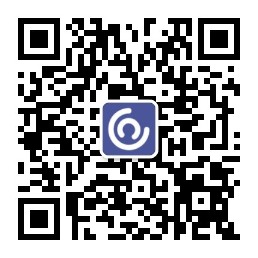
思路:贪心。
①按距离从小到大排序。
②初始点是否有站点,没有站点无法起步,输出0;
③当前站不加油,是否能直接到达终点。
④判断当前站点可达范围内是否存在比当前油价低的站点。
若有,1)油量足够,直接行驶到比当前油价低的站点。2)油量不足,加油到恰好足够行驶到比当前 油价低的站点。
若无,找到可达范围内油价最低的站点,并且在当前站点把油加满(如果当前站点可以直接到达终点,就直接加油到终点)。
若当前可达范围内没有站点,答案为当前站点距离+可行驶最大距离。
⑥当前站点为最后一站,要么直接去终点,要么到不了终点。
PS:可能思考不够仔细,见谅。
AC代码:
#include <bits/stdc++.h>
using namespace std;
const int maxn =550;
struct Station{
double p;
double d;
}s[maxn];
bool cmp(Station a,Station b)
{
return a.d<b.d;
}
int main()
{
int Cmax,D,Da,N;
while(scanf("%d%d%d%d",&Cmax,&D,&Da,&N)!=EOF)
{
for(int i=0;i<N;i++)
{
scanf("%lf%lf",&s[i].p,&s[i].d);
}
sort(s,s+N,cmp);
// 现在还有的油量 现在距离终点 已经行驶
double NowGas =0,Nowdis = D,dis=0;
double price=0;
int can=1;
if(s[0].d!=0){
printf("The maximum travel distance = 0.00\n"); /*寸步难行*/
continue;
}
for(int i=0;i<N;i++)
{
//printf("现在在加油站 : %d ,price = %.2lf , NowGas = %.2lf\n",i,price,NowGas);
//当前在i加油站
//下面没有加油站了
// 要么加油到达终点
// 要么加满走到哪算哪
//当前站可以直接到终点
if(s[i].d+NowGas*Da>=D)break;
if(i==N-1)
{
if(s[i].d+Cmax*Da<D)
{
dis = s[i].d+Cmax*Da;
can=0;
break;
}
else
{
price += (1.0*(D-s[i].d-NowGas*Da)/Da)*s[i].p;;
break;
}
}
else
{
double To = s[i].d+Cmax*Da;//最远可达
double CheapPrice = s[i+1].d;
int Cheapid = i+1;
if(s[i+1].d>To) /*后来意识到 中间路程就可能不可达*/
{
can=0;
dis=s[i].d+Cmax*Da;
break;
}
for(int j=i+1;s[j].d<=To;j++)
{
if(s[j].p<CheapPrice){
CheapPrice=s[j].p;
Cheapid = j;
}
if(s[j].p<s[i].p)break;
if(j+1>=N)break;
}
//printf("Cheapid is =%d\n",Cheapid);
if(CheapPrice<s[i].p){
//可达价格比当前站点便宜
//可以直接去便宜的站 不加油
if(s[i].d+NowGas*Da>=s[Cheapid].d)
{
NowGas-= 1.0*(s[Cheapid].d-s[i].d)/Da;
i=Cheapid-1;
}
else //要加油才能到达便宜的站 加恰好达到的油
{
price += (1.0*(s[Cheapid].d-s[i].d-NowGas*Da)/Da)*s[i].p;
NowGas=0;
i=Cheapid-1;
}
}
else{
//价格比当前站点贵 但是是最小的
//当前站点加满 或 当前站能到终点
if(s[i].d+Cmax*Da>=D)
{
price+= (1.0*(D-s[i].d-NowGas*Da)/Da)*s[i].p;
break;
}
else{
price +=(Cmax-NowGas)*s[i].p;
NowGas=Cmax;
NowGas -=(1.0*(s[Cheapid].d-s[i].d)/Da);
i=Cheapid-1;
}
}
}
}
if(can)printf("%.2lf\n",price);
else printf("The maximum travel distance = %.2lf\n",dis);
}
}