题目描述
一棵树上有n个节点,编号分别为1到n,每个节点都有一个权值w。我们将以下面的形式来要求你对这棵树完成
一些操作: I. CHANGE u t : 把结点u的权值改为t II. QMAX u v: 询问从点u到点v的路径上的节点的最大权值
III. QSUM u v: 询问从点u到点v的路径上的节点的权值和 注意:从点u到点v的路径上的节点包括u和v本身
输入
输入的第一行为一个整数n,表示节点的个数。接下来n – 1行,每行2个整数a和b,表示节点a和节点b之间有
一条边相连。接下来n行,每行一个整数,第i行的整数wi表示节点i的权值。接下来1行,为一个整数q,表示操作
的总数。接下来q行,每行一个操作,以“CHANGE u t”或者“QMAX u v”或者“QSUM u v”的形式给出。
对于100%的数据,保证1<=n<=30000,0<=q<=200000;中途操作中保证每个节点的权值w在-30000到30000之间。
输出
对于每个“QMAX”或者“QSUM”的操作,每行输出一个整数表示要求输出的结果。
样例输入
4
1 2
2 3
4 1
4 2 1 3
12
QMAX 3 4
QMAX 3 3
QMAX 3 2
QMAX 2 3
QSUM 3 4
QSUM 2 1
CHANGE 1 5
QMAX 3 4
CHANGE 3 6
QMAX 3 4
QMAX 2 4
QSUM 3 4
样例输出
4
1
2
2
10
6
5
6
5
16
题解
树链剖分的模版题啊。
扫描二维码关注公众号,回复:
2762522 查看本文章
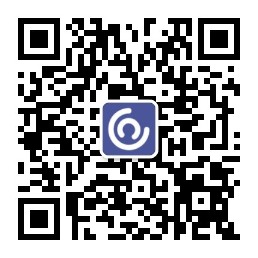
#include<cmath> #include<cstdio> #include<cstdlib> #include<cstring> #include<iostream> #include<algorithm> using namespace std; #define ll long long const int maxn=30000+10; struct SegmentTree{ int l,r,maxx,sum; }st[maxn*4]; int fir[maxn],next[maxn*2],to[maxn*2],ecnt; int top[maxn],fa[maxn],dep[maxn],sz[maxn],tree[maxn],pre[maxn],son[maxn]; int a[maxn],num,q,n; char s[10]; void add_edge(int u,int v){ next[++ecnt]=fir[u];fir[u]=ecnt;to[ecnt]=v; } void dfs1(int x){ dep[x]=dep[fa[x]]+1; sz[x]=1; for(int i=fir[x];i;i=next[i]) if(to[i]!=fa[x]){ fa[to[i]]=x; dfs1(to[i]); sz[x]+=sz[to[i]]; if(!son[x]||sz[son[x]]<sz[to[i]]) son[x]=to[i]; } } void dfs2(int x,int y){ top[pre[tree[x]=++num]=x]=y; if(!son[x]) return ; dfs2(son[x],y); for(int i=fir[x];i;i=next[i]) if(to[i]!=fa[x]&&to[i]!=son[x]) dfs2(to[i],to[i]); } void pushup(int root){ st[root].sum=st[root*2].sum+st[root*2+1].sum; st[root].maxx=max(st[root*2].maxx,st[root*2+1].maxx); } void build(int root,int l,int r){ st[root].l=l;st[root].r=r; if(l==r) st[root].sum=st[root].maxx=a[pre[l]]; else{ int m=l+r>>1; build(root*2,l,m);build(root*2+1,m+1,r); pushup(root); } } void change(int root,int x,int val){ if(st[root].l==st[root].r) st[root].sum+=val,st[root].maxx+=val; else{ int m=st[root].l+st[root].r>>1; if(x<=m) change(root*2,x,val); else change(root*2+1,x,val); pushup(root); } } int q_max(int root,int l,int r){ if(st[root].l>r||st[root].r<l) return -1e9; if(st[root].l>=l&&st[root].r<=r) return st[root].maxx; else return max(q_max(root*2,l,r),q_max(root*2+1,l,r)); } int q_sum(int root,int l,int r){ if(st[root].l>r||st[root].r<l) return 0; if(st[root].l>=l&&st[root].r<=r) return st[root].sum; else return q_sum(root*2,l,r)+q_sum(root*2+1,l,r); } int find_max(int x,int y){ int f1=top[x],f2=top[y],ans=-1e9; while(f1!=f2){ if(dep[f1]<dep[f2]) swap(f1,f2),swap(x,y); ans=max(ans,q_max(1,tree[f1],tree[x])); x=fa[f1];f1=top[x]; } if(dep[x]>dep[y]) swap(x,y); ans=max(ans,q_max(1,tree[x],tree[y])); return ans; } int find_sum(int x,int y){ int f1=top[x],f2=top[y],ans=0; while(f1!=f2){ if(dep[f1]<dep[f2]) swap(f1,f2),swap(x,y); ans+=q_sum(1,tree[f1],tree[x]); x=fa[f1];f1=top[x]; } if(dep[x]>dep[y]) swap(x,y); ans+=q_sum(1,tree[x],tree[y]); return ans; } template<typename T>void read(T& aa) { char cc; ll ff;aa=0;cc=getchar();ff=1; while((cc<'0'||cc>'9')&&cc!='-') cc=getchar(); if(cc=='-') ff=-1,cc=getchar(); while(cc>='0'&&cc<='9') aa=aa*10+cc-'0',cc=getchar(); aa*=ff; } int main(){ read(n);int x,y; for(int i=1;i<n;i++){ read(x),read(y); add_edge(x,y);add_edge(y,x); } for(int i=1;i<=n;i++) read(a[i]); dfs1(1); dfs2(1,1); build(1,1,n); read(q); while(q--){ scanf("%s",s); read(x),read(y); if(s[1]=='H'){ change(1,tree[x],y-a[x]); a[x]=y; } else printf("%d\n",s[1]=='M'?find_max(x,y):find_sum(x,y)); } return 0; }