一个轮廓一般对应一系列的点。也就是图像中的一条直线。
1. 寻找轮廓:findContours()函数
函数原型
void findContours(InputOutputArray image,OutputArrayOfArrays contours,OutputArray hierarchy,int mode,int method, Point offset=Point)
第四个参数,int类型的mode,轮廓检索模式
第五个参数
2. 绘制轮廓函数drawContours()
函数原型:
void drawCountours(InputOutputArray image,InputArrayOfArrays contours, int contourIdx,const Scalar & color,int thickness = 1, int lineType = 8, InputArrayhierarchy = noArray(),int maxLevel = INT_MAX,Point offset = Point())
3. 基础示例程序:轮廓查找
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <vector>
using namespace cv;
using namespace std;
int main()
{
//载入原始图
Mat srcImage = imread("1.jpg", 0);
//显示原始图
imshow("原始图", srcImage);
//初始化结果图
Mat dstImage = Mat::zeros(srcImage.rows, srcImage.cols, CV_8UC3);
//阈值化
srcImage = srcImage > 119;
imshow("阈值化后的原始图", srcImage);
//定义轮廓和层次结构
vector<vector<Point> >contours;
vector<Vec4i> hierarchy;
//查找轮廓
findContours(srcImage, contours, hierarchy, RETR_CCOMP, CHAIN_APPROX_SIMPLE);
//遍历所有的顶层的轮廓,随机颜色绘制每个连接组件颜色
int index = 0;
for (; index >= 0; index = hierarchy[index][0])
{
Scalar color(rand() & 255, rand() & 255, rand() & 255);
drawContours(dstImage, contours, index, color, FILLED, 8, hierarchy);
}
imshow("轮廓图", dstImage);
waitKey(0);
return 0;
}
扫描二维码关注公众号,回复:
2709606 查看本文章
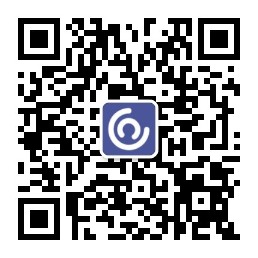
4. 综合示例
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <iostream>
using namespace cv;
using namespace std;
//定义宏部分
#define WINDOW_NAME1 "【原始图窗口】"
#define WINDOW_NAME2 "【轮廓图】"
//定义全局变量
Mat g_srcImage;
Mat g_grayImage;
int g_nThresh = 80;
int g_nThresh_max = 255;
RNG g_rng(12345);
Mat g_cannyMat_output;
vector<vector<Point> > g_vContours;
vector<Vec4i> g_vHierarchy;
//全局函数
void on_ThreshChange(int, void *);
int main()
{
//改变console字体颜色
system("color 1F");
//加载源图像
g_srcImage = imread("1.jpg", 1);
if (!g_srcImage.data)
{
printf("读取图片错误~!\n");
return false;
}
//转成灰度并模糊降噪
cvtColor(g_srcImage, g_grayImage, COLOR_BGR2GRAY);
blur(g_grayImage, g_grayImage, Size(3, 3));
//创建窗口
namedWindow(WINDOW_NAME1, WINDOW_AUTOSIZE);
imshow(WINDOW_NAME1, g_srcImage);
//创建轨迹条
createTrackbar("canny 阈值", WINDOW_NAME1, &g_nThresh, g_nThresh_max, on_ThreshChange);
on_ThreshChange(0, 0);
waitKey(0);
return 0;
}
//on_ThreshChange()函数
void on_ThreshChange(int, void *)
{
//调用canny算子检测边缘
Canny(g_grayImage, g_cannyMat_output, g_nThresh, g_nThresh * 2, 3);
//寻找轮廓
findContours(g_cannyMat_output, g_vContours, g_vHierarchy, RETR_TREE, CHAIN_APPROX_SIMPLE, Point(0, 0));
Mat drawing = Mat::zeros(g_cannyMat_output.size(), CV_8UC3);
for (int i = 0; i < g_vContours.size(); i++)
{
Scalar color = Scalar(g_rng.uniform(0, 255), g_rng.uniform(0, 255), g_rng.uniform(0, 255));
drawContours(drawing, g_vContours, i, color, 2, 8, g_vHierarchy, 0, Point());
}
imshow(WINDOW_NAME2, drawing);
}