写流程和代码之前呢,先简单的谈谈在写完线性回归和逻辑回归之后一点小体会,其实这些在tensorflow中玩无非都是一堆东西先定义好(初始化),然后在run一下初始化,接着定义模型的输出和损失函数,通过梯度下降,最小化损失函数,不断的迭代优化,在tensorflow中玩还是比较简单的,都是分模块的,这个是我人为划分的额,不多说,上代码和注释
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
import input_data #mnist数据集中py文件,后期用来下载训练集和测试集的,当然你也可以自己提前下载好,放在指定文件夹里
mnist = input_data.read_data_sets('data/', one_hot=True)
trainimg = mnist.train.images
trainlabel = mnist.train.labels
testimg = mnist.test.images
testlabel = mnist.test.labels
print ("MNIST loaded") #通过打印可以看到刚刚定义的input_data的作用,在mnist中又调用了train和test模块
打印如下:
Extracting data/train-images-idx3-ubyte.gz Extracting data/train-labels-idx1-ubyte.gz Extracting data/t10k-images-idx3-ubyte.gz Extracting data/t10k-labels-idx1-ubyte.gz MNIST loaded
print (trainimg.shape)
print (trainlabel.shape)
print (testimg.shape)
print (testlabel.shape)
#print (trainimg)
print (trainlabel[1]) #打印一些训练集和测试集数据 的形状和相关信息的其中0-9用one-hot编码向量的格式表示
打印如下:
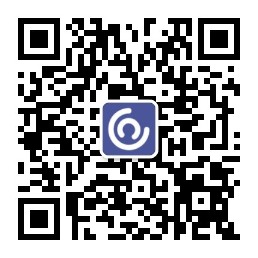
(55000, 784) (55000, 10) (10000, 784) (10000, 10) [0. 0. 0. 1. 0. 0. 0. 0. 0. 0.]
下面开始进入框架的中心环节了
x = tf.placeholder("float", [None, 784]) #先在会话框里占个坑,none暂时不指定大小,后面再给它赋值,784是像素
y = tf.placeholder("float", [None, 10]) # None is for infinite #这里的10 是从0-9 的类别数目
W = tf.Variable(tf.zeros([784, 10]))#这个784就理解为784个权重值,10理解为后面用softmax输出的10个得分值
b = tf.Variable(tf.zeros([10]))#b呢 先初始化为10个0,当然了这样为了简单才这样初始化的,一般比较好的做法还是初始化为高斯分布的
# LOGISTIC REGRESSION MODEL
actv = tf.nn.softmax(tf.matmul(x, W) + b) #因为是多分类,我们用softmax这个分类器做10分类
# COST FUNCTION
cost = tf.reduce_mean(-tf.reduce_sum(y*tf.log(actv), reduction_indices=1)) #定义损失函数,加在一起然后求个均值
# OPTIMIZER
learning_rate = 0.01#指定梯度下降的学习率
optm = tf.train.GradientDescentOptimizer(learning_rate).minimize(cost)#最小哈损失函数,优化模型
# PREDICTION
pred = tf.equal(tf.argmax(actv, 1), tf.argmax(y, 1)) #相等就为1,不等就为0
# ACCURACY
accr = tf.reduce_mean(tf.cast(pred, "float"))#布尔型转换成0和1的序列
# INITIALIZER
init = tf.global_variables_initializer()#定义初始化
下面一段代码解释一下几个相关的函数:
sess = tf.InteractiveSession() # tf.Session()和tf.InteractiveSession()的区别?唯一的区别在于:tf.InteractiveSession()加载它自身作为默认构建的session,tensor.eval()和operation.run()取决于默认的session.
换句话说:InteractiveSession
输入的代码少,原因就是它允许变量不需要使用session就可以产生结构
arr = np.array([[31, 23, 4, 24, 27, 34],
[18, 3, 25, 0, 6, 35],
[28, 14, 33, 22, 20, 8],
[13, 30, 21, 19, 7, 9],
[16, 1, 26, 32, 2, 29],
[17, 12, 5, 11, 10, 15]])
#tf.rank(arr).eval()#输出矩阵的维数
#tf.shape(arr).eval()#输出矩阵的形状
#tf.argmax(arr, 0).eval()#指定参数返回
# 0 -> 31 (arr[0, 0])
# 3 -> 30 (arr[3, 1])
# 2 -> 33 (arr[2, 2])
tf.argmax(arr, 1).eval()
# 5 -> 34 (arr[0, 5])
# 5 -> 35 (arr[1, 5])
# 2 -> 33 (arr[2, 2])
training_epochs = 100#设置这个数进行100次迭代,这个可以作为参数进行修改,然后模型的准确度
batch_size = 100#取一簇的数据100个进行训练
display_step = 5#预先设置一个参数,作为后面打印输出备用的
# SESSION
sess = tf.Session()
sess.run(init)#以上常规操作run初始化
# MINI-BATCH LEARNING
for epoch in range(training_epochs):
avg_cost = 0.#初始cost为0
num_batch = int(mnist.train.num_examples/batch_size)#计算有多少簇
for i in range(num_batch):
batch_xs, batch_ys = mnist.train.next_batch(batch_size)
sess.run(optm, feed_dict={x: batch_xs, y: batch_ys})#把数据喂给模型,让模型跑,注意喂得时候的定义结构
feeds = {x: batch_xs, y: batch_ys}
avg_cost += sess.run(cost, feed_dict=feeds)/num_batch#把每一簇最小化的损失值加在一起
# DISPLAY
if epoch % display_step == 0:#在一个簇中每个5个打印一次
feeds_train = {x: batch_xs, y: batch_ys}
feeds_test = {x: mnist.test.images, y: mnist.test.labels}
train_acc = sess.run(accr, feed_dict=feeds_train)
test_acc = sess.run(accr, feed_dict=feeds_test)#上面的几行代码和上面的结构和功能都一样
print ("Epoch: %03d/%03d cost: %.9f train_acc: %.3f test_acc: %.3f"
% (epoch, training_epochs, avg_cost, train_acc, test_acc))
print ("DONE")
打印结果:
Epoch: 000/100 cost: 1.176812563 train_acc: 0.850 test_acc: 0.853 Epoch: 005/100 cost: 0.440904123 train_acc: 0.910 test_acc: 0.895 Epoch: 010/100 cost: 0.383327348 train_acc: 0.870 test_acc: 0.905 Epoch: 015/100 cost: 0.357198811 train_acc: 0.890 test_acc: 0.908 Epoch: 020/100 cost: 0.341463638 train_acc: 0.870 test_acc: 0.913 Epoch: 025/100 cost: 0.330522055 train_acc: 0.890 test_acc: 0.914 Epoch: 030/100 cost: 0.322366304 train_acc: 0.950 test_acc: 0.916 Epoch: 035/100 cost: 0.315936294 train_acc: 0.910 test_acc: 0.917 Epoch: 040/100 cost: 0.310739638 train_acc: 0.930 test_acc: 0.918 Epoch: 045/100 cost: 0.306370187 train_acc: 0.910 test_acc: 0.919 Epoch: 050/100 cost: 0.302655735 train_acc: 0.920 test_acc: 0.919 Epoch: 055/100 cost: 0.299448199 train_acc: 0.920 test_acc: 0.919 Epoch: 060/100 cost: 0.296624474 train_acc: 0.900 test_acc: 0.920 Epoch: 065/100 cost: 0.294104092 train_acc: 0.910 test_acc: 0.920 Epoch: 070/100 cost: 0.291851377 train_acc: 0.960 test_acc: 0.921 Epoch: 075/100 cost: 0.289864607 train_acc: 0.930 test_acc: 0.920 Epoch: 080/100 cost: 0.287947868 train_acc: 0.930 test_acc: 0.921 Epoch: 085/100 cost: 0.286258464 train_acc: 0.940 test_acc: 0.922 Epoch: 090/100 cost: 0.284703854 train_acc: 0.900 test_acc: 0.922 Epoch: 095/100 cost: 0.283222901 train_acc: 0.910 test_acc: 0.921 DONE
当你运行到这里的时候,有没有自身感觉到整个模型等于是分模块进行的
1、初始化要用的东西
2、跑一遍初始化
3、定义模型
4、定义损失函数
5、给模型喂数据
6、跑模型、调参