变量:
helper、begin、startNo、step、nums
思路:
将helper放到first后面
helper和first移动到开始位置
结束条件是只剩下一个结点的时候
代码
public class Test {
public static void main(String[] args) {
CirList cirList = new CirList();
cirList.add(5);
cirList.show();
cirList.jp(1, 2, 5);
}
}
class CirList{
CirNode first = null;
public void add(int nums) {
if(nums < 1) {
System.out.print("数字不正确!");
return;
}else {
CirNode cur = null;
for(int i = 1; i <= nums; i++) {
CirNode boy = new CirNode(i);
if(i == 1) {
first = boy;
cur = first;
boy.setNext(first);
}else {
cur.setNext(boy);
cur = boy;
boy.setNext(first);
}
}
}
}
public void show() {
if(first.getNext() == null) {
System.out.print("循环链表为空!");
return;
}
CirNode cur = first;
while(true) {
System.out.println(cur);
cur = cur.getNext();
if(cur == this.first) {
break;
}
}
}
public void jp(int startNo, int step, int nums) {
if(first == null || startNo < 1 || startNo > nums) {
System.out.print("数据有误!");
return;
}
CirNode helper = first;
while(true) {
if(helper.getNext() == first) {
break;
}
helper = helper.getNext();
}
for(int i = 1; i <= startNo - 1; i++) {
first = first.getNext();
helper = helper.getNext();
}
while(true) {
if(first.getNext() == first) {
break;
}
for(int i = 1; i <= step - 1; i++) {
first = first.getNext();
helper = helper.getNext();
}
System.out.println("出圈:" + first.getNo());
first = first.getNext();
helper.setNext(first);
}
System.out.println("出圈:" + first.getNo());
}
}
class CirNode{
private int no;
private CirNode next;
public CirNode(int no){
this.no = no;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public CirNode getNext() {
return next;
}
public void setNext(CirNode next) {
this.next = next;
}
@Override
public String toString() {
return "HeroNode2 [no=" + this.no + "]";
}
}
扫描二维码关注公众号,回复:
15678660 查看本文章
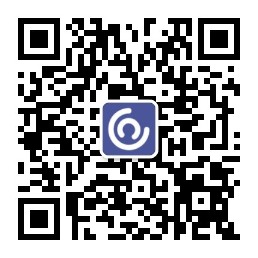