转换为gray灰度图
imgGray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
高斯模糊
imgBlur = cv2.GaussianBlur(imgGray,(5,5),1) #
#边缘检测 1,30
imgCanny = cv2.Canny(imgBlur,1,30)
膨胀处理,迭代2次
imgDial = cv2.dilate(imgCanny,kernel,iterations=2)
腐蚀处理,迭代1次
imgThres = cv2.erode(imgDial,kernel,iterations=1)
寻找轮廓
contours, hier = cv2.findContours(imgThres, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[-2:]
for cidx,cnt in enumerate(contours): #遍历轮廓
area = cv2.contourArea(cnt) #获取轮廓的面积
minAreaRect = cv2.minAreaRect(cnt) #获取最小外接矩形
((cx, cy), (w, h), theta) = minAreaRect #中心点坐标,长宽,旋转角度[-90,0),当矩形水平或竖直时均返回-90
print('面积: '+str(w)+' '+str(h)+' '+str(theta)+' '+str(cx)+' '+str(cy))
旋转图片
def rotate(img,angle,rotPoint=None):
(height,width) = img.shape[:2]
if rotPoint is None:
rotPoint = (width // 2,height // 2)
rotMAT = cv2.getRotationMatrix2D(rotPoint,angle,1.0)
dimension = (width,height)
return cv2.warpAffine(img,rotMAT,dimension)
bytes图片类型转为np.ndarray
img = cv2.imdecode(np.frombuffer(img_bytes, np.uint8), cv2.IMREAD_COLOR)
从文件路径获取到np.ndarray
img = cv2.imread('./photo_new/photo1/3.jpg')
np.ndarray转换为base64
file_img = []
base64_str = base64.b64encode(i)
file_img.append('data:image/jpeg;base64,' + str(base64_str,'utf8'))
把文字倾斜的图片扶正 by opencv
扫描二维码关注公众号,回复:
15302993 查看本文章
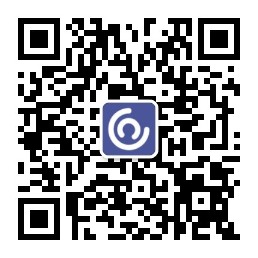
可以使用OpenCV的对极坐标变换(Polar Coordinate Transformation)实现,具体流程如下:
-
将图像转换为灰度图像。
-
使用Canny边缘检测算法检测图像中的边缘。
-
使用Hough变换检测图像中的直线。
-
根据检测到的直线,使用对极坐标变换(Polar Coordinate Transformation)计算文字倾斜的角度,并进行旋转矫正。
-
最后,使用仿射变换(Affine Transformation)将图像进行扶正。
import cv2
import numpy as np
# 读入图像
image = cv2.imread('image.jpg')
# 将图像转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用Canny边缘检测算法检测图像中的边缘
edges = cv2.Canny(gray_image, 100, 200)
# 使用Hough变换检测图像中的直线
lines = cv2.HoughLinesP(edges, 1, np.pi/180, 100)
# 根据检测到的直线,使用对极坐标变换(Polar Coordinate Transformation)计算文字倾斜的角度
angle = 0
for line in lines:
x1, y1, x2, y2 = line[0]
angle += np.arctan((y2 - y1) / (x2 - x1))
angle /= len(lines)
# 旋转矫正
(h, w) = image.shape[:2]
center = (w // 2, h // 2)
M = cv2.getRotationMatrix2D(center, angle, 1.0)
rotated_image = cv2.warpAffine(image, M, (w, h))
# 使用仿射变换(Affine Transformation)将图像进行扶正
corrected_image = cv2.warpAffine(rotated_image, M, (w, h))
# 保存结果
cv2.imwrite('corrected_image.jpg', corrected_image