【LeetCode】628. Maximum Product of Three Numbers 三个数的最大乘积(Easy)(JAVA)
题目地址: https://leetcode.com/problems/maximum-product-of-three-numbers/
题目描述:
Given an integer array nums, find three numbers whose product is maximum and return the maximum product.
Example 1:
Input: nums = [1,2,3]
Output: 6
Example 2:
Input: nums = [1,2,3,4]
Output: 24
Example 3:
Input: nums = [-1,-2,-3]
Output: -6
Constraints:
- 3 <= nums.length <= 10^4
- -1000 <= nums[i] <= 1000
题目大意
给定一个整型数组,在数组中找出由三个数组成的最大乘积,并输出这个乘积。
注意:
给定的整型数组长度范围是[3,104],数组中所有的元素范围是[-1000, 1000]。
输入的数组中任意三个数的乘积不会超出32位有符号整数的范围
解题方法
- 先对数组进行排序,时间复杂度 O(nlogn)
- 因为无法知道有几个数小于 0, 所以需要根据小 0 的个数来区分判断
- note: 其实这里只用到了最大的三个数和最小的两个数,只需要找出这 5 个数即可,可以优化到时间复杂度 O(n)
class Solution {
public int maximumProduct(int[] nums) {
if (nums.length < 3) return 0;
Arrays.sort(nums);
if (nums[nums.length - 1] <= 0) return nums[nums.length - 1] * nums[nums.length - 2] * nums[nums.length - 3];
if (nums[nums.length - 2] <= 0) return nums[0] * nums[1] * nums[nums.length - 1];
if (nums[nums.length - 3] <= 0) return nums[0] * nums[1] * nums[nums.length - 1];
return Math.max(nums[0] * nums[1], nums[nums.length - 2] * nums[nums.length - 3]) * nums[nums.length - 1];
}
}
执行耗时:12 ms,击败了69.60% 的Java用户
内存消耗:40.1 MB,击败了16.51% 的Java用户
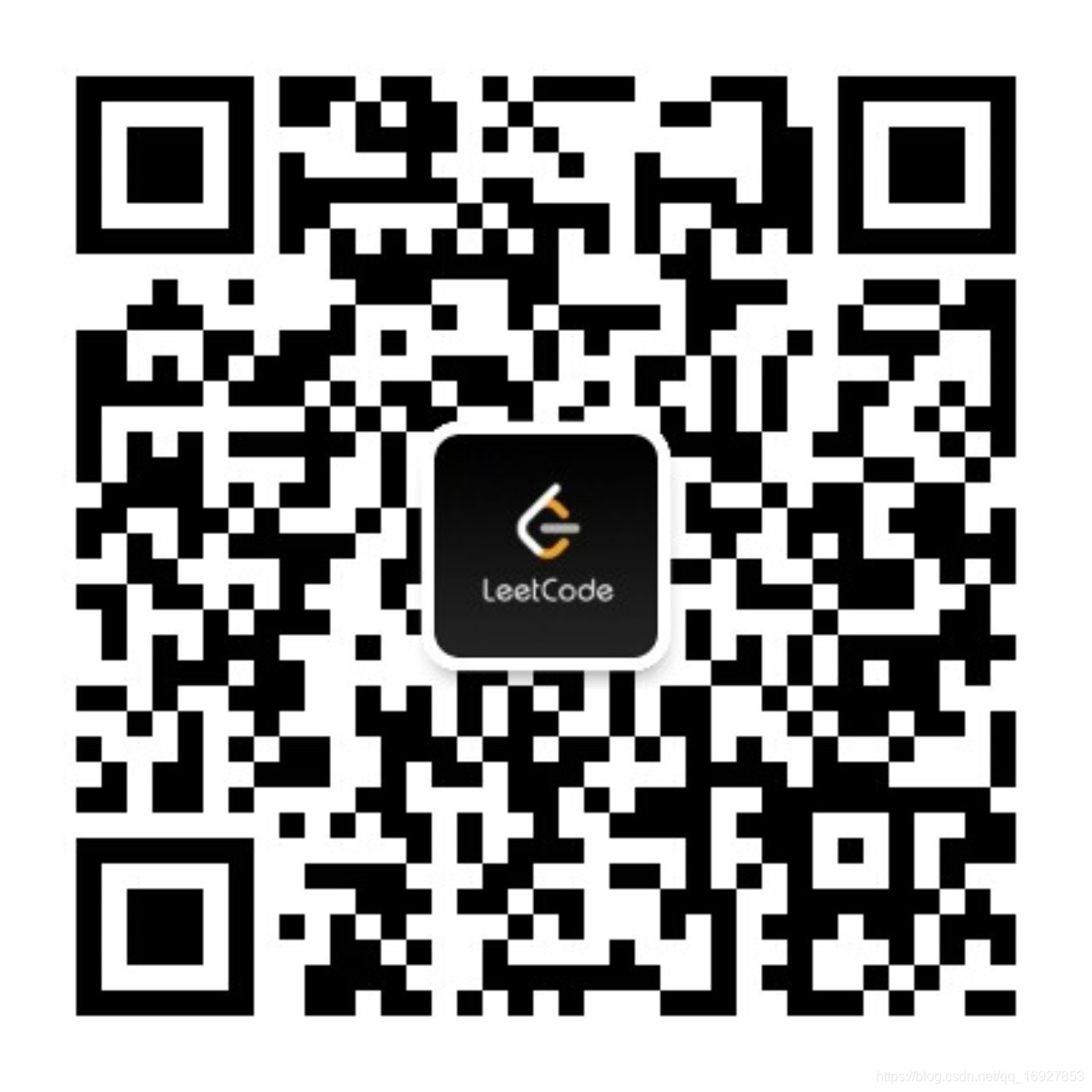