伪代码
FOR each char in string:
IF char is num :
new node(num), then push ptr into stack.
ELSE IF char is Ops:
get top-2 of stack as l、r,
then new node(Ops,l,r),
push ptr into stack.
ELSE:
print error-info, then exit.
RETURN the top of stack, which is root of tree.
过程
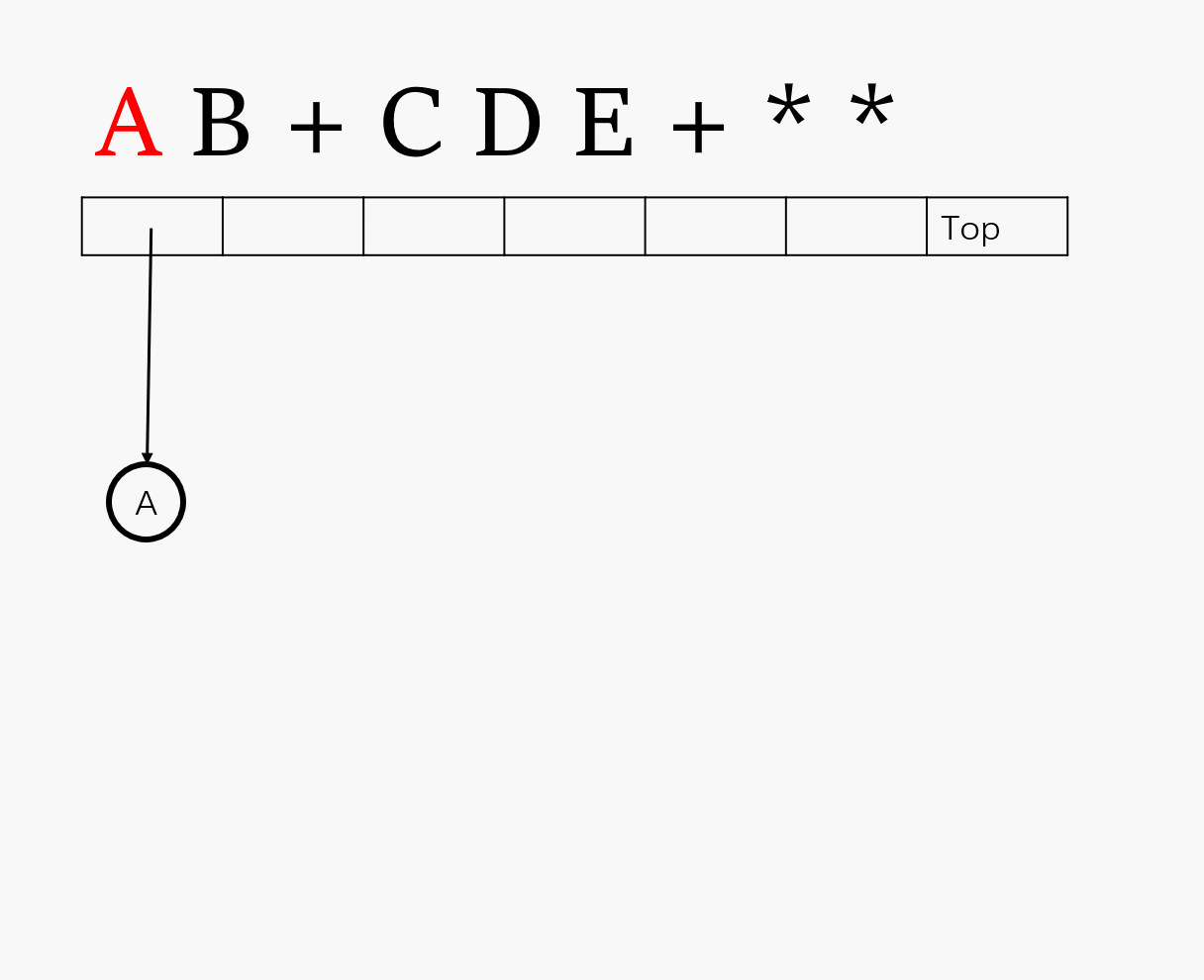
c++实现
#pragma once
#include<iostream>
#include<stack>
#include<cstring>
using namespace std;
typedef char Elem;
//binary tree node abstract class
template<class Elem>
class BinNode {
public:
virtual Elem& val() = 0;
virtual void setVal(const Elem&) = 0;
virtual BinNode* left() const = 0;
virtual void setLeft(BinNode*) = 0;
virtual BinNode* right() const = 0;
virtual void setRight(BinNode*) = 0;
virtual bool isLeaf() = 0;
};
template<class Elem>
class BinNodePtr : public BinNode<Elem> {
private:
Elem value;
BinNodePtr* lc;
BinNodePtr* rc;//right child
public:
BinNodePtr() :value(0), lc(nullptr), rc(nullptr) {}
BinNodePtr(Elem e, BinNodePtr* l = nullptr, BinNodePtr* r = nullptr) :value(e), lc(l), rc(r) {}
~BinNodePtr() {}
//func
Elem& val() { return value; }
void setVal(const Elem& e) { value = e; }
inline BinNode<Elem>* left() const { return lc; }
void setLeft(BinNode<Elem>* p) { lc = (BinNodePtr*)p; }
inline BinNode<Elem>* right() const { return rc; }
void setRight(BinNode<Elem>* p) { rc = (BinNodePtr*)p; }
bool isLeaf() {
return (rc == nullptr) && (lc == nullptr);
}
};
template<class Elem>
BinNodePtr<Elem>* createNode(const Elem &item, BinNodePtr<Elem>* l=nullptr, BinNodePtr<Elem>* r=nullptr) {
return new BinNodePtr<Elem>(item,l,r);
}
bool isOps(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
BinNodePtr<Elem>* fromPostfixExprCreateBiTree(const char* str) {
stack<BinNodePtr<Elem>*> stk;
int len = strlen(str);
for (int i = 0; i < len; i++)
{
char ch = str[i];
if (ch >= 'a' && ch <= 'z') {
stk.push(createNode(ch));
}
else if (isOps(ch)) {
if (stk.empty()) {
printf_s("str not right!!\n");
exit(-1);
}
BinNodePtr<Elem>* r = stk.top(); stk.pop();
BinNodePtr<Elem>* l = stk.top(); stk.pop();
stk.push(createNode(ch, l, r));
}
else {
printf_s("str not right!!\n");
exit(-1);
}
}
if (stk.size() != 1) {
printf_s("str not right!!\n");
exit(-1);
}
return stk.top();
}
void preVisit(BinNode<Elem>* root) {
if (root) {
printf_s("%c ", root->val());
preVisit(root->left());
preVisit(root->right());
}
}
void testFunc() {
char str[] = "ab+cde+**";
preVisit(fromPostfixExprCreateBiTree(str));
}