1. 创建 web-app 项目
导入初始4个 maven 依赖
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp.jstl</groupId>
<artifactId>jstl-api</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
TestServlet.java
package com.beyond.nothing.servlet;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@WebServlet("/test")
public class TestServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
List<String> list = new ArrayList<>();
list.add("张三");
list.add("李四");
list.add("王五");
list.add("博瑞");
req.setAttribute("list", list);
req.getRequestDispatcher("WEB-INF/jsp/test.jsp").forward(req, resp);
}
}
test.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %>
<%--引入jstl标签库--%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Title</title>
</head>
<body>
测试:
<c:forEach items="${list}" var="str">
${str}
</c:forEach>
</body>
</html>
测试:
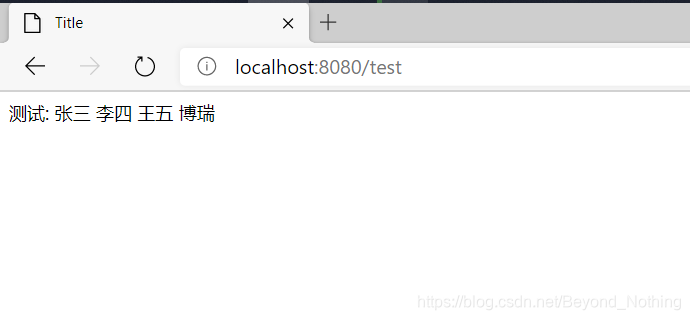
2. 连接数据库
导入依赖 驱动
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.49</version>
</dependency>
编写连接类 JDBCUtil
package com.beyond.nothing.utils;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class JDBCUtil {
private static final String USERNAME = "root";
private static final String PWD = "210374520";
private static final String URL = "jdbc:mysql://localhost:3308/thedemo";
private static final String DRIVER = "com.mysql.jdbc.Driver";
static {
try {
Class.forName(DRIVER);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getCoon() throws SQLException {
return DriverManager.getConnection(URL,USERNAME,PWD);
}
public static void main(String[] args) throws SQLException {
Connection coon = getCoon();
System.out.println(coon);
}
}
测试连接
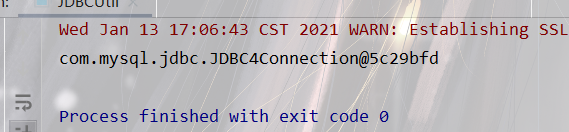
3. 开发一个简单的javaweb项目
Entity
package com.beyond.nothing.entity;
public class Product {
private Integer id;
private String name;
@Override
public String toString() {
return "Product{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Service 层
package com.beyond.nothing.service;
import com.beyond.nothing.dao.TestDao;
import com.beyond.nothing.entity.Product;
import java.util.List;
public class TestService {
private TestDao dao = new TestDao();
public List<Product> queryList(){
return dao.queryList();
}
}
Dao
package com.beyond.nothing.dao;
import com.beyond.nothing.entity.Product;
import com.beyond.nothing.utils.JDBCUtil;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class TestDao {
public List<Product> queryList() {
Connection connection = null ;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
List<Product> list = new ArrayList<>();
try {
connection = JDBCUtil.getCoon();
preparedStatement = connection.prepareStatement("select * from product");
resultSet = preparedStatement.executeQuery();
while (resultSet.next()){
Product product = new Product();
product.setId(resultSet.getInt("id"));
product.setName(resultSet.getString("name"));
list.add(product);
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.close(resultSet,preparedStatement,connection);
}
return list;
}
}
Controller
package com.beyond.nothing.servlet;
import com.beyond.nothing.service.TestService;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@WebServlet("/test")
public class TestServlet extends HttpServlet {
private TestService testService = new TestService();
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setAttribute("list", testService.queryList());
req.getRequestDispatcher("WEB-INF/jsp/test.jsp").forward(req, resp);
}
}
前端 test.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %>
<%--引入jstl标签库--%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Title</title>
</head>
<body>
测试:
<c:forEach items="${list}" var="p">
${
p.id}
${
p.name}
</c:forEach>
</body>
</html>
效果
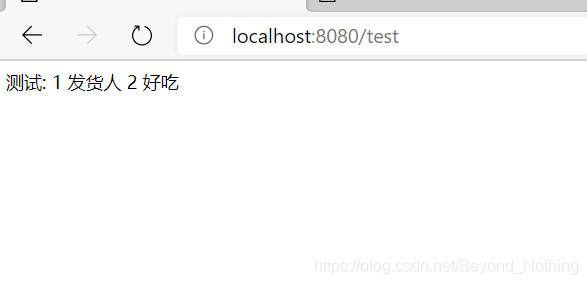