题目:
给定一个链表,返回链表开始入环的第一个节点。 如果链表无环,则返回 null。
为了表示给定链表中的环,我们使用整数 pos 来表示链表尾连接到链表中的位置(索引从 0 开始)。 如果 pos 是 -1,则在该链表中没有环。
说明:不允许修改给定的链表。
示例 1:
输入:head = [3,2,0,-4], pos = 1
输出:tail connects to node index 1
解释:链表中有一个环,其尾部连接到第二个节点。
示例 2:
扫描二维码关注公众号,回复:
11164453 查看本文章
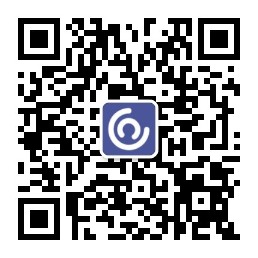
输入:head = [1,2], pos = 0
输出:tail connects to node index 0
解释:链表中有一个环,其尾部连接到第一个节点。
示例 3:
输入:head = [1], pos = -1
输出:no cycle
解释:链表中没有环。
解答:
链接:https://leetcode-cn.com/problems/linked-list-cycle-ii/
1 /** 2 * Definition for singly-linked list. 3 * struct ListNode { 4 * int val; 5 * ListNode *next; 6 * ListNode(int x) : val(x), next(NULL) {} 7 * }; 8 */ 9 class Solution { 10 public: 11 12 ListNode *detectCycle(ListNode *head) 13 { 14 auto fast=head,slow=head; 15 while(fast) 16 { 17 fast=fast->next; 18 slow=slow->next; 19 if(fast) 20 { 21 fast=fast->next; 22 } 23 else 24 { 25 break; 26 } 27 28 if(fast==slow) 29 { 30 fast=head; 31 while (fast!=slow) 32 { 33 fast=fast->next; 34 slow=slow->next; 35 } 36 return fast; 37 } 38 } 39 return NULL; 40 } 41 };