1. make project manager
The project manager is to manage more files
make project manager: also known as "automatic compilation manager".
effect:
1) Read the compilation content of the makefile file to perform specific compilation work
2) Automatically discover updated files based on file timestamps
3) Only compile the changed code files, without compiling them completely
For more information see:
https://www.gnu.org/software/make/
2. Makefile syntax
Makefile is the only configuration file read by make
target: dependency_files
<TAB> command
target: the compiled target, object file or executable
dependency_files dependent files
command Compiled command
for example:
hello.o: hello.c hello.h
gcc -c hello.c -o hello.o
There must be a "TAB key" in front of the command line, otherwise the compilation will report an error.

Just for only one test.c file above, according to the above grammar rules, we need the following Makefile content:
test:test.o
gcc test.o -o test
test.o: test.c
gcc -c -Wall test.c -o test.o
Makefile variables
Makef creates and uses variables in Makefiles. Variables are some names defined in the Makefile that are used instead of a text string called the value of the variable.
user-defined variable
predefined variable
automatic variable
environment variable
1) Custom variable: its value is set by the user
The value of the variable can be used to replace the target body, dependent files, commands and other parts of the Makefile
2) Automatic variables: used to represent the target files and dependent files appearing in the compilation statement, with local meaning.
Common automatic variables:
$< the name of the first dependent file
$@ the full name of the object file
$^ All non-repeating target dependent files, separated by spaces
$? All dependent files with timestamps later than the target file, separated by spaces
3) Predefined variables: pre-defined names of common compilers and assemblers and compilation options
CC The name of the C compiler, default is cc.
RM The name of the file removal program, the default is rm -f
CFLAGS options for the C compiler, no default
The name of the AR library file maintenance program, the default is ar
The name of the CPP C precompiler, default is $(CC) -E
CPPFLAGS C precompiled options, no default
Users can define some commands not related to compilation in a Makefile, such as program packaging, backup and deletion, etc.
Note: .PHONY indicates a pseudo-target
For example: Add the following in the Makefile
.PHONY: clean
clean:
-rm f1.o f2.o main.o test
How to use: make clean
Additional rules:
1) Implicit rule 1: Implicit rules for compiling C programs
Dependent targets for <n>.o targets are automatically deduced as <n>.c
2) Implicit rule 2: Implicit rules for linking object files
Dependency targets for <n> targets are automatically deduced as <n>.o
@echo $(SUBDIRS)
Let echo display the prompt
@(RM)
Predefined variables, rm -f
Make -C $@
Read the Makefile in the specified directory
export CC OBJS BIN OBJS_DIR BIN_DIR
Make submakefiles findable using these variables
Through these Makefile grammar rules, we can become the following Makefile:
gcc -Wall enable all gcc alerts
gcc -c generates .o files
OBJS= test.o
CFLAGS= -c -O -g -Wall
test:$(OBJS)
.PHONY:clean
clean:
-rm $(OBJS)
Execute make clean to delete test.o, the execution process is as follows:
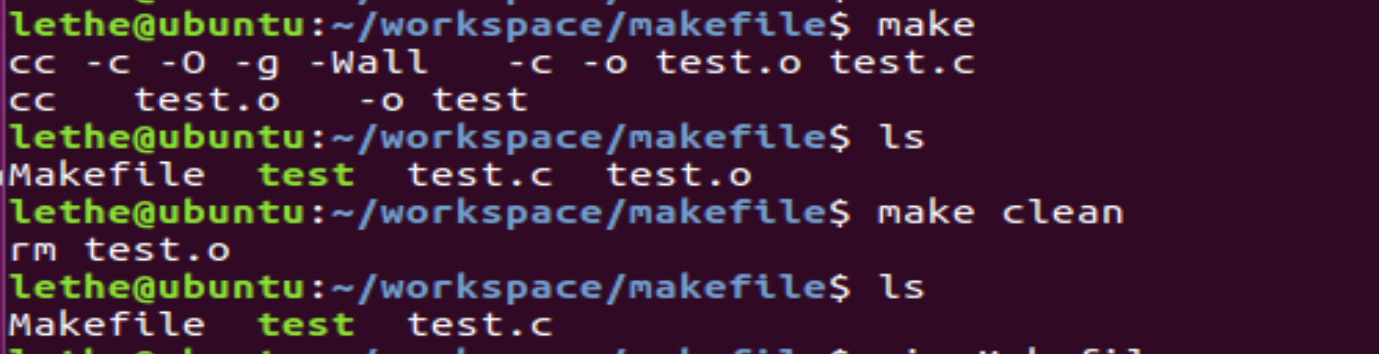
3. Use parameters of the make tool
How to use make:
1) Run make directly
2) make with options
-C //dir读取指定目录下的Makefile
-f //file读取当前目录下的fiLe作为Makefile
-i //忽略所有的命令执行错误
-l //dir指定被包含的Makefile所在目录
-n //只打印要执行的命令,但不执行这些命令
-p //显示make变量数据库和隐含规则
-s //在执行命令时不显示命令
-w //如果make执行时改变目录,打印当前目录名
4. Generate driver Makefile
Write a kernel hello.c
#include <linux/module.h> //所有模块都需要的头文件
#include <linux/init.h> // init&exit相关宏
static int __init hello_init(void){
printk(KERN_ERR "hello world");
return 0;
}
static void __exit hello_exit(void){
printk(KERN_EMERG "hello exit!");
}
module_init(hello_init);
module_exit(hello_exit);
ifneq ($(KERNELRELEASE),)
obj-m :=hello.o // 来指定模块名,注意模块名加.o而不是加.ko
else
KERNELDIR ?=/lib/modules/$(shell uname -r)/build // 目标板linux内核源码顶层目录的绝对路径
all:
make -C $(KERNELDIR) M=$(PWD) modules
clean:
make -C $(KERNELDIR) M=$(PWD) clean
endif
obj-y := hello.o means to compile hello.o into the kernel, do not write this in the project according to the specification.
Make -C indicates that the directory stored in the kernel executes its Makefile
The role of "M=" is: when the module needs to compile an external module based on the kernel, you need to add "M=dir" to the make modules command, and the program will go to the dir directory to find your source file for compilation, make clean is also similar, go to your current directory to clean up (delete all compiled files).
After make, the hello.ko file can be generated, and the file can be loaded into the kernel through insmod (insert module) operation, and the relevant information when loading can be viewed through dmesg.