I. Introduction
For ALIENTEK MiniSTM32, to complete the operation of the marquee, take the LED on the board as an example, to understand which IO port corresponds to the operation of the LED (hardware), and how to operate the IO port (software).
2. Hardware
For ALIENTEK MiniSTM32, the board's own LEDs (DS0, DS1) have been connected to the corresponding IO ports on the development board, DS0 is connected to PA8, and DS1 is connected to PD2.
(The above picture is the connection diagram of STM32 and LED)
3. Software
1. Initialize the IO port, initialize PA8 and PD2 as output ports (and the default output is 1), and enable the clocks of these two ports
(New file led.c)
#include"led.h"
void LED_Init(void)
{
RCC->APB2ENR|=1<<2; //使能PORTA时钟
//(问题:APB2ENR是什么,为什么要选用APB2)
RCC->APB2ENR|=1<<5; //使能PORTD时钟
GPIOA->CRH&=0XFFFFFFF0; //清除相应的位
GPIOA->CRH|=0X00000003; //PA8推挽输出
//(问题:什么是推挽输出,ODR是什么,位运算)
GPIOA->ODR|=1<<8; //PA8输出高
GPIOD->CRL&=0XFFFFF0FF;
GPIOD->CRL|=0X00000300; //PD2推挽输出
//(问题:CRL,CRH是什么)
GPIOD->ODR|=1<<2; //PD2输出高
}
What is APB2ENR?
APB2ENR is the peripheral clock enable register on the APB2 bus
Why choose APB2?
The clock is controlled by RCC, and RCC is mounted on the AHB system bus. If you need to turn on the clock of GPIOB, you need to turn on the peripheral clock of APB2.
What is a push-pull output?
It can output high and low levels and connect to digital devices. The push-pull circuit in stm32 consists of two MOS transistors: the P-MOS transistor is turned on when the output is high, and the pin is connected to VDD (3.3v). When the output is low, the N-MOS is turned on, and the pin is connected to GND.
What are ODRs?
ODR is a port output data register. This register is readable and writable, and the data read from this register can be used to judge the output status of the current IO port.
bit operation
& and 1 are unchanged, and 0 is 0
| or 0 is unchanged, or 1 is 1
What are CRL and CRH? 2
CRL and CRH are two 32-bit port configuration registers in configuration mode, which control the mode and output rate of each IO port.
For this part of the operation, you must first understand which IO port to operate, then select the initialization clock, configure the working mode of the IO port, and finally control the IO port to output high and low levels.
2 New led.h
#ifndef__LED_H
#define__LED_H
#include"sys.h" //sys.h里面定义了STM32的I/O口输入读取宏定义和输出宏定义
//LED端口定义
#define LED0 PAout(8) //PA8
#define LED1 PDout(2) //PD2
void LED_Init(void); //初始化
#endif
(Related macro definitions in sys.h) 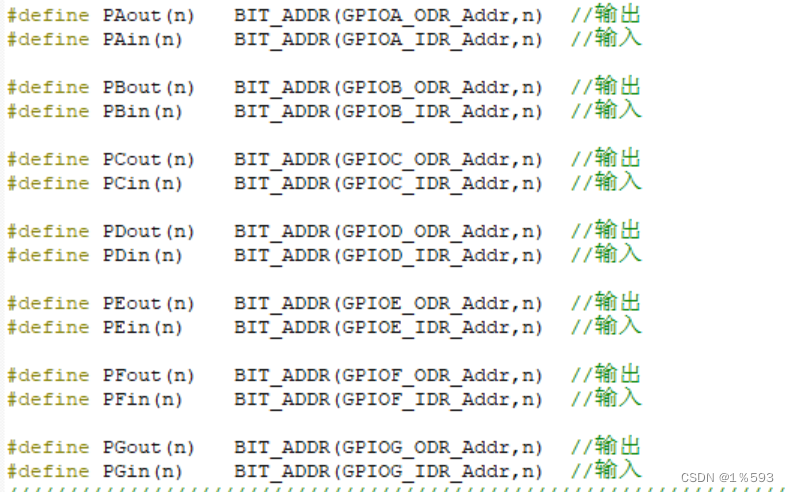
3. Create a new main function
#include "sys.h"
#include "delay.h"
#include "led.h"
int main(void)
{
Stm32_Clock_Init(9); //系统时钟设置
delay_init(72); //延时初始化
LED_Init(); //初始化与LED连接的硬件接口
while(1)
{
LED0=0;
LED1=1;
delay_ms(300);
LED0=1;
LED1=0;
delay_ms(300);
}
}
Clock initialization
In the Stm32_Clock_Init function, we set APB1 to divide by 2, APB2 to divide by 1, and AHB to divide by 1, and select PLLCLK as the system clock. This function has only one parameter PLL, which is used to configure the multiplier of the clock. For example, if the currently used crystal oscillator is 8Mhz, and the value of PLL is set to 9, then the STM32 will run at a speed of 72M.
(u8 represents an 8-bit data type, and a byte is 8 bits, so u8 is a byte.)