C+±029-结构体-2020-3-10
一、定义结构体
C++语言里提供了一种类型叫结构体,通过结构体,可以根据需要建立各种类型的数据类型。例如上面提到的学徒情况。可以这样建立:
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char address[50];
};//注意此处不可忽略fenhao
struct是声明结构体类型时所必须使用的关键字,不能省略,它的作用是声明这是一个"结构体类型"。
student是该结构体的名称。
大括弧内是该结构体中的各个成员,由它们组成一个结构体。例如,上例中的num、name、sex等都是成员。
该结构体只是一个模型,其中并无具体数据,系统对之也不分配实际单元。为了能使用结构体类型的数据,应当定义结构体类型的变量,并在其中存放具体的数据。如:
struct student student1,student2;
其中struct student为结构体类型名,student1,student2为结构体变量名。
定义了student1和student2为struct student 类型的变量,即它们具有struct student类型的结构。系统会为之分配内存空间。
如图
student1 | 10001 | 张三 | M | 16 | 95 | 浙江 |
---|---|---|---|---|---|---|
student2 | 10002 | 李四 | F | 16 | 92 | 北京 |
也可以在声明类型的同时定义变量,例如
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char address[50];
}student1,student2;//注意此处不可省略分号
当然也可以用数组的方式定义变量,例如:
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char address[50];
}stu[10];//注意此处不可省略分号
也可以定义结构体变量时对其初始化,例如
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char address[50];
}a={10001,"张三",'M',16,95,"北京西单999号"};
二、结构体变量的引用
实例一
用数组的方式定义变量
1.
#include<iostream>
using namespace std;
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char addr[50];
}stu[2]={{10001,"张三",'M',16,95,"北京西单999号"},
{10002,"李四",'F',17,91,"浙江66号"}};
int main()
{
cout<<stu[0].num<<stu[0].name<<stu[0].sex<<stu[0].age<<stu[0].score<<stu[0].addr<<endl;
cout<<stu[1].num<<stu[1].name<<stu[1].sex<<stu[1].age<<stu[1].score<<stu[1].addr<<endl;
}
10001张三M1695北京西单999号
10002李四F1791浙江66号
--------------------------------
Process exited with return value 0
Press any key to continue . . .
#include<iostream>
using namespace std;
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char addr[50];
};//不同之处
struct student stu[2]={{10001,"张三",'M',16,95,"北京西单999号"},//不同之处
{10002,"李四",'F',17,91,"浙江66号"}};
int main()
{
cout<<stu[0].num<<stu[0].name<<stu[0].sex<<stu[0].age<<stu[0].score<<stu[0].addr<<endl;
cout<<stu[1].num<<stu[1].name<<stu[1].sex<<stu[1].age<<stu[1].score<<stu[1].addr<<endl;
}
10001张三M1695北京西单999号
10002李四F1791浙江66号
--------------------------------
Process exited with return value 0
Press any key to continue . . .
实例二
定义结构体变量时对其初始化
#include<iostream>
using namespace std;
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char addr[50];
}a={10001,"张三",'M',16,95,"北京西单999号"},//注意此处为逗号
b={10002,"李四",'F',17,91,"浙江66号"};//结束为分号
int main()
{
cout<<a.num<<a.name<<a.sex<<a.age<<a.score<<a.addr<<endl;
cout<<b.num<<b.name<<b.sex<<b.age<<b.score<<b.addr<<endl;
}
10001张三M1695北京西单999号
10002李四F1791浙江66号
--------------------------------
Process exited with return value 0
Press any key to continue . . .
实例三
输入结构体变量中并打印
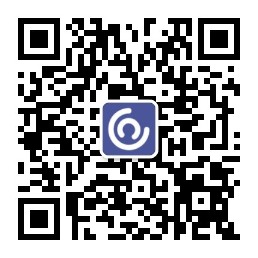
#include<iostream>
using namespace std;
struct student
{
int num;
char name[20];
char sex;
int age;
float score;
char addr[50];
};
struct student a,b;
int main()
{
cin>>a.num>>a.name>>a.sex>>a.age>>a.score>>a.addr;
cin>>b.num>>b.name>>b.sex>>b.age>>b.score>>b.addr;
cout<<a.num<<a.name<<a.sex<<a.age<<a.score<<a.addr<<endl;
cout<<b.num<<b.name<<b.sex<<b.age<<b.score<<b.addr<<endl;
}
1
2
3
4
5
6
7
8
9
10
11
12
123456
789101112
--------------------------------
Process exited with return value 0
Press any key to continue . . .
引用结构体变量应遵循以下规则:
(1)不能将一个结构体变量作为一个整体进行输入和输出,例如已定义student1和student2为结构体变量并且它们已有值。不能这样引用:
cout<<student1: (2)对成员变量可以像普通变量一样进行各种运算(根据其类型决定可以进行的运算)例如
student1.score=student2.score; sum=student1,score+student2.score;
student1.age++;
三、指向结构体类型数据的指针
一个结构体变量的指针就是该变量所占据的段的起始地址。可以设一个指针变量,用来指向一个结构体变量,此时该指针变量的值是结构体变量的起始地址。指针变量也可能用来指向结构体数组中的元素。
实例
#include<iostream>
#include<cstring>
using namespace std;
int main()
{
struct student
{
int num;
char name[20];
char sex;
float score;
};
struct student stu;
struct student *p;
p=& stu;
stu.num=10001;
strcpy(stu.name,"Mike");//注意此处赋值的方式
stu.sex='M';
stu.score=90.5;
cout<<stu.num<<stu.name<<stu.sex<<stu.score<<endl;
cout<<(*p).num<<(*p).name<<(*p).sex<<(*p).score<<endl;
return 0;
}
10001MikeM90.5
10001MikeM90.5
--------------------------------
Process exited with return value 0
Press any key to continue . . .
在C++语言中,为了使用方便和使之直观,可以把(*p).num改用p->num来代替,即p所指的结构体变量中的num成员。同样,(*p).name等价于p->name。
下面是一个指针结构体数组的指针实例:
#include<iostream>
#include<cstring>
using namespace std;
struct student
{
int num;
char name[20];
char sex;
float score;
};
struct student stu[2]={{10001,"张三",'M',95},//不同之处
{10002,"李四",'F',91}};
int main()
{
struct student *p;
for(p=stu;p<stu+2;p++)//p为指向结构体
cout<<p->num<<p->name<<p->sex<<p->score<<endl;
return 0;
}
10001张三M95
10002李四F91
--------------------------------
Process exited with return value 0
Press any key to continue . . .