转发和重定向
success.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
登陆成功<br>
欢迎你:
<%
String name=request.getParameter( "username" );
%>
<%=name%>
</body>
</html>
转发
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>show</title>
</head>
<body>
<%
//设置编码
request.setCharacterEncoding( "UTF-8" );
String name=request.getParameter( "username" );
String pwd=request.getParameter( "password" );
if(name.equals( "zs" )&&pwd.equals( "abc" )){
request.getRequestDispatcher( "success.jsp" ).forward( request,response );
//可以获取到数据,并且地址栏没有改变(任然保留转发时的地址)
}else{
out.print( "登陆失败" );
}
%>
</body>
</html>
重定向
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>show</title>
</head>
<body>
<%
//设置编码
request.setCharacterEncoding( "UTF-8" );
String name=request.getParameter( "username" );
String pwd=request.getParameter( "password" );
if(name.equals( "zs" )&&pwd.equals( "abc" )){
response.sendRedirect( "success.jsp" ); //重定向会导致数据丢失
}else{
out.print( "登陆失败" );
}
%>
</body>
</html>
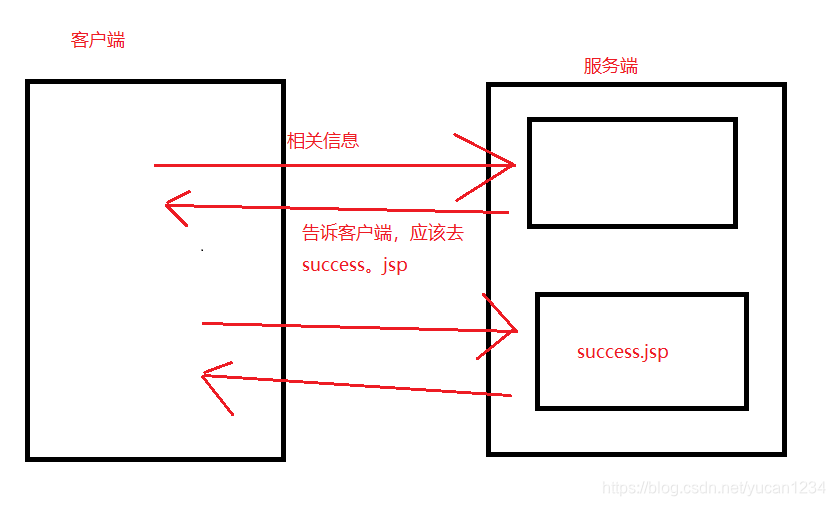
Session
Cookie(客户端,不是内置对象):
Cookie:Key=value
不是内置对象,由java的一个包java.servlet.http.Cookie提供
常用方法
public Cookie(String key,String value) 构造方法
String getName(); 获取name
String getValue(); 获取value
void setMaxAge(int expiry); 设置有效期(秒)
Cookie由服务端产生,再发送给客户端保存。相当于本地缓存的作用
比如访问网站,第一次访问的时候,客户端产生一个Cookie,发送给客户端,然后第二次访问的时,就非常迅速了。
服务端发送给客户端的过程
客户端准备Cookie: respond.addCookie(Cookie cookie);
页面跳转(转发,重定向);
客户端获取cookie: request。getCookie();
注意: a。服务端增加cookie:response对象;客户端获取对象;request对象
b。不能直接过去某一个对象,只能一次性将全部的cookies拿到
优点:提高访问速率,但是安全性较差。
缺点:安全性较差(密码等)
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>show</title>
</head>
<body>
<%
Cookie cookie1=new Cookie( "name","b" );
Cookie cookie2=new Cookie( "password","34" );
response.addCookie( cookie1 );
response.addCookie( cookie2 );
//页面跳转到客户端
response.sendRedirect( "result.jsp" );
%>
</body>
</html>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%
Cookie[] cookies=request.getCookies();
for(Cookie cookie:cookies){
out.print(cookie.getName()+"--"+cookie.getValue()+"<br>");
}
%>
</body>
</html>
显示结果
通过F12查看控制台发现,除了自己设置的Cookies对象外,还有个name为JSESSIONID的Cookie
利用Cookie自动保存用户名
index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java"
import="java.util.Date" %>
<html>
<head>
<title>$Title$</title>
</head>
<body>
<%!
String name;
%>
<%
Cookie[] cookies=request.getCookies();
for(Cookie cookie:cookies){
if(cookie.getName().equals( "name" )){
name=cookie.getValue();
}
}
%>
<form action="show.jsp" method="post">
用户名:<input type="text" name="username" value="<%=(name==null?"":name)%>"/><br/>
//这里有个等式表达式包括三目运算符的地方,需要注意
密码:<input type="password" name="password"/><br/>
<input type="submit" value=登录>
</form>
</body>
</html>
show.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>show</title>
</head>
<body>
<%
//将相关信息存放到cookies中
request.setCharacterEncoding( "utf-8" );
String name=request.getParameter( "username" );
String password=request.getParameter( "password" );
Cookie cookie_name=new Cookie( "name",name );
response.addCookie( cookie_name );
%>
</body>
</html>
第一次进入浏览器的时候,用户名为空,但是第二次进入的时候,会自动显示第一次输入的用户名
session(会话)
浏览网站:开始–关闭 购物:浏览–付款–退出 这一系列都是一次会话
session机制:
客户端第一次请求服务端时,服务端会产生一个session对象(用户保存该客户的信息);
并且每个session对象,都会有一个唯一的sessionId(用于区分其他session)
服务端又会产生一个cookie,并且该cookie的name=JSESSIONID,value=服务器sessionId的值,然后服务器会在响应客户端的同时,将该cookie发送给客户端,至此,客户端就有了一个cookie(JSESSIONID);因此,客户端的cookie就可以 和服务器的session一一对应(JSESSIONID对应sessionId)
之后的请求,服务端会根据客户端cookie中的JSESSIONID去服务端的session中匹配sessionId如果匹配成功(cookie的JSESSIONID和session的sessionId)说明用户不是第一次访问,无需登录。
常用方法:
String geiId(); //获取sessionId
boolean IsNew(); //判断是否是新用户(第一次访问)
void invalidate(); //使session失效;(退出登录,注销)
setAttribute();
getAttribute();
void setMaxInactiveInterval(); //设置最大有效非活动时间(秒)
int getMaxInactiveInterval(); //获取最大有效非活动时间
案例,利用session获取用户名
index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java"
import="java.util.Date" %>
<html>
<head>
<title>$Title$</title>
</head>
<body>
<form action="check.jsp" method="post">
用户名:<input type="text" name="username" /><br/>
密码:<input type="password" name="password"/><br/>
<input type="submit" value=登录>
</form>
</body>
</html>
check.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%
request.setCharacterEncoding( "utf-8" );
String name=request.getParameter( "username" );
String password=request.getParameter( "password" );
if(name.equals( "zs" )&&password.equals( "abc" )){
//只有登录成功session中才会存在username和password
session.setAttribute( "username",name );
session.setAttribute( "password",password );
request.getRequestDispatcher( "sessionDemo.jsp" ).forward( request,response );
}else {
response.sendRedirect( "index.jsp" );
}
%>
</body>
</html>
sessionDeom.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
欢迎你:
<%
String name= (String) session.getAttribute( "username" );
//如果用户没有登陆,直接访问sessionDemo.jsp则必然获得name==null
if(name!=null){
out.print( name );
}else{
response.sendRedirect( "index.jsp" );
}
%>
</body>
</html>