Checkbox
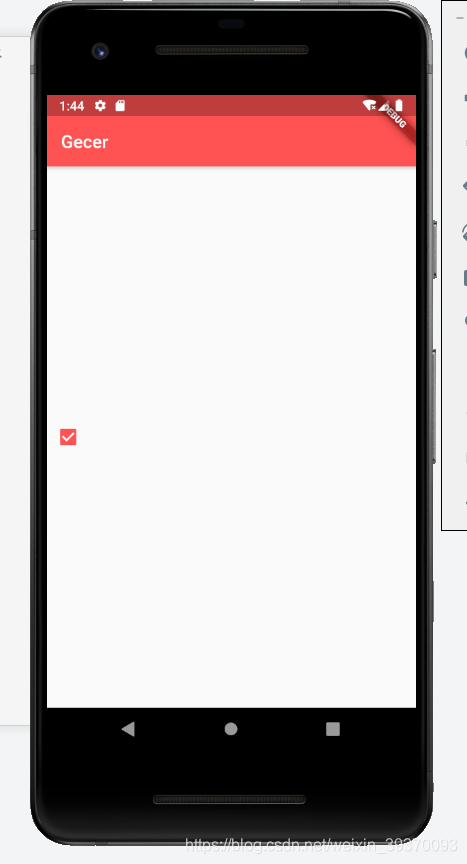
class _MyHomeState extends State<MyHome> with SingleTickerProviderStateMixin {
AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(vsync: this);
}
@override
void dispose() {
super.dispose();
_controller.dispose();
}
bool _checkBoxState=true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Gecer')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Checkbox(
value: _checkBoxState,
onChanged: (value) {
setState(() {
_checkBoxState=value;
});
},
activeColor: Colors.redAccent,//选中颜色
)
]));
}
}
CheckboxListTile
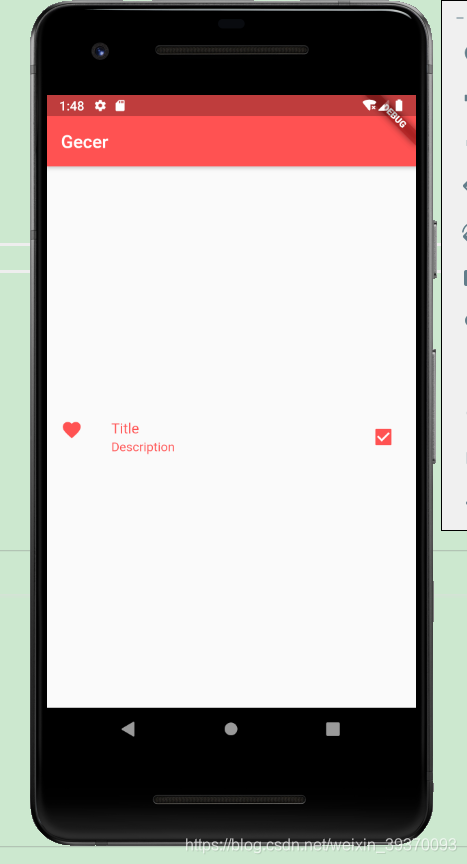
CheckboxListTile(
value: _checkBoxState,
onChanged: (value) {
setState(() {
_checkBoxState = value;
});
},
title: Text('Title'),
subtitle: Text('Description'),
secondary: Icon(Icons.favorite),
selected: _checkBoxState,
activeColor: Colors.redAccent,
)
Radio
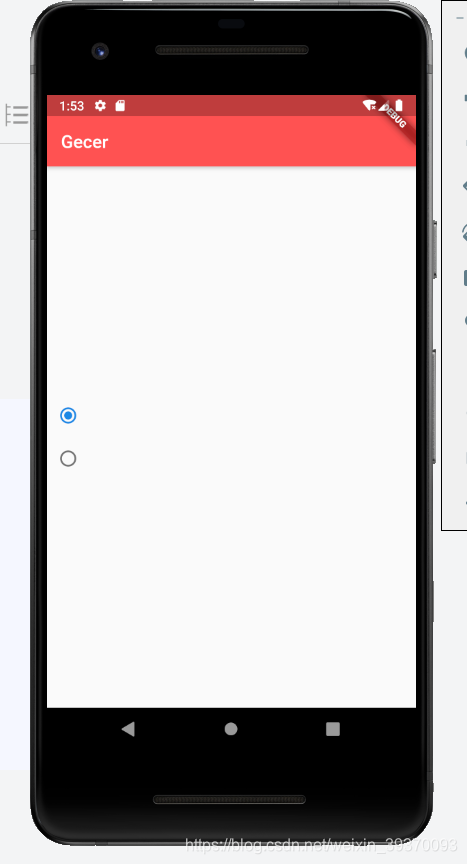
//选中的值
int _selectedValue = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Gecer')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Radio(
value: 0,
groupValue: _selectedValue,
onChanged: (value) {
setState(() {
_selectedValue = value;
});
},
),
Radio(
value: 1,
groupValue: _selectedValue,
onChanged: (value) {
setState(() {
_selectedValue = value;
});
},
)
]));
}
RadioListTile
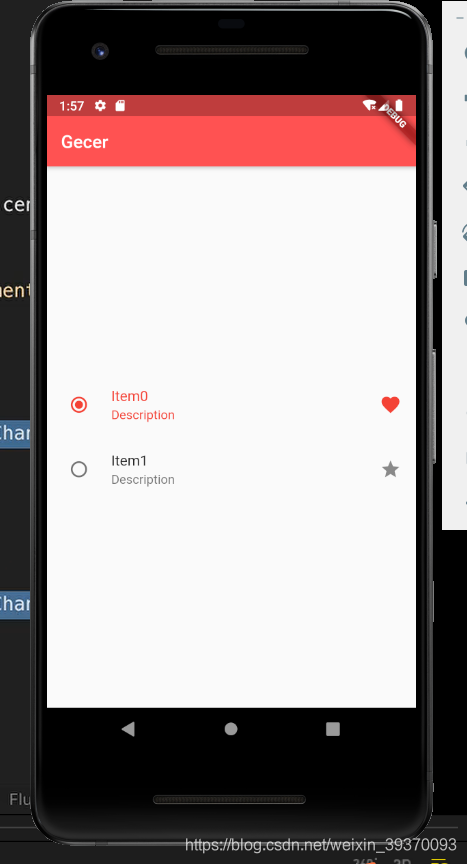
int _selectedValue = 0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Gecer')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
RadioListTile(
value: 0,
groupValue: _selectedValue,
onChanged: (value) {
setState(() {
_selectedValue = value;
});
},
title: Text('Item0'),
subtitle: Text('Description'),
secondary: Icon(Icons.favorite),
selected: _selectedValue == 0,//是否显示显示样式
activeColor: Colors.red,
),
RadioListTile(
value: 1,
groupValue: _selectedValue,
onChanged: (value) {
setState(() {
_selectedValue = value;
});
},
title: Text('Item1'),
subtitle: Text('Description'),
secondary: Icon(Icons.star),
selected: _selectedValue == 1,
activeColor: Colors.red,
)
]));
}
Switch
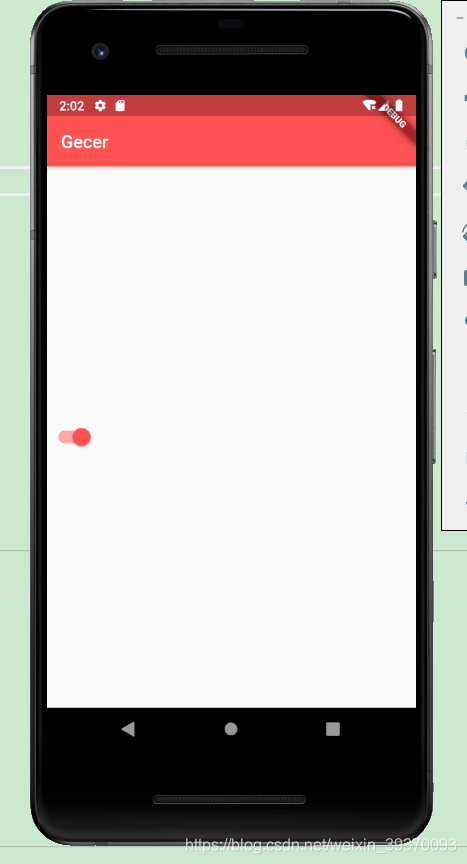
Switch(
activeColor: Theme.of(context).primaryColor,
value: _isSwitched,
onChanged: (value) {
setState(() {
_isSwitched = value;
});
},
),
SwitchListTile
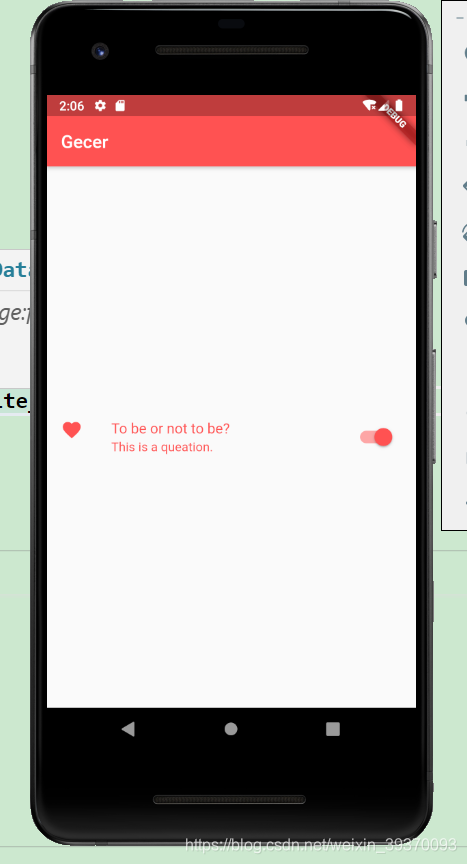
SwitchListTile(
activeColor: Theme.of(context).primaryColor,
value: _isSwitched,
onChanged: (value) {
setState(() {
_isSwitched = value;
});
},
title: Text('To be or not to be?'),
subtitle: Text('This is a queation.'),
secondary:
Icon(_isSwitched ? Icons.favorite : Icons.favorite_border),//切换图标
selected: _isSwitched,//样式是否激活
),
Slider
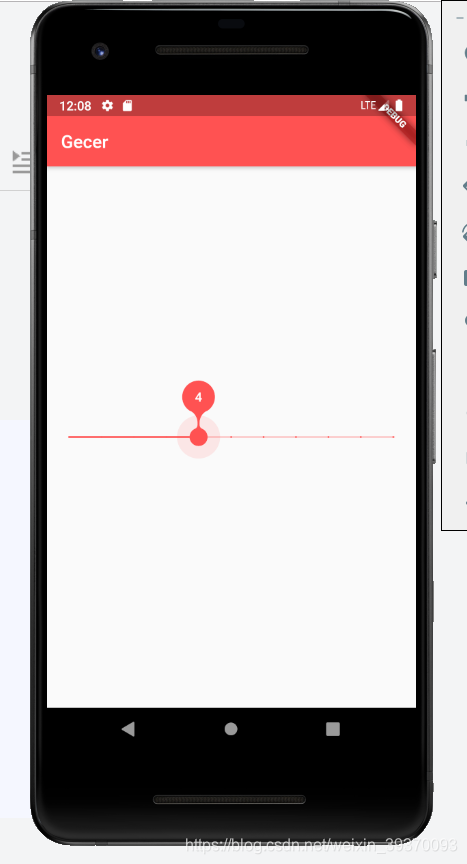
Slider(
value: _sliderValue,
onChanged: (value) {
setState(() {
_sliderValue = value;
});
},
activeColor: Theme.of(context).primaryColor,//激活的颜色
inactiveColor: Theme.of(context).primaryColor.withOpacity(0.3),//未激活的颜色
min: 0.0,
max: 10.0,
divisions: 10,//分割成份数
label: '${_sliderValue.toInt()}',//拖动时显示的lable
),
showTimePicker
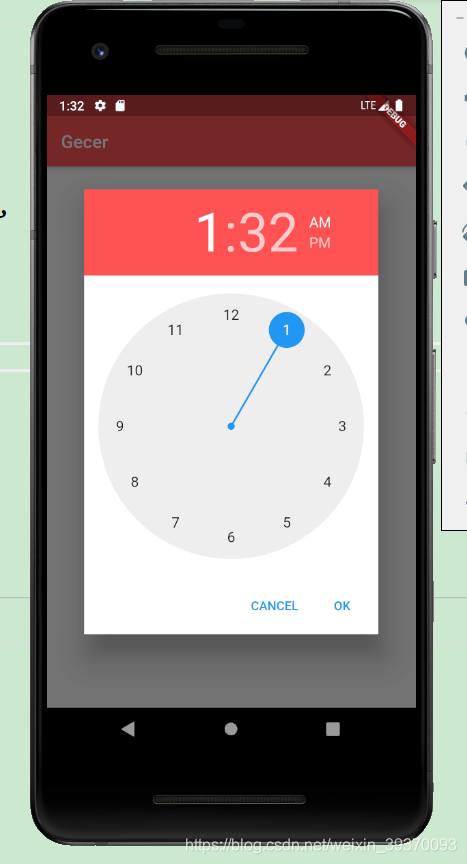
TimeOfDay _selectedTime = TimeOfDay.now();
//异步显示选择时间
Future<void> _selectTime() async {
final TimeOfDay time = await showTimePicker(
context: context,
initialTime: _selectedTime,
);
if (time == null) return;
setState(() {
_selectedTime = time;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Gecer')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
//使用InkWell 是为了给子元素添加点击事件
InkWell(
onTap: _selectTime,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Icon(
Icons.favorite,
color: Colors.redAccent,
),
//DateFormat 引用于intl
Text(_selectedTime.format(context))
]),
)
]));
}
showDatePicker
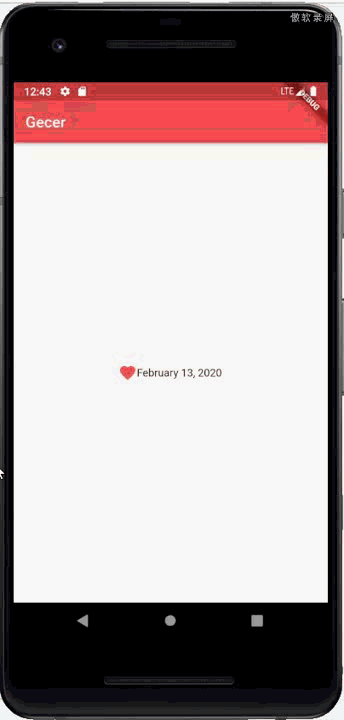
DateTime _selectedDate = DateTime.now();
//异步显示选择日期
Future<void> _selectDate() async {
final DateTime date = await showDatePicker(
context: context,
initialDate: _selectedDate,//初始选中的日期
firstDate: DateTime(1900),//开始日期
lastDate: DateTime(2100),//结束日期作为选择范围
);
if (date == null) return;
setState(() {
_selectedDate = date;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Gecer')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
//使用InkWell 是为了给子元素添加点击事件
InkWell(
onTap: _selectDate,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Icon(Icons.favorite,color: Colors.redAccent,),
//DateFormat 引用于intl
Text(DateFormat.yMMMMd().format(_selectedDate))
]),
)
]));
}