1、简介
这篇内容,主要简单实现了根据Spring的RedisTemplate类实现对Redis进行增删改查的简单Demo。
2、构建Spring Boot项目
构建Spring Boot项目的方式有很多种:
- 在 https://start.spring.io 网站构建Spring Boot项目
- 通过idea+maven构建
- 通过eclipse+maven构建
这里不再验证具体的步骤。首先贴出pom文件需要的配置,如下所示:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.hsh</groupId>
<artifactId>jenkins</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<finalName>Jenkins</finalName>
</build>
</project>
注:这个项目是原来测试jenkins用的项目,所以会有一些jenkins的相关信息,可以忽略。
其中,主要是spring-boot-starter-data-redis、spring-boot-starter-test两个依赖,因为最后是通过单元测试进行了测试,所以spring-boot-starter-web依赖在这个过程中没有使用。
然后修改Spring Boot应用的启动类(后续单元测试的时候,需要用到),代码如下:
package com.hsh.jenkins;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
@SpringBootApplication
public class Application extends SpringBootServletInitializer{
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(Application.class);
}
}
3、开始集成Redis
其实,在 上面的pom文件中,我们已经引入了Redis的依赖文件,下面我需要继续进行相关配置。
3.1、配置文件-增加Redis相关配置
在application.properties文件中,配置Redis需要的参数,如下所示:
#应用配置
server.port=8080
server.servlet.context-path=/jenkins
#数据库的索引编号
spring.redis.database=0
#主机ip
spring.redis.host=192.168.0.182
#端口号
spring.redis.port=6379
#密码,默认空
spring.redis.password=123456
# 连接超时时间 单位 ms(毫秒)
spring.redis.timeout=3000
# 连接池中的最大空闲连接,默认值也是8。
spring.redis.pool.max-idle=8
#连接池中的最小空闲连接,默认值也是0。
spring.redis.pool.min-idle=0
# 如果赋值为-1,则表示不限制;如果pool已经分配了maxActive个jedis实例,则此时pool的状态为exhausted(耗尽)。
spring.redis.pool.max-active=8
# 等待可用连接的最大时间,单位毫秒,默认值为-1,表示永不超时。如果超过等待时间,则直接抛出JedisConnectionException
spring.redis.pool.max-wait=-1
其中,各个参数的含义,已经在注释上解释,不再赘述了。
3.2、添加Redis配置类
添加Redis配置类,其实就是通过java的方式,注册了一个StringRedisTemplate类的实例到上下文中。这个StringRedisTemplate类是RedisTemplate类的一个子类,主要处理字符串相关操作,本实例就是通过StringRedisTemplate实例完成了和Redis交互的demo。
package com.hsh.jenkins;
import java.net.UnknownHostException;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.StringRedisTemplate;
@Configuration
public class RedisConfiguration {
@Bean
@ConditionalOnMissingBean(StringRedisTemplate.class)
public StringRedisTemplate stringRedisTemplate(
RedisConnectionFactory redisConnectionFactory)
throws UnknownHostException {
StringRedisTemplate template = new StringRedisTemplate();
template.setConnectionFactory(redisConnectionFactory);
return template;
}
}
3.3、封装Service层
封装一个RedisService类,这个类实现了Redis的增删改查功能。在实际的开发中,这个类就可以作为操作Redis的封装类来使用了。本实例就直接通过单元测试验证该类中定义的方法了。代码如下:
package com.hsh.jenkins.redis;
import java.util.concurrent.TimeUnit;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class RedisService {
@Autowired
private StringRedisTemplate stringRedisTemplate;
//增、改
public void add(String key, String str, Long time) {
stringRedisTemplate.opsForValue().set(key, str, time, TimeUnit.MINUTES);
}
//删
public void delete(String key) {
stringRedisTemplate.opsForValue().getOperations().delete(key);
}
//查
public String get(String key) {
String source = stringRedisTemplate.opsForValue().get(key);
return source;
}
}
3.4、验证RedisService中的方法
这一步主要通过单元测试的方法,验证RedisService 定义的方法。具体代码如下:
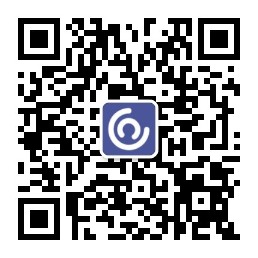
package com.hsh.test.redis;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import com.hsh.jenkins.Application;
import com.hsh.jenkins.redis.RedisService;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = Application.class)
public class RedisTest {
@Autowired
private RedisService redisService;
@Before
public void setUp() {
}
@Test
public void add() {
redisService.add("testKey", "testValue", 10L);
}
@Test
public void get() {
String val = redisService.get("testKey");
System.out.println("val:" + val);
}
@Test
public void del() {
redisService.delete("testKey");
}
}
其中,这个类中需要注意的就是@SpringBootTest注解中的classes的值,因为这个单元测试类RedisTest和SpringBoot应用的启动类不再同一个包下,所以需要把SpringBoot启动类Application类引入进来。
4、异常处理
- DENIED Redis is running in protected mode because protected mode is enabled ……
如果在执行过程中,出现如下错误,说明Redis服务的配置需要调整,即Redis开启了保护模式(默认开启),同时Redis又没有设置访问密码造成的。仔细阅读报错,可以发现,Redis提供了4中解决该报错的方式,具体如下:
第一种:通过命令行CONFIG SET protected-mode no关闭保护模式,这种方式是临时的,重启服务后,就会失效。(不建议在连接的互联网的环境中使用)
第二种:通过修改redis.conf配置文件实现,即把protected-mode yes修改成protected-mode no,然后重启服务器即可,这种方式和第一种相比,第一种方法不需要重启服务器,这种方法是永久生效的。
第三种:启动Redis服务的时候,添加–protected-mode no参数。这种方法也是用于测试的情况。
第四中:设置bind的地址和Redis密码。这种方式是在正式、联网环境可以安全使用的方法。(requirepass 的密码建议要复杂一些,否则不安全,我这里是内网环境所以用的比较简单)
5、实例源码
本篇实例的源码下载地址:https://gitee.com/hsh2015/learningDemo/tree/master/jenkins-test