业务逻辑文件编写
use think\Action; //自己封装的curl方法,详情看附录 define("TOKEN", "你设置的token"); class Customer extends Controller { //校验服务器地址URL public function checkServer(){ if (isset($_GET['echostr'])) { $this->valid(); }else{ $this->responseMsg(); } } public function valid() { $echoStr = $_GET["echostr"]; if($this->checkSignature()){ header('content-type:text'); echo $echoStr; exit; }else{ echo $echoStr.'+++'.TOKEN; exit; } } private function checkSignature() { $signature = $_GET["signature"]; $timestamp = $_GET["timestamp"]; $nonce = $_GET["nonce"]; $token = TOKEN; $tmpArr = array($token, $timestamp, $nonce); sort($tmpArr, SORT_STRING); $tmpStr = implode( $tmpArr ); $tmpStr = sha1( $tmpStr ); if( $tmpStr == $signature ){ return true; }else{ return false; } } public function responseMsg() { //此处推荐使用file_get_contents('php://input')获取后台post过来的数据 $postStr = file_get_contents('php://input'); if (!empty($postStr) && is_string($postStr)){ $postArr = json_decode($postStr,true); if(!empty($postArr['MsgType']) && $postArr['MsgType'] == 'text'){ //用户给客服发送文本消息 if($postArr['Content'] == 7){ //接收到指定的文本消息,触发事件 $fromUsername = $postArr['FromUserName']; //发送者openid $media_id = '上传到微信服务器的图片id,看第三部分'; //输入想要回复的图片消息的media_id $this->requestIMAGE($fromUsername,$media_id); } } else if(!empty($postArr['MsgType']) && $postArr['MsgType'] == 'image'){ //用户给客服发送图片消息,按需求设置 } else if($postArr['MsgType'] == 'event' && $postArr['Event']=='user_enter_tempsession'){ //用户进入客服事件 $fromUsername = $postArr['FromUserName']; //发送者openid $content = '你好,欢迎来到***客服,有什么能帮助您的么'; $this->requestTXT($fromUsername,$content); } else{ exit('error'); } }else{ echo "empty"; exit; } } //文本回复 public function requestTXT($fromUsername,$content){ $data=array( "touser"=>$fromUsername, "msgtype"=>"text", "text"=>array("content"=>$content) ); $json = json_encode($data,JSON_UNESCAPED_UNICODE); $this->requestAPI($json); } //图片回复 public function requestIMAGE($fromUsername,$media_id){ $data=array( "touser"=>$fromUsername, "msgtype"=>"image", "image"=>array("media_id"=>$media_id) ); $json = json_encode($data,JSON_UNESCAPED_UNICODE); $this->requestAPI($json); } public function requestAPI($json){ $access_token = $this->get_accessToken(); $action = new Action(); //自己封装的curl方法,详情看附录 $url = "https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token=".$access_token; $output = $action->curl_post($url,$json); if($output == 0){ echo 'success'; exit; } } //调用微信api,获取access_token,有效期7200s public function get_accessToken(){ $url = 'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=你的appid&secret=你的秘钥'; //替换成自己的小程序id和secret $res = file_get_contents($url); $data = json_decode($res,true); $token = $data['access_token']; return $token; } }
上传回复图片到微信服务器
这个是临时的素材接口,只能存在3天,目前小程序不支持永久素材上传,只有公众号支持
public function uploadWxMedia(){ $token = $this->get_accessToken(); $type = "image"; $filepath = Env::get('root_path').'public\\assets\\imageName.png'; //文件在服务器的绝对路径,按自己存放位置修改 $data = array("media"=>new \CURLFile($filepath)); //php5.6以上必须用这种方法上传文件 $url = "https://api.weixin.qq.com/cgi-bin/media/upload?access_token=".$token."&type=".$type; $action = new Action(); //封装的curl方法,看附录 $result = $action->curl_post($url,$data); print_r($result); } //调用微信api,获取access_token,有效期7200s public function get_accessToken(){ $url = 'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=你的appid&secret=你的秘钥'; //替换成自己的小程序id和secret $res = file_get_contents($url); $data = json_decode($res,true); $token = $data['access_token']; return $token; }
访问 uploadWxMedia() 方法就会把设置好的图片上传,会返回一个json数据:
{"type":"image","media_id":"LTbNsi***************JqG","created_at":1558062553}
其中的 media_id 就是用来填写进第二步的回复图片中的值
附录
封装的curl方法
namespace think; class Action { //get方式请求接口 public function get_json($url) { $data = file_get_contents($url); //转换成数组 $data = json_decode($data,true); //输出 return $data; } //post方式请求接口 public function curl_post($url,$data,$headers = null) { //$data 是一个 array() 数组;未编码 $curl = curl_init(); // 启动一个CURL会话 if(substr($url,0,5)=='https'){ // 跳过证书检查 curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); //只有在CURL低于7.28.1时CURLOPT_SSL_VERIFYHOST才支持使用1表示true,高于这个版本就需要使用2表示了(true也不行)。 curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 2); } curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_HEADER, 0); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_POSTFIELDS, $data); if($headers != null){ //post请求中携带header参数 curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); } //返回api的json对象 $response = curl_exec($curl); //关闭URL请求 curl_close($curl); //返回json对象 return $response; } }
扫描二维码关注公众号,回复:
9138357 查看本文章
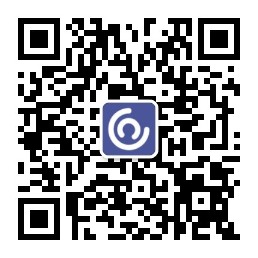