夜光序言:
人生不要被过去所控制,决定你前行的,是未来;
人生不要被安逸所控制,决定你成功的,是奋斗;
人生不要被别人所控制,决定你命运的,是自己;
人生不要被金钱所控制,决定你幸福的,是知足;
人生不要被表象所控制,决定你成熟的,是看透。
扫描二维码关注公众号,回复:
8894087 查看本文章
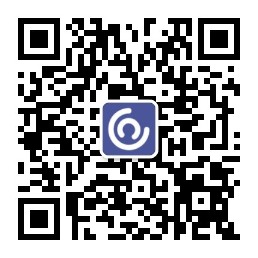
正文:
以道御术 / 以术识道
我们先把项目的框架搭建起来
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<!-- 夜光:下面这个id很重要~~,class对应实体类-->
<bean id="helloBean" class="com.hy.entity.HelloWorld">
<property name="name" value="牧尘" />
</bean>
</beans>
package com.hy.entity;
public class HelloWorld {
//HelloWorld类中有一个name属性
//有name的set方法,还有一个sayHello()方法
private String name;
public void setName(String name) {
this.name = name;
}
public void sayHello() {
System.out.println("setName开始");
System.out.println("hello--- " + name);
System.out.println("setName结束");
}
}
package com.hy.test;
import com.hy.entity.HelloWorld;
public class HellloTest {
public static void main(String[] args) {
System.out.println("测试开始");
HelloWorld hello = new HelloWorld();
hello.setName("萧炎");
hello.sayHello();
System.out.println("测试结束");
}
}
package com.hy.test;
import com.hy.entity.HelloWorld;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class HelloSpringTest {
public static void main(String[] args) {
System.out.println("夜光:spring开始");
//夜光:调用我们的xml文件
ApplicationContext context = new ClassPathXmlApplicationContext("com/hy/applicationContext.xml");
//夜光:(HelloWorld) context.getBean("helloBean") → 获取bean
HelloWorld hello = (HelloWorld) context.getBean("helloBean");
//夜光:调用方法
hello.sayHello();
System.out.println("夜光:spring结束");
}
}
package com.hy;
public class IntroduceMeDemo {
//夜光:定义几个私有变量
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
//定义一个方法
public void Introduce(){
System.out.println("大家好~~,我叫:"+this.name + "今年"+this.age+"岁");
}
}
package com.hy;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class RunTest {
public static void main(String[] args) {
//夜光:这里创建Spring上下文(加载Bean.xml),第一步
ApplicationContext acx= new ClassPathXmlApplicationContext("Bean.xml");
//夜光:这里获取HelloWorld实例,getbean
IntroduceMeDemo id=acx.getBean("IntroduceMeDemo",IntroduceMeDemo.class);
//夜光:这里,我们调用方法Introduce
id.Introduce();
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="IntroduceMeDemo" class="com.hy.IntroduceMeDemo">
<property name="name" value="萧炎"/>
<property name="age" value="26"/>
</bean>
</beans>
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--这里呢、。。我们可以将这个bean理解为我们的javaBean,其中两个property标签即表示给IntroduceMeDemo类中的name和age属性赋值,很有意思-->
<bean id="IntroduceMeDemo" class="com.hy.IntroduceMeDemo" scope="singleton">
</bean>
</beans>
package com.hy;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class RunTest1 {
public static void main(String[] args) {
//夜光:这里创建Spring上下文(加载Bean.xml),第一步
ApplicationContext acx= new ClassPathXmlApplicationContext("Bean1.xml");
//夜光:这里获取HelloWorld实例,getbean
IntroduceMeDemo id=acx.getBean("IntroduceMeDemo",IntroduceMeDemo.class);
//因为采用的是单例,所以,我们需要手动设置一下
id.setName("萧炎");
id.setAge(26);
//夜光:这里,我们调用方法Introduce
id.Introduce();
}
}
package com.hy;
public class IntroduceMeDemo2 {
//夜光:定义几个私有变量
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
//定义一个方法
public void Introduce(){
System.out.println("大家好~~,我叫:"+this.name + "今年"+this.age+"岁");
}
//夜光:bean初始化时调用的方法
public void init(){
System.out.println("嗯唔~~,Bean初始化.....");
}
//夜光:bean销毁时调用的方法
public void destroy(){
System.out.println("嗯唔~~,Bean销毁.....");
}
}
package com.hy;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class RunTest2 {
public static void main(String[] args) {
//夜光:这里创建Spring上下文(加载Bean.xml),第一步
ApplicationContext yg = new ClassPathXmlApplicationContext("Bean2.xml");
//夜光:这里获取HelloWorld实例,getbean
IntroduceMeDemo2 id = yg.getBean("IntroduceMeDemo2",IntroduceMeDemo2.class);
//因为采用的是单例,所以,我们需要手动设置一下
id.setName("萧炎");
id.setAge(26);
//夜光:这里,我们调用方法Introduce
id.Introduce();
//这里,我们需要销毁实例对象
((ClassPathXmlApplicationContext) yg).close();
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--这里呢、。。我们可以将这个bean理解为我们的javaBean,其中两个property标签即表示给IntroduceMeDemo类中的name和age属性赋值,很有意思-->
<bean id="IntroduceMeDemo2" class="com.hy.IntroduceMeDemo2" scope="singleton" init-method="init" destroy-method="destroy">
</bean>
</beans>