注:博客中内容主要来自《狄泰软件学院》,博客仅当私人笔记使用。
测试环境:Ubuntu 10.10
GCC版本:4.4.5
一、基本概念
1)条件编译的行为类似于C语言中的if...else...
2)条件编译是预编译指示命令,用于控制是否编译某段代码
#define C 1
int main()
{
#if(1 == C)
printf("This is first printf.\n");
#else
printf("This is second printf.\n");
#endif
return 0;
}
实例分析
条件编译初探
22-1.c
#include <stdio.h>
#define C 1
int main()
{
const char* s;
#if( C == 1 )
s = "This is first printf...\n";
#else
s = "This is second printf...\n";
#endif
printf("%s", s);
return 0;
}
操作:
1) gcc 22-1.c -o 22-1.out编译正确,打印结果:
This is first printf...
分析:
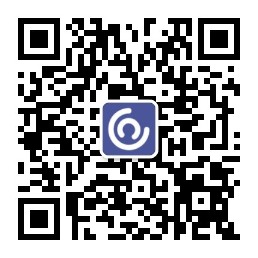
#if……#else……#endif的使用方法类似if…else…
二、条件编译的本质
1)预编译器根据条件编译指令有选择的删除代码
2)编译器不知道代码分支的存在
3)if...else...语句在运行期进行分支判断
4)条件编译指令在预编译期进行分支判断
5)可以通过命令行定义宏
gcc -Dmacro=value file.c
或
gcc -Dmacro file.c
编程实验
通过命令行定义宏
22-2.c
//#include <stdio.h>
int main()
{
const char* s;
#ifdef C //如果有C这个宏,s="This is first printf...\n"
s = "This is first printf...\n";
#else
s = "This is second printf...\n";
#endif
//printf("%s", s);
return 0;
}
操作:
1) 编译时进行宏定义:gcc -DC=1 -o 22-2.out 22-2.c,打印结果:
This is first printf...
2) 编译时不定义宏:gcc -o 22-2.out 22-2.c,打印结果:
This is second printf...
分析:
#ifdef C //如果有C这个宏就打印:
s = "This is first printf...\n";
#else
s = "This is second printf...\n";
#endif
三、#include的本质
1)#include的本质是将已经存在的文件内容嵌入到当前文件中
2)#include的间接包含同样会产生嵌入文件内容的操作
问题:
间接包含同一个头文件是否会产生编译错误?
实例分析
条件编译的使用
global.h
int global = 10;
test.h
#include "global.h"
const char* NAME = "test.h";
char* hello_world()
{
return "Hello world!\n";
}
22-3.c
#include <stdio.h>
#include "test.h"
#include "global.h"
int main()
{
const char* s = hello_world();
int g = global;
printf("%s\n", NAME);
printf("%d\n", g);
return 0;
}
操作:
1) gcc 22-3.c -o 22-3.out编译报错:
In file included from 22-3.c:3:0:
global.h:4:5: error: redefinition of ‘global’
int global = 10;
^
错误:重复定义global
In file included from test.h:4:0,
from 22-3.c:2:
global.h:4:5: note: previous definition of ‘global’ was here
int global = 10;
^
提前定义'global'
解决办法:使用#ifndef和#define
test.h
#ifndef _TEST_H_
#define _TEST_H_
#include "global.h"
const char* NAME = "test.h";
char* hello_world()
{
return "Hello world!\n";
}
#endif
global.h
#ifndef _GLOBAL_H_
#define _GLOBAL_H_
int global = 10;
#endif
22-3.c
#include <stdio.h>
#include "test.h"
#include "global.h"
int main()
{
const char* s = hello_world();
int g = global;
printf("%s\n", NAME);
printf("%d\n", g);
return 0;
}
分析:
第一次预编译头文件时判断是否被编译过,没有被编译过就定义一个宏作为标记;第二次预编译时,发现这个宏后,就不会再次展开头文件防止重复定义内容出错。
3)条件编译可以解决头文件重复包含的编译错误
#ifndef _HEADER_FILE_H_
#define _HEADER_FILE_H_
//source code
#endif
四、条件编译的意义
1)条件编译使得我们可以按不同的条件编译不同的代码段,因而可以产生不同的目标代码
2)#if...#else...#endif被预编译器处理,而if...else...语句被编译器处理,必然被编译进目标代码
3)实际工程中条件编译主要用于以下两种情况:
- 不同的产品线共用一份代码
- 区分编译产品的调试版和发布版
实例分析
产品线区分及调试代码应用
product.h
#define DEBUG 1
#define HIGH 1
22-4.c
#include <stdio.h>
#include "product.h"
#if DEBUG
#define LOG(s) printf("[%s:%d] %s\n", __FILE__, __LINE__, s)
#else
#define LOG(s) NULL
#endif
#if HIGH
void f()
{
printf("This is the high level product!\n");
}
#else
void f()
{
}
#endif
int main()
{
LOG("Enter main() ...");
f();
printf("1. Query Information.\n");
printf("2. Record Information.\n");
printf("3. Delete Information.\n");
#if HIGH
printf("4. High Level Query.\n");
printf("5. Mannul Service.\n");
printf("6. Exit.\n");
#else
printf("4. Exit.\n");
#endif
LOG("Exit main() ...");
return 0;
}
操作:
1) gcc 22-4.c -o 22-4.out编译正确,打印结果:
[22-4.c:24] Enter mian() ...
This is the high level product!
1. Query Information.
2. Record Information.
3. Delete Information.
4. High Level Query.
5. Mannul Service.
6. Exit.
[22-4.c:40] Exit main() ...
分析:
宏定义DEBUG 1,该变了预编译时的结果。
修改宏定义值:
#define DEBUG 0
#define HIGH 0
打印结果:
1. Query Information.
2. Record Information.
3. Delete Information.
4. Exit.
分析:
条件编译(#if……#else……#endif)对宏进行逻辑判断,0为假,非0为真。通过宏定义方式对一份代码产生多种效果(调试版和发布版等)。
小结
1)通过编译器命令行能够定义预处理器使用的宏
2)条件编译可以避免重复包含同一个头文件
3)条件编译是在工程开发中可以区别不同产品线的代码
4)条件编译可以定义产品的发布版和调试版