上篇:第5章 HBase原理
1、判断是否有旧的API
操作步骤
(1)打开IDEA工具,创建HBase工程项目步骤:
新建项目后在pom.xml中添加依赖:
<dependencies>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-server</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>1.3.1</version>
</dependency>
//注意:这个包不用导入,导入的话可能会出错
<dependency>
<groupId>jdk.tools</groupId>
<artifactId>jdk.tools</artifactId>
<version>1.8</version>
<scope>system</scope>
<systemPath>${JAVA_HOME}/lib/tools.jar</systemPath>
</dependencies>
(2)在java文件目录下创建一个com.study包,并在该包下创建一个TestHBase类
具体代码的实现:
TestHBase.java
package com.study;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import java.io.IOException;
public class TestHBase {
//判断表是否存在
public static boolean tableExist(String tableName) throws IOException {
//HBase配置文件
HBaseConfiguration configuration = new HBaseConfiguration();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
//获取HBase管理员对象
HBaseAdmin admin = new HBaseAdmin(configuration);
//执行
boolean tableExists = admin.tableExists(tableName);
//关闭资源
admin.close();
return tableExists;
}
public static void main(String[] args) throws IOException {
System.out.println( tableExist("student"));
System.out.println(tableExist("staff"));
}
}
之后,在Linux环境下启动HRegionServer服务
[root@hadoop106 hbase-1.3.1]# jps
7568 DataNode
7508 QuorumPeerMain
8235 HRegionServer
12861 Jps
接着,在IDEA开发工具,运行此程序,控制台打印出:
2、判断是否有新的API
首先在HBaseAdmin代码点击查看源码:ctrl+鼠标
说明,需要创建一个Connection来代替Admin,就是新 代替旧的
敲出这个代码 ConnectionFactory,点击(ctrl+鼠标)进去
若HBaseConfiguration对象还是过时,我们可以查看源代码点击进去
HBaseConfiguration#create() to construct a plain Configuration:表示不要重新new HBaseConfiguration
具体代码实现:(过时的API变成新的API)
package com.study;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import java.io.IOException;
public class TestHBase {
//判断表是否存在
public static boolean tableExist(String tableName) throws IOException {
//HBase配置文件
// HBaseConfiguration configuration = new HBaseConfiguration();
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = ConnectionFactory.createConnection(configuration);
Admin admin = connection.getAdmin();
//获取HBase管理员对象
// HBaseAdmin admin = new HBaseAdmin(configuration);
//执行
// boolean tableExists = admin.tableExists(tableName);
boolean tableExists = admin.tableExists(TableName.valueOf(tableName));
//关闭资源
admin.close();
return tableExists;
}
public static void main(String[] args) throws IOException {
System.out.println( tableExist("student"));
System.out.println(tableExist("staff"));
}
}
同理:运行此程序,控制台打印出:
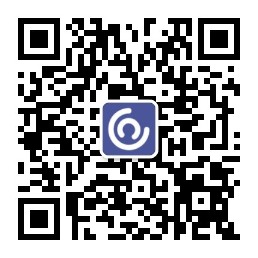
代码也可以另一种写法:
package com.study;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import java.io.IOException;
public class TestHBase {
private static Admin admin=null;
static {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = null;
try {
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
private void close(Connection conn,Admin admin) throws IOException {
if(conn!=null) {
conn.close();
}
if(admin!=null){
admin.close();
}
}
//判断表是否存在
public static boolean tableExist(String tableName) throws IOException {
//HBase配置文件
// HBaseConfiguration configuration = new HBaseConfiguration();
/* Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = ConnectionFactory.createConnection(configuration);
Admin admin = connection.getAdmin();*/
//获取HBase管理员对象
// HBaseAdmin admin = new HBaseAdmin(configuration);
//执行
// boolean tableExists = admin.tableExists(tableName);
boolean tableExists = admin.tableExists(TableName.valueOf(tableName));
//关闭资源
admin.close();
return tableExists;
}
public static void main(String[] args) throws IOException {
System.out.println( tableExist("student"));
System.out.println(tableExist("staff"));
}
}
理:运行此程序,控制台打印出:
3、HBase创建表
具体代码实现:
package com.study;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import java.io.IOException;
public class TestHBase {
private static Admin admin=null;
private static Object String;
static {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = null;
try {
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
private void close(Connection conn,Admin admin) throws IOException {
if(conn!=null) {
conn.close();
}
if(admin!=null){
admin.close();
}
}
//判断表是否存在
public static boolean tableExist(String tableName) throws IOException {
//HBase配置文件
// HBaseConfiguration configuration = new HBaseConfiguration();
/* Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = ConnectionFactory.createConnection(configuration);
Admin admin = connection.getAdmin();*/
//获取HBase管理员对象
// HBaseAdmin admin = new HBaseAdmin(configuration);
//执行
// boolean tableExists = admin.tableExists(tableName);
boolean tableExists = admin.tableExists(TableName.valueOf(tableName));
//关闭资源
admin.close();
return tableExists;
}
//创建表
public static void createTable(String tableName,String... cfs) throws IOException {
if (tableExist(tableName)) {
System.out.println("表已存在!!");
return;
}
//创建表描述器
HTableDescriptor hTableDescriptor = new HTableDescriptor(TableName.valueOf(tableName));
//添加列族
for (String cf:cfs) {
//创建列描述器
HColumnDescriptor hColumnDescriptor = new HColumnDescriptor(cf);
//改变列族版本
// hColumnDescriptor.setMaxVersions(1);默认是1个
hTableDescriptor.addFamily(hColumnDescriptor);
}
//创建表操作
admin.createTable(hTableDescriptor);
System.out.println("表创建成功!!");
}
public static void main(String[] args) throws IOException {
System.out.println( tableExist("student"));
System.out.println(tableExist("staff"));
createTable("staff","info");
System.out.println(tableExist("staff"));
}
}
运行此程序,控制台打印出:
这样我们就可以在Linux环境下,在Hbase的客户端查看表中的数据:
hbase(main):008:0> list
TABLE
staff
1 row(s) in 2.0910 seconds
=> ["staff"]
说明:staff表被创建成功!
4、HBase删除表
具体带代码实现:
package com.study;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import java.io.IOException;
public class TestHBase {
private static Admin admin=null;
private static Connection connection = null;
static {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
try {
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void close(Connection conn,Admin admin) throws IOException {
if(conn!=null) {
conn.close();
}
if(admin!=null){
admin.close();
}
}
//判断表是否存在
public static boolean tableExist(String tableName) throws IOException {
//HBase配置文件
// HBaseConfiguration configuration = new HBaseConfiguration();
/* Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = ConnectionFactory.createConnection(configuration);
Admin admin = connection.getAdmin();*/
//获取HBase管理员对象
// HBaseAdmin admin = new HBaseAdmin(configuration);
//执行
// boolean tableExists = admin.tableExists(tableName);
boolean tableExists = admin.tableExists(TableName.valueOf(tableName));
//关闭资源
admin.close();
return tableExists;
}
//创建表
public static void createTable(String tableName,String... cfs) throws IOException {
if (tableExist(tableName)) {
System.out.println("表已存在!!");
return;
}
//创建表描述器
HTableDescriptor hTableDescriptor = new HTableDescriptor(TableName.valueOf(tableName));
//添加列族
for (String cf:cfs) {
//创建列描述器
HColumnDescriptor hColumnDescriptor = new HColumnDescriptor(cf);
//改变列族版本
// hColumnDescriptor.setMaxVersions(1);默认是1个
hTableDescriptor.addFamily(hColumnDescriptor);
}
//创建表操作
admin.createTable(hTableDescriptor);
System.out.println("表创建成功!!");
}
//删除表
public static void deleteTable(String tableName) throws IOException {
//使用不可用(下线)
admin.disableTable(TableName.valueOf(tableName));
//执行删除操作
admin.deleteTable(TableName.valueOf(tableName));
System.out.println("表已删除!!");
}
public static void main(String[] args) throws IOException {
// System.out.println( tableExist("student"));
System.out.println(tableExist("staff"));
//创建表测试
// createTable("staff","info");
//删除表测试
deleteTable("staff");
System.out.println(tableExist("staff"));
//关闭资源
close(connection,admin);
}
}
运行此程序,控制台打印出:
这样我们就可以在Linux环境下,在Hbase的客户端查看表中的数据:
hbase(main):009:0> list
TABLE
0 row(s) in 0.1240 seconds
=> []
说明:staff表被删除成功!
若对不存在的表进行删除,程序会报错
解决这个问题,我们需要对程序进行作一个判断:
if(!tableExist(tableName)){
return;
}
具体代码(全部)
package com.study;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import java.io.IOException;
public class TestHBase {
private static Admin admin=null;
private static Connection connection = null;
static {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
try {
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void close(Connection conn,Admin admin) throws IOException {
if(conn!=null) {
conn.close();
}
if(admin!=null){
admin.close();
}
}
//判断表是否存在
public static boolean tableExist(String tableName) throws IOException {
//HBase配置文件
// HBaseConfiguration configuration = new HBaseConfiguration();
/* Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "192.168.219.105");
Connection connection = ConnectionFactory.createConnection(configuration);
Admin admin = connection.getAdmin();*/
//获取HBase管理员对象
// HBaseAdmin admin = new HBaseAdmin(configuration);
//执行
// boolean tableExists = admin.tableExists(tableName);
boolean tableExists = admin.tableExists(TableName.valueOf(tableName));
//关闭资源
admin.close();
return tableExists;
}
//创建表
public static void createTable(String tableName,String... cfs) throws IOException {
if (tableExist(tableName)) {
System.out.println("表已存在!!");
return;
}
//创建表描述器
HTableDescriptor hTableDescriptor = new HTableDescriptor(TableName.valueOf(tableName));
//添加列族
for (String cf:cfs) {
//创建列描述器
HColumnDescriptor hColumnDescriptor = new HColumnDescriptor(cf);
//改变列族版本
// hColumnDescriptor.setMaxVersions(1);默认是1个
hTableDescriptor.addFamily(hColumnDescriptor);
}
//创建表操作
admin.createTable(hTableDescriptor);
System.out.println("表创建成功!!");
}
//删除表
public static void deleteTable(String tableName) throws IOException {
if(!tableExist(tableName)){
return;
}
//使用不可用(下线)
admin.disableTable(TableName.valueOf(tableName));
//执行删除操作
admin.deleteTable(TableName.valueOf(tableName));
System.out.println("表已删除!!");
}
public static void main(String[] args) throws IOException {
// System.out.println( tableExist("student"));
System.out.println(tableExist("staff"));
//创建表测试
// createTable("staff","info");
//删除表测试
deleteTable("staff");
System.out.println(tableExist("staff"));
//关闭资源
close(connection,admin);
}
}
重新启动程序运行,控制台打印信息:
做了逻辑判断后,尽管数据没有表,进行删除也不会报错!
注意:
HBase的API操作跟别的API操作有点不同:
(1)别的API操作没有数据,删除不存在的数据是不会报错的!
(2)HBase的API在做项目的过程中,删除不存在的数据会报错,所以,在测试删除不存在的数据,必要做逻辑判断:
if(!tableExist(tableName)){
return;
}