大一下半期数据结构
搜索树判断
对于二叉搜索树,我们规定任一结点的左子树仅包含严格小于该结点的键值,而其右子树包含大于或等于该结点的键值。如果我们交换每个节点的左子树和右子树,得到的树叫做镜像二叉搜索树。
现在我们给出一个整数键值序列,请编写程序判断该序列是否为某棵二叉搜索树或某镜像二叉搜索树的前序遍历序列,如果是,则输出对应二叉树的后序遍历序列。
输入格式:
输入的第一行包含一个正整数N(≤1000),第二行包含N个整数,为给出的整数键值序列,数字间以空格分隔。
输出格式:
输出的第一行首先给出判断结果,如果输入的序列是某棵二叉搜索树或某镜像二叉搜索树的前序遍历序列,则输出YES
,否侧输出NO
。如果判断结果是YES
,下一行输出对应二叉树的后序遍历序列。数字间以空格分隔,但行尾不能有多余的空格。
输入样例1:
7
8 6 5 7 10 8 11
输出样例1:
YES
5 7 6 8 11 10 8
输入样例2:
7
8 6 8 5 10 9 11
输出样例2:
No
题目解答:
扫描二维码关注公众号,回复:
8812465 查看本文章
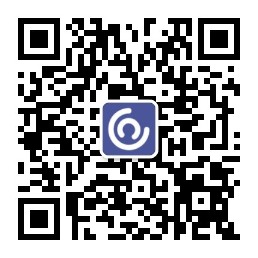
#include <stdio.h>
#include<stdlib.h>
typedef struct node *Node;
struct node {
int Num;
Node Left;
Node Right;
};
int a[10001];
int flag;
int Result;
Node Insert(Node,Node);
Node Read();
void DLR(Node H) {
if(H&&Result) {
// printf("{%d-%d}",a[flag],H->Num);
if(H->Num!=a[flag++])Result=0;
DLR(H->Left);
DLR(H->Right);
}
}
void LRD(Node H) {
if(H) {
LRD(H->Left);
LRD(H->Right);
if(!Result)printf(" ");
else Result=0;
printf("%d",H->Num);
}
}
void Swap(Node H) {
if(H) {
Swap(H->Left);
Swap(H->Right);
Node temp=H->Left;
H->Left=H->Right;
H->Right=temp;
}
}
int main() {
int n;
scanf("%d",&n);
Node Head=NULL;
for(int i=0; i<n; i++) {
Node temp=Read();
a[i]=temp->Num;
Head=Insert(Head,temp);
}
flag=0;
Result=1;
DLR(Head);
if(!Result) {
Swap(Head);
Result=1;
flag=0;
DLR(Head);
}
if(Result) {
printf("YES\n");
// Result=0;
LRD(Head);
} else printf("NO");
return 0;
}
Node Insert(Node H,Node K) {
if(!H) {
return K;
}
if(K->Num<H->Num) {
H->Left=Insert(H->Left,K);
} else H->Right=Insert(H->Right,K);
return H;
}
Node Read() {
Node temp=(Node)malloc(sizeof(struct node));
scanf("%d",&temp->Num);
temp->Left=NULL;
temp->Right=NULL;
return temp;
}