前言
面试的时候Handler几乎是必问的,因为Android中很多事件都是由handler来驱动,比如activity的生命周期,我们通常说的View渲染60fps等,但是对于这么一个重要的东西,很多面试者只能说出Looper,MessageQueue,Handler,Message各自的职责,以及Handler是怎么触发和回调的。但凡问的深入一些,很多人就有点懵逼了。
- handler的dispatchMessage方法具体是怎么实现的
- handler是如何实现线程切换的
- 主线程的Looper在什么时候创建,为什么Looper一直在主线程循环却没有造成ANR
- Handler是如何保证系统消息优先处理的
- .........
实际上还有很多问题值得去探究,如果要一一解答感觉有点太浪费时间,而且别人嚼碎的东西再塞到你嘴里也没啥味道了,所以今天我来带大家分析一下Handler的源码,虽然Handler机制非常重要,但是源码却不是很难,把Handler涉及到的几个重要类都分析一遍,然后再参考着系统实现的方式我们自己手写一个简易版Handler,相信以后在面试过程中遇到Handler必定能战无不胜。
先放一张图让大家温习一下
看完了嘛?我们要开始了。。。。。。。。
1.源码分析
1.1 Message的取出与分发
Hander机制中有几个非常重要的类,Handler.java, MessageQueue.java, Looper.java, Message.java, ThreadLocal.Java。 除开这几个实际上还包含了一个ActivityThread,因为这正是Handler机制开始的地方,首先我们来看下ActivityThread
我们找到ActivityThread类中的main方法,实际上这也是应用程序的入口方法,你看了绝对不会陌生,贴出来的代码中我把一些无关紧要的方法都省略了,仅仅保留和Handler相关的
//哈哈哈,是不是看到特别亲切
public static void main(String[] args) {
..........
//初始化Looper
Looper.prepareMainLooper();
ActivityThread thread = new ActivityThread();
thread.attach(false, startSeq);
if (sMainThreadHandler == null) {
sMainThreadHandler = thread.getHandler();
}
if (false) {
Looper.myLooper().setMessageLogging(new
LogPrinter(Log.DEBUG, "ActivityThread"));
}
// End of event ActivityThreadMain.
Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
//开启Loop循环遍历MessageQueue中的消息
Looper.loop();
throw new RuntimeException("Main thread loop unexpectedly exited");
}
ActivityThread中关于Handler的东西就做了两件事
- 为主线程创建一个全局唯一Looper
- 开启loop循环遍历MessageQueue
接下来肯定要看Looper.prepareMainLooper()中具体干了什么事了
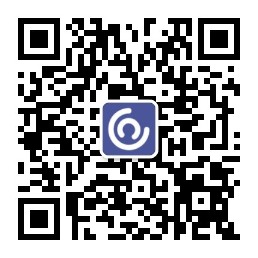
public static void prepareMainLooper() {
//创建Looper
prepare(false);
//检查全局遍历sMainLooper是否不为空
//如果返回true就抛出异常,因为一个线程只能有一个Looper
synchronized (Looper.class) {
if (sMainLooper != null) {
throw new IllegalStateException("The main Looper has already been prepared.");
}
//赋值
sMainLooper = myLooper();
}
}
再来看prepare()方法的具体实现
private static void prepare(boolean quitAllowed) {
//Looper是保存在sThreadLocal中的,如果sThreadLocal已经存在Looper了,直接抛出异常
if (sThreadLocal.get() != null) {
throw new RuntimeException("Only one Looper may be created per thread");
}
//创建一个Looper并保存至ThreadLocal
sThreadLocal.set(new Looper(quitAllowed));
}
注意:Looper的构造方法是私有的,这是为了防止开发者随意创建Looper,违反一个线程只有一个Looper的原则,如果你想自己在子线程创建Looper,只能使用:
/** Initialize the current thread as a looper.
* This gives you a chance to create handlers that then reference
* this looper, before actually starting the loop. Be sure to call
* {@link #loop()} after calling this method, and end it by calling
* {@link #quit()}.
*/
public static void prepare() {
prepare(true);
}
我们再来看Looper的构造方法,这里面也有点意思
//私有构造方法
private Looper(boolean quitAllowed) {
//在创建Looper的同时,顺带着把MessageQueue也创建了
mQueue = new MessageQueue(quitAllowed);
mThread = Thread.currentThread();
}
在Looper被创建的时候,MessageQueue也被创建了,这样保证了Looper和MessageQueue是一对一的关系
接下来就是loop()方法了,我们知道Message被放到MessageQueue中,至于Message是怎么被取出来的,这一部分的逻辑都包含在loop()方法中,按照惯例依然只会保留核心代码
public static void loop() {
//拿到looper对象,这个方法很简单,就是从threadlocal中获取到looper
final Looper me = myLooper();
if (me == null) {
throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread.");
}
//拿到MessageQueue的引用
final MessageQueue queue = me.mQueue;
// Make sure the identity of this thread is that of the local process,
// and keep track of what that identity token actually is.
Binder.clearCallingIdentity();
final long ident = Binder.clearCallingIdentity();
..........省略代码
//开启一个while(true)循环
for (;;) {
//不断地调用MessageQueue的next()方法获取Message对象
Message msg = queue.next(); // might block
if (msg == null) {
// No message indicates that the message queue is quitting.
return;
}
..........省略代码
try {
//msg对象不为空,开始分发出去,msg.target实际上就是一个Handler
msg.target.dispatchMessage(msg);
dispatchEnd = needEndTime ? SystemClock.uptimeMillis() : 0;
} finally {
if (traceTag != 0) {
Trace.traceEnd(traceTag);
}
}
..........省略代码
// Make sure that during the course of dispatching the
// identity of the thread wasn't corrupted.
final long newIdent = Binder.clearCallingIdentity();
..........省略代码
//msg对象使用完成,开始回收到Message池,方便下次使用
//因为Android系统中无时无刻不在大量地使用Message对象,所以创建一个池来维护Message对象,防止Message对象创建过多造成内存过高,这一点可以参考线程池
msg.recycleUnchecked();
}
}
核心的代码都加了注释,相信看一遍会有个大概的印象,但是这里我们依然有一个疑问,在主线程使用while(true),怎么能不出现ANR呢?
实际上这是一种阻塞式的轮询,我们在代码中写一个普通的死循环之所以会造成ANR是因为一直占用着cpu的资源没有释放,但是looper使用的是Linux下的epoll阻塞式模型,这个模型有两个特点
- 就是当内核缓冲区没有数据写入的时候,处理读出数据的线程就会阻塞调,并掉出了系统的调度队列,暂时不会去瓜分CPU宝贵的时间片了。
- 当内核缓冲区,有数据写入的时候,内核同样会发送事件,唤醒 处理读出数据的线程,重新开始工作。
个人感觉这个模型和java中的BlockingQueue非常相似,有兴趣的可以去研究一下
loop()方法中还有两个非常重要的地方:
- Message msg = queue.next(); 从MessageQueue中取出消息
- msg.target.dispatchMessage(msg); 消息的分发
Message next() {
// Return here if the message loop has already quit and been disposed.
// This can happen if the application tries to restart a looper after quit
// which is not supported.
final long ptr = mPtr;
if (ptr == 0) {
return null;
}
int pendingIdleHandlerCount = -1; // -1 only during first iteration
int nextPollTimeoutMillis = 0;
for (;;) {
..........省略代码
synchronized (this) {
// Try to retrieve the next message. Return if found.
final long now = SystemClock.uptimeMillis();
Message prevMsg = null;
Message msg = mMessages;
//如果msg.target不为空且msg.isAsynchronous(异步消息),优先取出
//只有系统消息才满足上述两个条件,所以会被优先处理
if (msg != null && msg.target == null) {
// Stalled by a barrier. Find the next asynchronous message in the queue.
do {
prevMsg = msg;
msg = msg.next;
} while (msg != null && !msg.isAsynchronous());
}
//将msg从链表中取出并赋值给全局变量mMessages
if (msg != null) {
if (now < msg.when) {
// Next message is not ready. Set a timeout to wake up when it is ready.
nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);
} else {
// Got a message.
mBlocked = false;
if (prevMsg != null) {
prevMsg.next = msg.next;
} else {
mMessages = msg.next;
}
msg.next = null;
if (DEBUG) Log.v(TAG, "Returning message: " + msg);
msg.markInUse();
//返回msg
return msg;
}
} else {
// No more messages.
nextPollTimeoutMillis = -1;
}
..................省略代码
}
..................省略代码
}
}
next()方法实际上就是在MessageQueue中拿到Message对象,只是在这里为了保证系统的流畅度,系统发送的Message会优先被取出,使用了一个do while循环做处理。由于我们使用Handler直接发送消息的时候Message的target属性总是会被赋值,而且我们不能直接调用MessageQueue中的enqueueMessage方法来将Message放入队列,因为这个方法是package-private,所以肯定不满足while中的条件,关于这两点我们后续还会讲到。
现在我们取出了Message对象,再来看看如何将Message回调到handler
msg.target.dispatchMessage(msg);
上面有讲到msg.target实际上就是一个Handler,所以我们直接去看Handler的dispatchMessage(Message msg)
/**
* Handle system messages here.
*/
public void dispatchMessage(Message msg) {
//回调1
if (msg.callback != null) {
handleCallback(msg);
} else {
//回调2
if (mCallback != null) {
if (mCallback.handleMessage(msg)) {
return;
}
}
//回调3
handleMessage(msg);
}
}
我们看到dispatchMessage一共有三种方式,那么这三种方式的先决条件是什么呢?我们来逐一分析
回调1:
msg.callback实际上是一个Runnable,我们在使用handler.post(Runnable r)的时候就会用到
public final boolean post(Runnable r)
{
//通过getPostMessage(r)将runnable封装成一个Message对象
return sendMessageDelayed(getPostMessage(r), 0);
}
private static Message getPostMessage(Runnable r) {
//在Message池中拿到一个Message
Message m = Message.obtain();
//给callback赋值
m.callback = r;
return m;
}
回调2:
mCallback又是什么呢?查看源码我们发现mCallback是Handler中的一个内部接口,我们在new Handler的时候有时候会这么写:
Handler handler = new Handler(new Handler.Callback() {
@Override
public boolean handleMessage(Message msg) {
return false;
}
});
如果在构造方法中传入了一个Handler.Callback,自然而然地会给mCallback赋值。
我们再来看看这个接口函数的返回值有什么含义?
public interface Callback {
/**
* @param msg A {@link android.os.Message Message} object
* @return 返回true表示没有额外的事情需要处理
*/
public boolean handleMessage(Message msg);
}
结合着dispatchMessage方法来看,如果在回调2中返回true,那么回调3将不会执行。所以我们很好奇回调3又是什么东西呢?实际上这是很多开发者最常用的一种方式,具体的用法如下:
Handler handler = new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
}
};
再来源码中handleMessage(Message msg)是怎么的存在
/**
* Subclasses must implement this to receive messages.
*/
public void handleMessage(Message msg) {
}
啥都没有,就是一个空实现! 这个方法就是用于给子类重写的。
以上我们分析了Handler机制中如何通过Looper和MessageQueue取消息,然后回调到handlerMessage中,下面我们再来看看Message是怎么放入MessageQueue的。
1.2 Handler如何发送消息
Handler发送消息的姿势简直太多了,最大程度上满足了我们的需求,列举出下面几种方式
handler.post(new Runnable() {
@Override
public void run() { }});
handler.sendMessage(msg);
handler.sendMessageDelayed(msg, 1000);
handler.sendEmptyMessageAtTime(1, SystemClock.uptimeMillis());
handler.sendEmptyMessage(1);
............
面对如此众多的姿势,handler是如何应对的呢?
我们查看源码发现,无论发送的姿势太多,handler都会将这些消息封装成一个Message,并对相应的属性赋值。实际上所有的发送方法都会走到enqueueMessage()
private boolean enqueueMessage(MessageQueue queue, Message msg, long uptimeMillis) {
msg.target = this;
if (mAsynchronous) {
msg.setAsynchronous(true);
}
return queue.enqueueMessage(msg, uptimeMillis);
}
通过调用MessageQueue的enqueueMesssage方法将Message放入队列
boolean enqueueMessage(Message msg, long when) {
if (msg.target == null) {
throw new IllegalArgumentException("Message must have a target.");
}
if (msg.isInUse()) {
throw new IllegalStateException(msg + " This message is already in use.");
}
synchronized (this) {
if (mQuitting) {
IllegalStateException e = new IllegalStateException(
msg.target + " sending message to a Handler on a dead thread");
Log.w(TAG, e.getMessage(), e);
msg.recycle();
return false;
}
msg.markInUse();
msg.when = when;
Message p = mMessages;
boolean needWake;
if (p == null || when == 0 || when < p.when) {
// New head, wake up the event queue if blocked.
msg.next = p;
mMessages = msg;
needWake = mBlocked;
} else {
// Inserted within the middle of the queue. Usually we don't have to wake
// up the event queue unless there is a barrier at the head of the queue
// and the message is the earliest asynchronous message in the queue.
needWake = mBlocked && p.target == null && msg.isAsynchronous();
Message prev;
for (;;) {
prev = p;
p = p.next;
if (p == null || when < p.when) {
break;
}
if (needWake && p.isAsynchronous()) {
needWake = false;
}
}
msg.next = p; // invariant: p == prev.next
prev.next = msg;
}
// We can assume mPtr != 0 because mQuitting is false.
if (needWake) {
nativeWake(mPtr);
}
}
return true;
}
这边通过enqueueMessage存入消息,另外一边looper又在不断地取消息,我们整个handler机制就这样跑起来了。
但是看到这里我还是有疑问呀,Handler怎么就拿到了MessageQueue的引用了?我们再来看看Handler的构造方法
ublic Handler(Callback callback, boolean async) {
if (FIND_POTENTIAL_LEAKS) {
final Class<? extends Handler> klass = getClass();
if ((klass.isAnonymousClass() || klass.isMemberClass() || klass.isLocalClass()) &&
(klass.getModifiers() & Modifier.STATIC) == 0) {
Log.w(TAG, "The following Handler class should be static or leaks might occur: " +
klass.getCanonicalName());
}
}
//获取到线程中唯一的looper对象
mLooper = Looper.myLooper();
if (mLooper == null) {
throw new RuntimeException(
"Can't create handler inside thread " + Thread.currentThread()
+ " that has not called Looper.prepare()");
}
//通过looper获取到MessageQueue
mQueue = mLooper.mQueue;
mCallback = callback;
mAsynchronous = async;
}
这样看是不是恍然大悟呀,至此:Handler,MessageQueue,Looper,ThreadLocal已经全部串联起来了,至于Message,个人认为这就是一个消息体,在Handler,MQ,Looper中飞来飞去而已。不过Message中还是有很多有意思的方法和属性,这个大家有兴趣的可以去看源码。
2. 手写Handler
一开始想着把这部分也写到这篇博客,但是觉得篇幅过长,所以分开了。有兴趣的可以查看