概要
- 列表、元组操作
- 字符串操作
- 字典操作
- 集合操作
- 文件操作
- 字符编码与转码
一、列表、元祖操作
列表是我们最以后最常用的数据类型之一,通过列表可以对数据实现最方便的存储、修改等操作
定义列表
1 names = ['Richard',"Tom",'Jack']
通过下标访问列表中的元素,下标从0开始计数
1 >>> names[0] 2 'Richard' 3 >>> names[2] 4 'Jack' 5 >>> names[-1] 6 'Jack' 7 >>> names[-2] #还可以倒着取 8 'Tom'
切片:取多个元素

1 >>> names = ["Richard", "Tom", "Jack", "Rain", "Bob", "Amy"] 2 >>> names[1:4] #取下标1至下标4之间的数字,包括1,不包括4 3 ['Tom', 'Jack', 'Rain'] 4 >>> names[1:-1] #取下标1至-1的值,不包括-1 5 ['Tom', 'Jack', 'Rain', 'Bob'] 6 >>> names[0:3] 7 ['Richard', 'Tom', 'Jack'] 8 >>> names[:3] #如果是从头开始取,0可以忽略,跟上句效果一样 9 ['Richard', 'Tom', 'Jack'] 10 >>> names[3:] #如果想取最后一个,必须不能写-1,只能这么写 11 ['Rain', 'Bob', 'Amy'] 12 >>> names[3:-1] #这样-1就不会被包含了 13 ['Rain', 'Bob'] 14 >>> names[0::2] #后面的2是代表,每隔一个元素,就取一个 15 ['Richard', 'Jack', 'Bob'] 16 >>> names[::2] #和上一句效果一样 17 ['Richard', 'Jack', 'Bob']
追加

1 >>> names = ["Richard", "Tom", "Jack", "Rain", "Bob", "Amy"] 2 >>> names.append("Jim") 3 >>> names 4 ['Richard', 'Tom', 'Jack', 'Rain', 'Bob', 'Amy', 'Jim']
插入

1 >>> names 2 ['Richard', 'Tom', 'Jack', 'Rain', 'Bob', 'Amy', 'Jim'] 3 >>> names.insert(2,"强行从Jack前面插入") 4 >>> names 5 ['Richard', 'Tom', '强行从Jack前面插入', 'Jack', 'Rain', 'Bob', 'Amy', 'Jim'] 6 >>> names.insert(5,"从Jack后面插入") 7 >>> names 8 ['Richard', 'Tom', '强行从Jack前面插入', 'Jack', 'Rain', '从Jack后面插入', 'Bob', 'Amy', 'Jim'] 9 >>>
修改

1 >>> names 2 ['Richard', 'Tom', '强行从Jack前面插入', 'Jack', 'Rain', '从Jack后面插入', 'Bob', 'Amy', 'Jim'] 3 >>> names[2] = "该换人了" 4 >>> names 5 ['Richard', 'Tom', '该换人了', 'Jack', 'Rain', '从Jack后面插入', 'Bob', 'Amy', 'Jim'] 6 >>>
删除

1 >>> del names[2] #删除指定位置的值 2 >>> names 3 ['Richard', 'Tom', 'Jack', 'Rain', '从Jack后面插入', 'Bob', 'Amy', 'Jim'] 4 >>> del names[4] 5 >>> names 6 ['Richard', 'Tom', 'Jack', 'Rain', 'Bob', 'Amy', 'Jim'] 7 8 >>> names.remove("Tom") #删除指定元素 9 >>> names 10 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy', 'Jim'] 11 12 >>> names.pop() #删除列表最后一个值,并返回结果 13 'Jim' 14 >>> names 15 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy']
扩展

1 >>> names 2 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy'] 3 >>> b = [1,2,3] 4 >>> names.extend(b) 5 >>> names 6 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy', 1, 2, 3]
拷贝

1 >>> names 2 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy', 1, 2, 3] 3 >>> name_copy = names.copy() 4 >>> name_copy 5 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy', 1, 2, 3]
关于浅拷贝和深拷贝后面再补充。。。
扫描二维码关注公众号,回复:
7683445 查看本文章
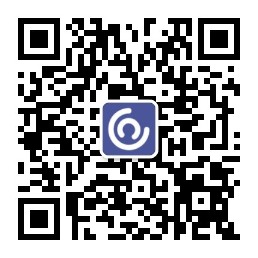
统计

1 >>> names 2 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy', 1, 2, 3] 3 >>> names.count("Richard") 4 1
排序&翻转

1 >>> names 2 ['Richard', 'Jack', 'Rain', 'Bob', 'Amy', 1, 2, 3] 3 >>> names.insert(2,"Bob") 4 >>> names.insert(6,"Andy") 5 >>> names 6 ['Richard', 'Jack', 'Bob', 'Rain', 'Bob', 'Amy', 'Andy', 1, 2, 3] 7 >>> names.sort() #排序 8 Traceback (most recent call last): 9 File "<pyshell#36>", line 1, in <module> 10 names.sort() #排序 11 TypeError: '<' not supported between instances of 'int' and 'str' #3.0里不同数据类型不能放在一起排序了,擦 12 >>> names[-1] = "3" 13 >>> names[-2] = "2" 14 >>> names[-3] = "1" 15 >>> names 16 ['Amy', 'Andy', 'Bob', 'Bob', 'Jack', 'Rain', 'Richard', '1', '2', '3'] 17 >>> names.sort() 18 >>> names 19 ['1', '2', '3', 'Amy', 'Andy', 'Bob', 'Bob', 'Jack', 'Rain', 'Richard'] 20 >>> 21 >>> names.reverse() 22 >>> names 23 ['Richard', 'Rain', 'Jack', 'Bob', 'Bob', 'Andy', 'Amy', '3', '2', '1']
获取下标

1 >>> names 2 ['Richard', 'Rain', 'Jack', 'Bob', 'Bob', 'Andy', 'Amy', '3', '2', '1'] 3 >>> names.index("Richard") 4 0 5 >>> names.index("Bob") #只返回找到的第一个值的下标 6 3
元组
元组其实跟列表差不多,也是存一组数,只不是它一旦创建,便不能再修改,所以又叫只读列表
语法
1 names = ("alex","jack","eric")
它只有2个方法,一个是count,一个是index,完毕。
程序练习
程序:购物车程序
需求:
- 启动程序后,让用户输入工资,然后打印商品列表
- 允许用户根据商品编号购买商品
- 用户选择商品后,检测余额是否够,够就直接扣款,不够就提醒
- 可随时退出,退出时,打印已购买商品和余额

1 print('----------欢迎进入自助购物。----------') 2 golds_list = [ 3 ('macbook', 12000), 4 ('iphone', 5800), 5 ('book', 15), 6 ('coffee', 35), 7 ('glass', 20), 8 ('bike', 1000) 9 ] #定义一个商品列表 10 shopping_car = [] #定义一个空的购物车列表 11 12 while True: 13 salary = input('请输入余额:') 14 if salary.isdigit(): #判断输入值是否是数字 15 salary = int(salary) #如果是数字则转成整数型 16 while True: 17 for id,p_item in enumerate(golds_list): #打印出商品列表,enumerate()方法是按编号输出 18 print(id+1, p_item) 19 user_choice = input('请输入所购商品编号(退出请按:q/Q):') #提示用户输入搜购买商品编号,或按q/Q退出 20 if user_choice.isdigit(): #判断输入值是否是数字 21 user_choice = int(user_choice) #如果是数字则转成整数型 22 if user_choice > 0 and user_choice < len(golds_list): #判断输入的编号是否在商品列表范围之内 23 p_item = golds_list[user_choice - 1] 24 if salary >= p_item[1]: #判断余额是否大于输入编号所对应商品的价钱 25 salary -= p_item[1] #成立则余额对应减少 26 print('已添加{0}至购物车,当前余额为{1}'.format(p_item[0], salary)) 27 shopping_car.append(p_item) #将所选商品存入"购物车"列表 28 else: 29 print('余额不足,无法购买,请选择其他商品!') 30 continue 31 else: 32 print('请输入正确的商品编号,或输入q/Q退出') 33 continue 34 elif user_choice == 'q' or user_choice == 'Q': #输入q/Q则退出,并打印所购商品以及余额 35 print('--------shopping lists--------') 36 for id, i in enumerate(shopping_car): 37 print(id+1, i) 38 print('所剩余额为:{}。'.format(salary)) 39 print('--------shopping end--------') 40 exit() #退出程序 41 else: 42 print('格式有误,请重新输入!') 43 continue 44 else: 45 print('格式有误,请重新输入!') 46 continue
二、字符串操作
特性:不可修改

1 name.capitalize() 首字母大写 2 name.casefold() 大写全部变小写 3 name.center(50,"-") 输出 '---------------------Alex Li----------------------' 4 name.count('lex') 统计 lex出现次数 5 name.encode() 将字符串编码成bytes格式 6 name.endswith("Li") 判断字符串是否以 Li结尾 7 "Alex\tLi".expandtabs(10) 输出'Alex Li', 将\t转换成多长的空格 8 name.find('A') 查找A,找到返回其索引, 找不到返回-1 9 10 format : 11 >>> msg = "my name is {}, and age is {}" 12 >>> msg.format("alex",22) 13 'my name is alex, and age is 22' 14 >>> msg = "my name is {1}, and age is {0}" 15 >>> msg.format("alex",22) 16 'my name is 22, and age is alex' 17 >>> msg = "my name is {name}, and age is {age}" 18 >>> msg.format(age=22,name="ale") 19 'my name is ale, and age is 22' 20 format_map 21 >>> msg.format_map({'name':'alex','age':22}) 22 'my name is alex, and age is 22' 23 24 25 msg.index('a') 返回a所在字符串的索引 26 '9aA'.isalnum() True 27 28 '9'.isdigit() 是否整数 29 name.isnumeric 30 name.isprintable 31 name.isspace 32 name.istitle 33 name.isupper 34 "|".join(['alex','jack','rain']) 35 'alex|jack|rain' 36 37 38 maketrans 39 >>> intab = "aeiou" #This is the string having actual characters. 40 >>> outtab = "12345" #This is the string having corresponding mapping character 41 >>> trantab = str.maketrans(intab, outtab) 42 >>> 43 >>> str = "this is string example....wow!!!" 44 >>> str.translate(trantab) 45 'th3s 3s str3ng 2x1mpl2....w4w!!!' 46 47 msg.partition('is') 输出 ('my name ', 'is', ' {name}, and age is {age}') 48 49 >>> "alex li, chinese name is lijie".replace("li","LI",1) 50 'alex LI, chinese name is lijie' 51 52 msg.swapcase 大小写互换 53 54 55 >>> msg.zfill(40) 56 '00000my name is {name}, and age is {age}' 57 58 59 60 >>> n4.ljust(40,"-") 61 'Hello 2orld-----------------------------' 62 >>> n4.rjust(40,"-") 63 '-----------------------------Hello 2orld' 64 65 66 >>> b="ddefdsdff_哈哈" 67 >>> b.isidentifier() #检测一段字符串可否被当作标志符,即是否符合变量命名规则 68 True
三、字典操作
字典一种key - value 的数据类型,使用就像我们上学用的字典,通过笔划、字母来查对应页的详细内容。
语法:
info = { 'stu1101': "Richard", 'stu1102': "Alex", 'stu1103': "William", }
字典的特性:
- dict是无序的
- key必须是唯一的,so 天生去重
增加

1 >>> info["stu1104"] = "Rain" 2 >>> info 3 {'stu1102': 'Alex', 'stu1104': 'Rain', 'stu1103': 'William', 'stu1101': 'Richard'}
修改

1 >>> info['stu1101'] = "理查德" 2 >>> info 3 {'stu1102': 'Alex', 'stu1103': 'William', 'stu1101': '理查德'}
删除

1 >>> info = { 2 'stu1101': "Richard", 3 'stu1102': "Alex", 4 'stu1103': "William", 5 } 6 >>> info 7 {'stu1101': 'Richard', 'stu1102': 'Alex', 'stu1103': 'William'} 8 >>> info.pop("stu1101")#第一种,删除指定的内容,返回删除的值 9 'Richard' 10 >>> info 11 {'stu1102': 'Alex', 'stu1103': 'William'} 12 >>> del info['stu1103'] #第二种,删除后无返回值 13 >>> info 14 {'stu1102': 'Alex'} 15 >>> info = {'stu1101': "Richard",'stu1102': "Alex",'stu1103': "William",} 16 >>> info 17 {'stu1101': 'Richard', 'stu1102': 'Alex', 'stu1103': 'William'} 18 >>> info.popitem() #第三种,随机删除一个,并返回结果 19 ('stu1103', 'William') 20 >>> info 21 {'stu1101': 'Richard', 'stu1102': 'Alex'}
查找

1 >>> info = {'stu1101': "Richard",'stu1102': "Alex",'stu1103': "William",} 2 >>> "stu1102" in info #第一种,返回布尔值 3 True 4 >>> info.get('stu1102') #第二种,获取并返回其值(常用) 5 'Alex' 6 >>> info['stu1102'] #第三种,同上,但是如果key不在则会报错 7 'Alex' 8 >>> info['stu1105'] 9 Traceback (most recent call last): 10 File "<pyshell#68>", line 1, in <module> 11 info['stu1105'] 12 KeyError: 'stu1105' 13 >>>
多级字典嵌套及操作

1 >>> city= { 2 "北京市":{ 3 "大兴区": ["亦庄镇"], 4 "朝阳区": ["朝阳群众贼厉害!"], 5 "东城区": ["天安门广场","王府井大街"] 6 }, 7 "广州市":{ 8 "海珠区":["广州塔","夜晚还是挺漂亮的!"] 9 }, 10 "深圳市":{ 11 "福田区":["平安大厦","港铁4号线"] 12 } 13 } 14 >>> city["广州市"]["海珠区"][1] += ",一起去饮茶!" 15 >>> city["广州市"]["海珠区"] 16 ['广州塔', '夜晚还是挺漂亮的!,一起去饮茶!']
其它姿势

1 >>> info = {'stu1101':'Richard','stu1102':'Andy'} 2 >>> info 3 {'stu1101': 'Richard', 'stu1102': 'Andy'} 4 >>> info.values() #得到所有字典的value 5 dict_values(['Richard', 'Andy']) 6 7 >>> info.keys() #得到所有字典的key 8 dict_keys(['stu1101', 'stu1102']) 9 10 >>> info.setdefault('stu1105','Yucoo') #如果key不存在字典中,则添加此键 11 'Yucoo' 12 13 >>> info 14 {'stu1101': 'Richard', 'stu1102': 'Andy', 'stu1105': 'Yucoo'} 15 >>> info.setdefault('stu1102','安迪') #如果key存在,则返回key值 16 'Andy' 17 >>> info 18 {'stu1101': 'Richard', 'stu1102': 'Andy', 'stu1105': 'Yucoo'} 19 >>> 20 >>> info 21 {'stu1101': 'Richard', 'stu1102': 'Andy', 'stu1105': 'Yucoo'} 22 >>> b = {'stu1102':'安迪',1:2,3:4} 23 >>> info.update(b) #把字典2中的keys/values更新到字典1中,有重复的key时,覆盖字典1中的value 24 >>> info 25 {'stu1101': 'Richard', 'stu1102': '安迪', 'stu1105': 'Yucoo', 1: 2, 3: 4} 26 >>> 27 >>> 28 >>> info.items() #转换成列表 29 dict_items([('stu1101', 'Richard'), ('stu1102', '安迪'), ('stu1105', 'Yucoo'), (1, 2), (3, 4)])
循环dict
1 #方法1 2 for key in info: #推荐使用,效率跟高 3 print(key,info[key]) 4 5 #方法2 6 for k,v in info.items(): #会先把dict转成list,数据里大时莫用 7 print(k,v)
程序练习
程序: 三级菜单
要求:
- 打印省、市、县三级菜单
- 可返回上一级
- 可随时退出程
代码待提交!!!
四、集合操作
集合是一个无序的,不重复的数据组合,它的主要作用如下:
- 去重,把一个列表变成集合,就自动去重了
- 关系测试,测试两组数据之前的交集、差集、并集等关系
常用操作

1 s = set([3,5,9,10]) #创建一个数值集合 2 3 t = set("Hello") #创建一个唯一字符的集合 4 5 6 a = t | s # t 和 s的并集 7 8 b = t & s # t 和 s的交集 9 10 c = t – s # 求差集(项在t中,但不在s中) 11 12 d = t ^ s # 对称差集(项在t或s中,但不会同时出现在二者中) 13 14 15 16 基本操作: 17 18 t.add('x') # 添加一项 19 20 s.update([10,37,42]) # 在s中添加多项 21 22 23 24 使用remove()可以删除一项: 25 26 t.remove('H') 27 28 29 len(s) 30 set 的长度 31 32 x in s 33 测试 x 是否是 s 的成员 34 35 x not in s 36 测试 x 是否不是 s 的成员 37 38 s.issubset(t) 39 s <= t 40 测试是否 s 中的每一个元素都在 t 中 41 42 s.issuperset(t) 43 s >= t 44 测试是否 t 中的每一个元素都在 s 中 45 46 s.union(t) 47 s | t 48 返回一个新的 set 包含 s 和 t 中的每一个元素 49 50 s.intersection(t) 51 s & t 52 返回一个新的 set 包含 s 和 t 中的公共元素 53 54 s.difference(t) 55 s - t 56 返回一个新的 set 包含 s 中有但是 t 中没有的元素 57 58 s.symmetric_difference(t) 59 s ^ t 60 返回一个新的 set 包含 s 和 t 中不重复的元素 61 62 s.copy() 63 返回 set “s”的一个浅复制
五、文件操作
对文件操作流程
- 打开文件,得到文件句柄并赋值给一个变量
- 通过句柄对文件进行操作
- 关闭文件