Write an algorithm to determine if a number is "happy".
A happy number is a number defined by the following process: Starting with any positive integer, replace the number by the sum of the squares of its digits, and repeat the process until the number equals 1 (where it will stay), or it loops endlessly in a cycle which does not include 1. Those numbers for which this process ends in 1 are happy numbers.
Example: 19 is a happy number
- 12 + 92 = 82
- 82 + 22 = 68
- 62 + 82 = 100
- 12 + 02 + 02 = 1
我的解法:每次计算出和,判断是否为1,不为1则判断是否在set里(在set里,则跟之前的重复,若重复了,那么永远不能为1了,返回false),
class Solution {
public boolean isHappy(int n) {Set<Integer> set=new HashSet<Integer>();
int sum=n;
while (true) {
n=sum;
sum = 0;
while (n > 0) {
sum += Math.pow(n % 10, 2);
n = n / 10;
}
if(sum==1)
return true;
else
{
if(set.contains(sum))
return false;
else
set.add(sum);
}
}
}
}
不占用额外空间的c++算法,fast和slow分别作为指针,fast每次走两步,slow只走一步,等到fast追上slow时,判断slow是否为1,用时间换空间
作者,
Freezen
扫描二维码关注公众号,回复:
762544 查看本文章
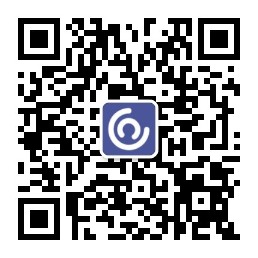
int digitSquareSum(int n) {
int sum = 0, tmp;
while (n) {
tmp = n % 10;
sum += tmp * tmp;
n /= 10;
}
return sum;
}
bool isHappy(int n) {
int slow, fast;
slow = fast = n;
do {
slow = digitSquareSum(slow);
fast = digitSquareSum(fast);
fast = digitSquareSum(fast);
} while(slow != fast);
if (slow == 1) return 1;
else return 0;
}