目录
前言
非常适合练手小游戏,主要使用jQuery实现dom操作和动画效果。通过练习这个小游戏我对JS的了解更加深入了,也熟悉了jQuery事件、动画、回调函数的应用。
预览
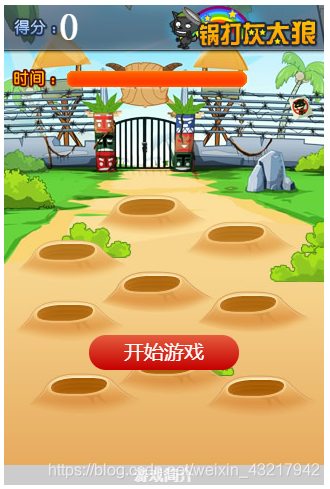
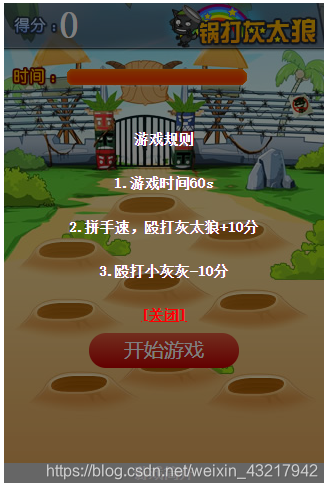
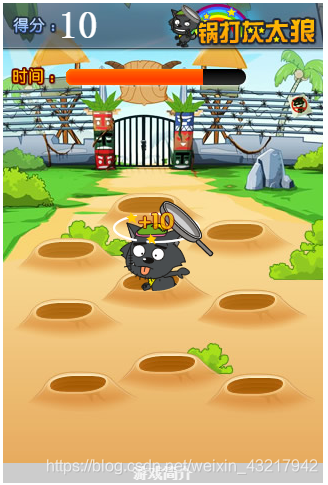
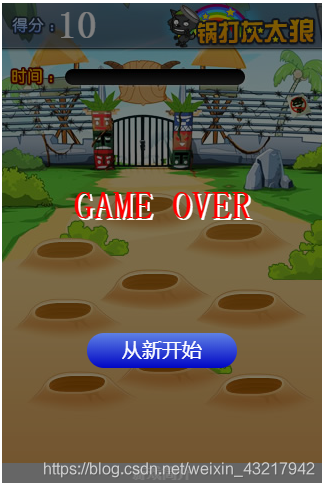
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>狂拍灰太狼</title>
<meta name="viewport" content="width=device-width,shrink-to-fit=no">
<link rel="stylesheet" href="css/index.css">
<script src="js/jquery-1.12.4.js"></script>
<script src="js/index.js"></script>
</head>
<body>
<div class="container">
<span class="score">0</span>
<div class="process"></div>
<button class="start">开始游戏</button>
<div class="rules">
<p>游戏简介</p>
</div>
<div class="rule">
<p>游戏规则</p>
<p>1.游戏时间60s</p>
<p>2.拼手速,殴打灰太狼+10分</p>
<p>3.殴打小灰灰-10分</p>
<a href="javascript:void(0)">[关闭]</a>
</div>
<div class="mask">
<h1>GAME OVER</h1>
<button class="restart">从新开始</button>
</div>
</div>
</body>
</html>
CSS代码
*{
padding:0;
margin:0;
}
body{
font-family: Serif,"Microsoft YaHei UI",-apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, "Helvetica Neue", Arial, "Noto Sans", sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji";
font-size: 14px;
color: white;
}
.container{
width: 320px;
height: 480px;
background: url("../images/game_bg.jpg") no-repeat 0 0;
margin: 20px auto;
position: relative;
}
.container>.score{
display: block;
width: 100px;
position: relative;
font-size: 38px;
font-weight: bold;
left:55px;
}
.container>.process{
background: url("../images/progress.png");
width: 180px;
height: 16px;
position: relative;
left: 63px;
top:23px;
}
.container>.start{
width: 150px;
line-height: 35px;
color:white;
background: linear-gradient(#e55c3d,#c50000);
border-radius:20px;
border: none;
font-size: 20px;
position:absolute;
top:330px;
left:50%;
margin-left:-75px;
}
.container>.rules{
width:100%;
height:20px;
background: #CCC;
position:absolute;
left:0;
bottom:0;
}
.container>.rules>p{
width: 100%;
height: 100%;
line-height: 20px;
text-align: center;
font-weight: bold;
color: whitesmoke;
}
.container>.rule{
width: 100%;
height: 100%;
background: rgba(0,0,0,0.5);
padding: 100px 0;
box-sizing: border-box;
position: absolute;
left: 0;
top:0;
display: none;
}
.container>.rule>*{
text-align: center;
margin-top: 28px;
font-weight: bold;
user-select: none;
}
.container>.rule>a{
display: block;
width: 60px;
margin: 28px auto;
text-align: center;
color: red;
}
.container>.mask{
width: 100%;
height: 100%;
background: rgba(0,0,0,0.5);
padding: 100px 0;
box-sizing: border-box;
position: absolute;
left: 0;
top:0;
display: none;
}
.container>.mask>.restart{
width: 150px;
line-height: 35px;
color:white;
background: linear-gradient(#5e81e5, #0006c5);
border-radius:20px;
border: none;
font-size: 20px;
position:absolute;
top:330px;
left:50%;
margin-left:-75px;
}
.container>.mask>h1{
text-align: center;
margin-top: 80px;
color:red;
font-size: 38px;
font-weight: bold;
text-shadow: 2px 2px 0 white;
}
JS/jQuery代码
$(function () {
var $rule =$(".container>.rule");//所有规则展示面板
var $mask =$(".container>.mask"); //游戏结束
var $start = $(".container>.start");
var $restart = $(".container>.mask>.restart");
var $rules = $(".container>.rules");//游戏简介
//1.监听游戏规则点击
$rules.click(function () {
$rule.fadeIn(400,function () {
console.log("打开游戏介绍");
});
});
//2.监听游戏规则关闭点击
$(".container>.rule>a").click(function () {
$rule.fadeOut(400,function () {
console.log("关闭游戏介绍");
})
});
//3.监听开始按钮的点击
$start.click(function () {
$(this).stop().fadeOut(300,function () {
console.log("开始游戏");
});
processHandle();
startWolfAnimation();
});
//4.监听重新开始按钮
$restart.click(function () {
//关闭游戏结束面板(mask)
$mask.fadeOut(300,function () {
console.log("重新开始游戏")
});
//恢复进度条
processHandle();
//重新开始动画
startWolfAnimation();
//分数清零
score=0;
});
var $process = $(".container>.process");//时间进度条
//定义处理进度条方法
function processHandle() {
//设置进度条为100%状态
$process.css("width","180px");
var timer = setInterval(function () {
//拿到进度条当前的宽度
var procressWidth = $process.width();
//减少当前宽度
procressWidth-=1;
//从新赋值
$process.css("width",procressWidth);
//判断进度条是否走完
if(procressWidth<=0){
//清除定时器
clearInterval(timer);
//显示游戏结束画面
$mask.fadeIn(300,function () {
console.log("游戏结束");
});
stopWolfAnimation();
}
},100);
}
// 1.定义两个数组保存所有灰太狼和小灰灰的图片
var wolf_1=['./images/h0.png','./images/h1.png','./images/h2.png','./images/h3.png','./images/h4.png','./images/h5.png','./images/h6.png','./images/h7.png','./images/h8.png','./images/h9.png'];
var wolf_2=['./images/x0.png','./images/x1.png','./images/x2.png','./images/x3.png','./images/x4.png','./images/x5.png','./images/x6.png','./images/x7.png','./images/x8.png','./images/x9.png'];
// 2.定义一个数组保存所有可能出现的位置
var arrPos = [
{left:"100px",top:"115px"},
{left:"20px",top:"160px"},
{left:"190px",top:"142px"},
{left:"105px",top:"193px"},
{left:"19px",top:"221px"},
{left:"202px",top:"212px"},
{left:"120px",top:"275px"},
{left:"30px",top:"295px"},
{left:"209px",top:"297px"}
];
var wolfTimer;
var wolfPos;
var wolfType;
//处理狼运动的方法
function startWolfAnimation(){
wolfPos = Math.round(Math.random()*8);
wolfType = Math.round(Math.random()*2)===1?wolf_1:wolf_2;
//生成狼jQuery dom对象
var $wolfImage = $("<img src='' class='wolfImage' alt=''>");
$wolfImage.css({
position:"absolute",
left:arrPos[wolfPos].left,
top:arrPos[wolfPos].top,
display:"none"
});
//声明全局变量,此处的挂载算var声明添加
window.wolfIndex = 0;
window.wolfIndexEnd = 5;
//将图片添加到界面容器中
$(".container").append($wolfImage);
$wolfImage.fadeIn(300);
wolfTimer = setInterval(function () {
if(wolfIndex>wolfIndexEnd){
$wolfImage.stop().remove();
clearInterval(wolfTimer);
startWolfAnimation();
}
$wolfImage.attr("src",wolfType[wolfIndex]);
wolfIndex++;
},200);
//判断加分或减分
judgeRule($wolfImage);
}
// console.log(Math.round(Math.random()*8));
function stopWolfAnimation() {
$(".wolfImage").stop().fadeOut(400).remove();
clearInterval(wolfTimer);
}
var score = 0;
var $score = $(".container>.score");
function judgeRule(wolf){
$(wolf).one("click",function () {
//修改索引,播放打击效果。
window.wolfIndex = 5;
window.wolfIndexEnd = 9;
//取得点击图片地址
var $src = $(this).attr("src");
//根据图片地址判断是否是灰太狼
var booFlog = $src.indexOf("h")>-1;//在得到的地址中查找是否包含h,如果返回的索引值大于-1说明找到了
if(booFlog){
$score.text(score+=10);
}else{
$score.text(score-=10);
}
});
}
});
源码:https://download.csdn.net/download/weixin_43217942/11875294
PS:希望对你有帮助,欢迎评论,求点赞,你的认可是我写作的最大动力~~