不知不觉用 Go 开发也两年多了. 筹备点经验汇总, 方便后面的同学能快速上手.
提纲
1. Go 安装
2. Go ide 搭建
3. Go modules 模块管理
4. Go unit test
5. Go debug 调试
6. Go pprof 火焰图
7. Go online 调试
8. Go future 思考
1. Go 安装
Golang 官网 https://golang.org/
Download 页面 https://golang.org/dl/
Install 页面 https://golang.org/doc/install
登录 Golang 官网. 如果访问有问题, 请激发这辈子最大潜力去深入突破此间魔障.
别问
问
就是不合适
别多想
想
就是没缘分
然后[2019/10/21]点到下载页面, 这里下载的是 go1.12.12.linux-amd64.tar.gz
随后按照安装页面提示操作. 这里简单梳理以下脚本
sudo rm -rf /usr/local/go
sudo tar -C /usr/local -xzf go1.12.12.linux-amd64.tar.gz
sudo vi /etc/profile
Shift + G
i
export PATH=$PATH:/usr/local/go/bin
~
wq!
source /etc/profile
通过 go version 查看安装版本

原本是想通过 window 平台带大家演示一遍提纲中内容. 不过经常待在 ubuntu 旁边,
只能顺手用 linux 环境给同学演示一遍. 有心人可自行选择环境实操, 思路都差不多.
2. Go ide 搭建
Download VSCode https://code.visualstudio.com/
这里推荐 VSCode 搭建 Golang 开发换机, 下载 deb 包并安装.
sudo dpkg -i code_1.39.2-1571154070_amd64.deb
筹备下面环境
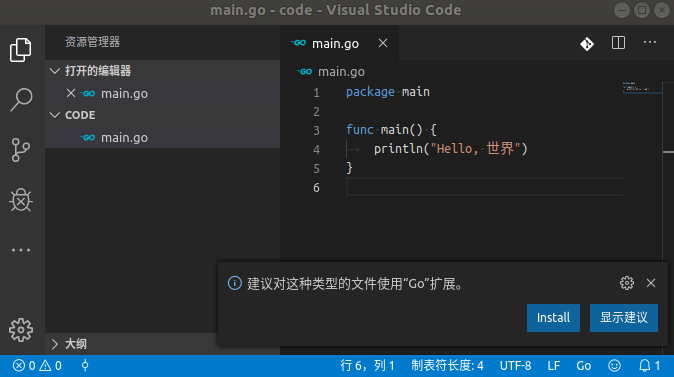
点击 [Install] -End-> [F1] -> [Go: Install/Update Tools] -> [全选] -> [确定]
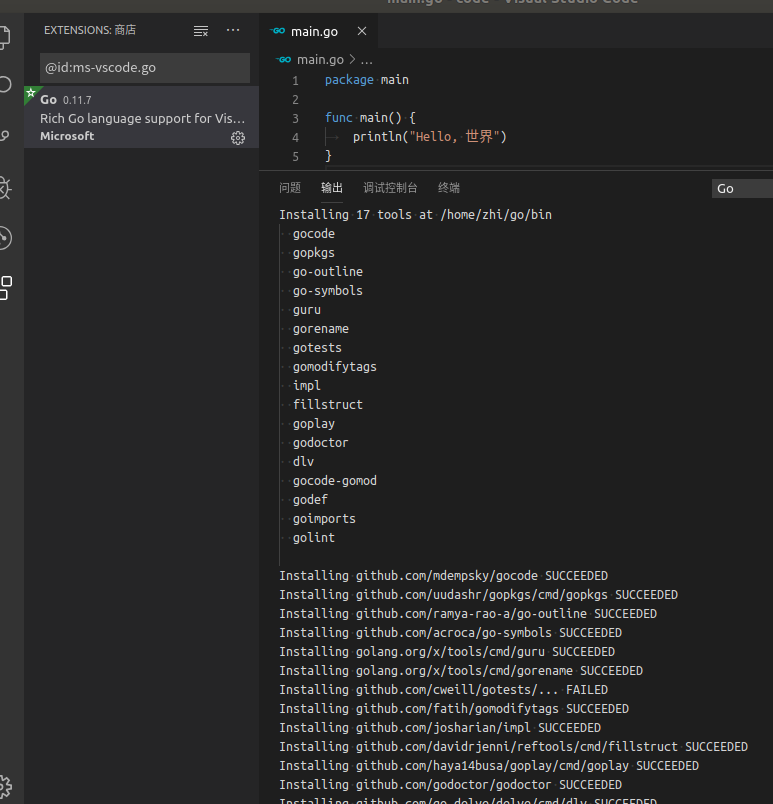
大概齐都成功后, 对着项目来下 [F5] 跑起来
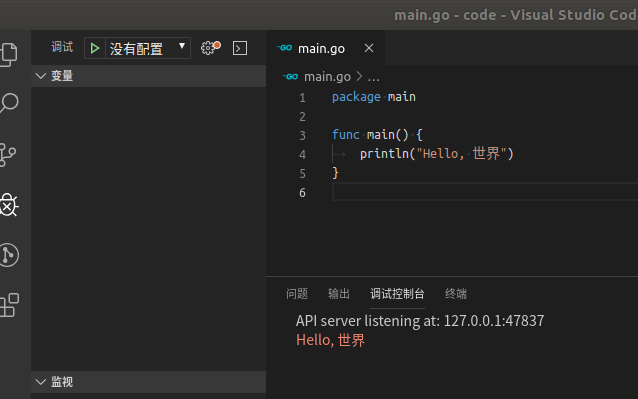
别惊讶, 咱们的 IDE 已经 OK 了!
3. Go modules 模块管理
Go modules 是 Go 解决包依赖管理方面特别棒的一次尝试. 这里带大家简单先用起来.
有心的同学可以查询更多的相关资料, 或者通过 go mod help 自行晋升.
开始准备素材
code/arithmetic/div.go
package arithmetic
import "errors"
// Div 除法操作
func Div(a, b int) (c int, err error) {
if b == 0 {
err = errors.New("divisor is 0")
return
}
c = a / b
return
}
code/main.go
package main
import (
"fmt"
"code/arithmetic"
)
func main() {
println("Hello, 世界")
c, err := arithmetic.Div(1, 0)
fmt.Printf("c = %d, err = %+v\n", c, err)
}
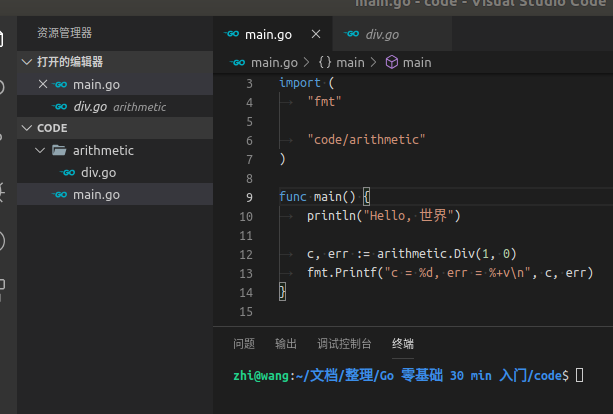
有了上面这些, 开始着手构建
go mod init code
go build
./code
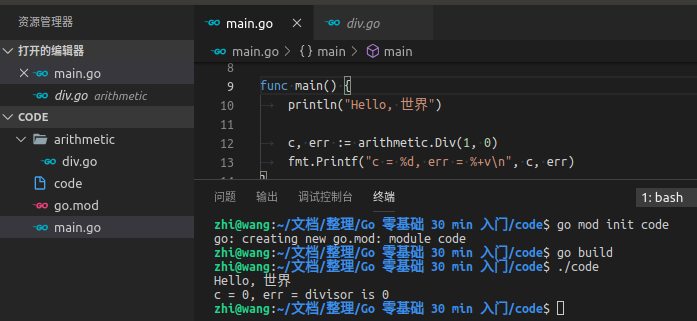
越纯粹越懂自己
4. Go unit test
code/arithmetic/div_test.go
package arithmetic
import (
"testing"
"math/rand"
)
func TestDiv(t *testing.T) {
var (
a, b, c int
err error
)
a, b = 1, 2
c, err = Div(a, b)
t.Logf("a = %d, b = %d, c = %d, err = %+v", a, b, c, err)
a, b = 1, 0
c, err = Div(a, b)
t.Logf("a = %d, b = %d, c = %d, err = %+v", a, b, c, err)
}
func BenchmarkDiv(b *testing.B) {
for i := 0; i < b.N; i++ {
Div(rand.Int(), rand.Int())
}
}
Go 中单元测试好简单, 特殊一点要求就是命名, 总结起来有下面几小点
1. 文件命必须是 *_test.go
2. 测试函数 func Test*(t *testing.T) { ... }
3. 性能测试函数 func Benchmark*(b *testing.B) { for i := 0; i < b.N; i++ { ... } }
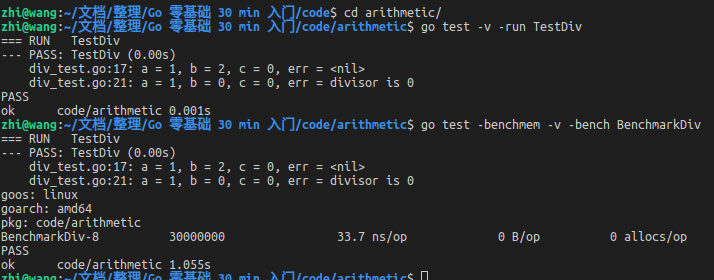
有测试比没有要好很多 /(ㄒoㄒ)/~~
5. Go debug 调试
别深究
深究
就是 F5 F10 F11 go -race
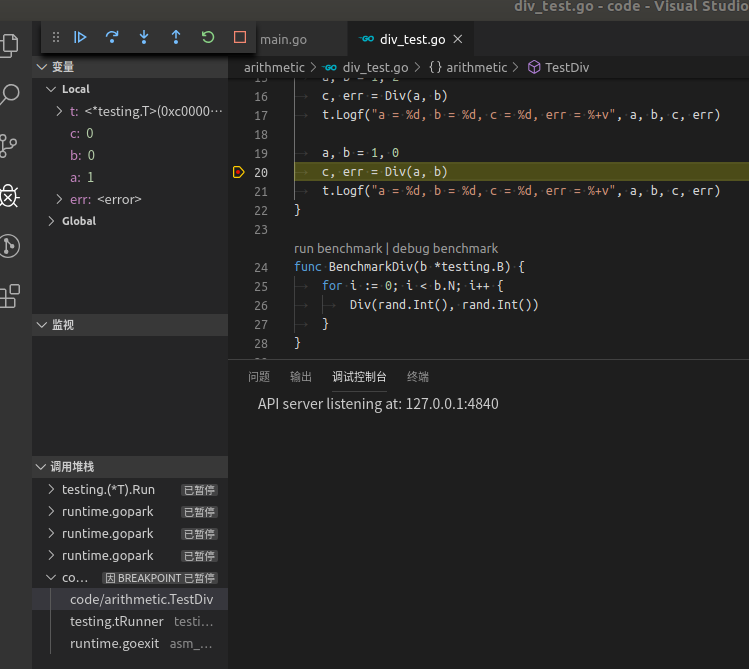
6. Go pprof 火焰图
筹备素材
package main
import (
"fmt"
"log"
"time"
"net/http"
_ "net/http/pprof"
"code/arithmetic"
)
func main() {
println("Hello, 世界")
c, err := arithmetic.Div(1, 0)
fmt.Printf("c = %d, err = %+v\n", c, err)
for i := 0; i < 1000000; i++ {
go func() {
time.Sleep(time.Second * 10)
}()
}
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {})
err = http.ListenAndServe(":8088", nil)
if err != nil {
log.Fatalf("ListenAndServe: %+v\n", err)
}
}
启动服务, 查看程序运行状态
go pprof debug http://127.0.0.1:8088/debug/pprof/
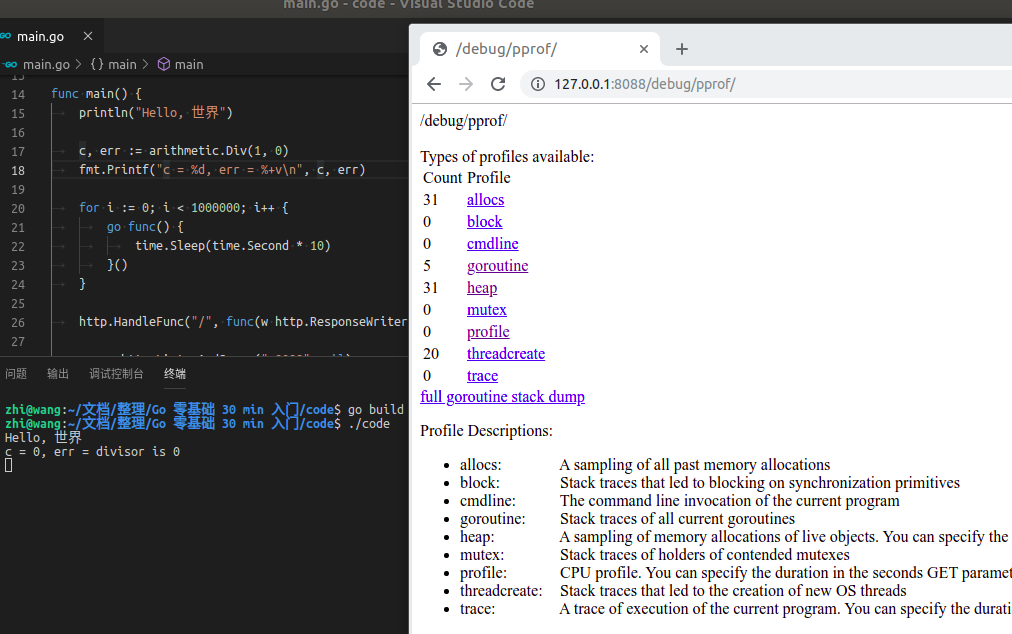
更加详细的可以一块查看一下 cpu 运行火焰图
go tool pprof http://127.0.0.1:8088/debug/pprof/profile -seconds 10
随后会生成一个 profile 相关文件, 用工具打开 pprof.code.samples.cpu.001.pb.gz
go tool pprof -http=:8081 /home/zhi/pprof/pprof.code.samples.cpu.001.pb.gz
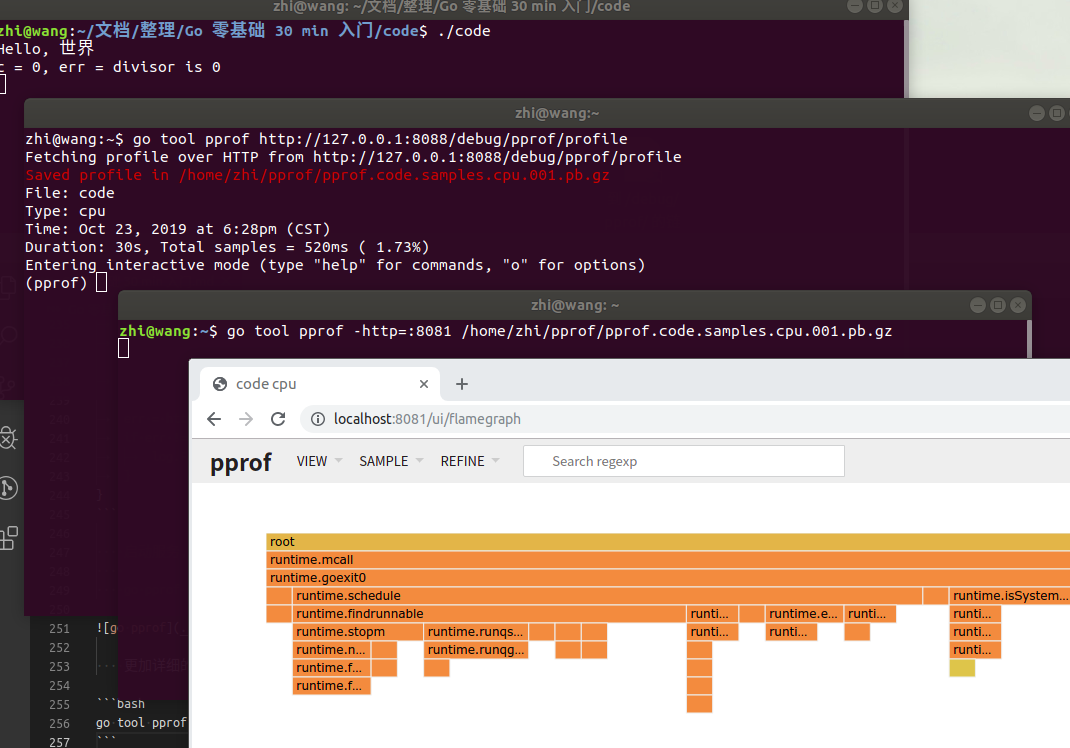
全有了, 是否如虎添翼不知道, 但知道, 你应对问题的方式方法会多一些.
7. Go online 调试
delve https://github.com/go-delve/delve
抛开看日志, 也可以在服务摘除后, 通过 delve 进行 b r n c ...
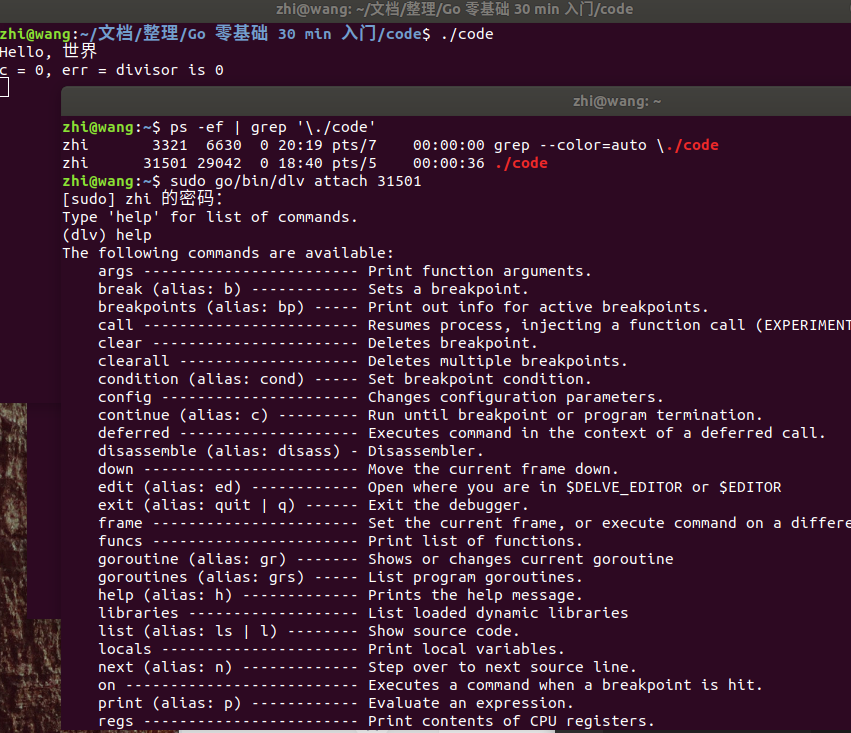
佛度有缘人
8. Go future 思考
Go 许多理念都很普通, 实现方面也很取舍, 没想到最后居然如此的实在, 开发工程杠杠的.
个人觉得其一大亮点是为实际生产的工程师服务, 其次为机器服务, 合理的解决二者的痛点.
关于 Go 未来的思考, 很期待泛型模式的到来.