给定一个数字字符串 S,比如 S = "123456579",我们可以将它分成斐波那契式的序列 [123, 456, 579]。
形式上,斐波那契式序列是一个非负整数列表 F,且满足:
0 <= F[i] <= 2^31 - 1,(也就是说,每个整数都符合 32 位有符号整数类型);
F.length >= 3;
对于所有的0 <= i < F.length - 2,都有 F[i] + F[i+1] = F[i+2] 成立。
另外,请注意,将字符串拆分成小块时,每个块的数字一定不要以零开头,除非这个块是数字 0 本身。
返回从 S 拆分出来的所有斐波那契式的序列块,如果不能拆分则返回 []。
示例 1:
输入:"123456579"
输出:[123,456,579]
示例 2:
输入: "11235813"
输出: [1,1,2,3,5,8,13]
示例 3:
输入: "112358130"
输出: []
解释: 这项任务无法完成。
示例 4:
输入:"0123"
输出:[]
解释:每个块的数字不能以零开头,因此 "01","2","3" 不是有效答案。
示例 5:
输入: "1101111"
输出: [110, 1, 111]
解释: 输出 [11,0,11,11] 也同样被接受。
提示:
1 <= S.length <= 200
字符串 S 中只含有数字。
扫描二维码关注公众号,回复:
7420739 查看本文章
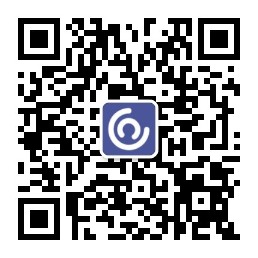
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/split-array-into-fibonacci-sequence
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
代码有点儿烂,做了好久,超过人数才5点几,真TM fuck。
1 public class Solution { 2 private String s; 3 private int len = -1; 4 private List<Integer> res; 5 private int e1; 6 private int e2; 7 private int e3; 8 9 private String isContinue(int m,int n,int o){ 10 String r = null; 11 String a = s.substring(m, n); 12 String b = s.substring(n, o); 13 if (a.length() > 10 || b.length() > 10) 14 return ""; 15 long a0 = Long.parseLong(a); 16 long b0 = Long.parseLong(b); 17 if (a0 > 2147483647 || b0 > 2147483647) 18 return ""; 19 e1 = (int)a0; 20 e2 = (int)b0; 21 if (a0+b0 > 2147483647) 22 return ""; 23 e3 = (int)(a0+b0); 24 String c = String.valueOf(a0 + b0); 25 if (a.charAt(0)=='0' && a.length()!=1 || b.charAt(0)=='0' && b.length() != 1) 26 r = ""; 27 else if (c.length() > len-o) 28 r = ""; 29 else if (!s.substring(o,o+c.length()).equals(c)) 30 r = ""; 31 return (r != null) ? "" : c; 32 } 33 34 private boolean helper(int m, int n, int o){ 35 if (isContinue(m,n,o).equals("")){ 36 return false; 37 } 38 39 for (int i = n; i < len; i++) { 40 for (int j = i+1; j < len; j++) { 41 String c = isContinue(m, i, j); 42 if (c.equals("")) 43 continue; 44 if (s.substring(j,j+c.length()).equals(c)){ 45 if (c.length() > len-j) 46 return false; 47 48 res.add(e1); 49 50 if (c.length() == len-j) { 51 if (e2 == e1 || res.get(res.size()-1) != e2) 52 res.add(e2); 53 res.add(e3); 54 return true; 55 } 56 if (helper(i, j, j+c.length())) 57 return true; 58 59 res.remove(res.size()-1); 60 } 61 } 62 } 63 return false; 64 } 65 public List<Integer> splitIntoFibonacci(String S) { 66 s = S; 67 len = s.length(); 68 res = new ArrayList<>(); 69 70 for (int i = 1; i < len; i++) { 71 for (int j = i+1; j < len; j++) { 72 if (helper(0,i,j)) 73 return res; 74 } 75 } 76 return res; 77 } 78 79 public static void main(String[] args) { 80 List<Integer> integers = new Solution().splitIntoFibonacci("3611537383985343591834441270352104793375145479938855071433500231900737525076071514982402115895535257195564161509167334647108949738176284385285234123461518508746752631120827113919550237703163294909"); 81 System.out.println("integers = " + integers); 82 } 83 }