一、测试用例的依赖关系--->(dependsOnMethods = {"依赖方法名"})
1、在实现自动化的过程中,有些测试用例必须在其它测试用例执行之后才能运行,两者之间存在一定依赖关系。
2、案例演示场景:
testMethod1需要依赖testMethod2执行后才能顺利执行,而testMethod2需要依赖testMethod3执行完成后才能继续执行;
testMethod4是独立的testcase。
1 package testclasses; 2 3 import org.testng.annotations.AfterClass; 4 import org.testng.annotations.BeforeClass; 5 import org.testng.annotations.Test; 6 7 public class TestNG_DependentTests { 8 9 /* 10 * 场景:testMethod1需要依赖testMethod2执行后才能顺利执行,而testMethod2需要依赖testMethod3执行完成后才能继续执行; 11 * testMethod4是独立的testcase。 12 */ 13 14 @Test(dependsOnMethods = {"testMethod2"}) 15 public void testMethod1() { 16 System.out.println("testMethod1"); 17 } 18 19 @Test 20 public void testMethod2() { 21 System.out.println("testMethod2"); 22 } 23 24 @Test(dependsOnMethods = {"testMethod1"}) 25 public void testMethod3() { 26 System.out.println("testMethod3"); 27 } 28 29 @Test 30 public void testMethod4() { 31 System.out.println("testMethod4"); 32 } 33 34 @BeforeClass 35 public void beforeClass() { 36 System.out.println("BeforeClass"); 37 } 38 39 @AfterClass 40 public void afterClass() { 41 System.out.println("AfterClass"); 42 } 43 }
运行结果:
从运行结果可以看出testMethod1需要依赖testMethod2,所以在testMethod1执行前,testMethod2就先执行了,但testMethod1执行完成后,testMethod3才开始执行。而testMethod4因为是独立的,不需要依赖任何的testMethod,所以在testMethod2执行完成后就立即执行了。
3、如果在运行代码的过程中,被依赖的方法出现了错误,那么其它需要依赖该方法的方法就会t跳过不执行,如果需要被执行就在注解后面加上alwaysRun=true。
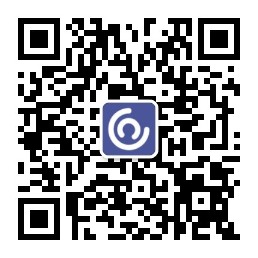
二、禁用测试方法
1、如果需要禁止某个test执行,在注解后面加上(enabled=false)即可。
1 package testclasses; 2 3 import org.testng.annotations.AfterClass; 4 import org.testng.annotations.BeforeClass; 5 import org.testng.annotations.Test; 6 7 public class TestNG_EnableTimeout { 8 9 // Enable=false:带有次属性的方法不会执行 10 @Test(enabled=false) 11 public void testMethod1() { 12 System.out.println("testMethod3"); 13 } 14 15 @Test 16 public void testMethod2() { 17 System.out.println("testMethod4"); 18 } 19 20 @BeforeClass 21 public void beforeClass() { 22 System.out.println("Before Class"); 23 } 24 25 @AfterClass 26 public void afterClass() { 27 System.out.println("After Class"); 28 } 29 }
运行结果:从运行结果可知testMethod1因为加上了(enabled=false),因为未执行,所以在结果中没有显示。
四、让测试方法超时
1、如果我们对test运行时间有要求,那么可以通过在注解后面加上(timeOut=100)进行设置,如果被设置的test在timeOut设置的时间内未运行完成就会立即失败并停止运行。
timeOut=100表示对test设置的限制时间为100毫秒,超过则失败。
1 package testclasses; 2 3 import org.testng.annotations.AfterClass; 4 import org.testng.annotations.BeforeClass; 5 import org.testng.annotations.Test; 6 7 public class TestNG_EnableTimeout { 8 9 // Enable=false:带有此属性的方法不会执行 10 // Enable=true:带有此属性的方法会执行 11 @Test(enabled=true) 12 public void testMethod1() { 13 System.out.println("testMethod3"); 14 } 15 16 @Test(timeOut=10) 17 public void testMethod2() throws InterruptedException { 18 System.out.println("testMethod4"); 19 Thread.sleep(20); 20 } 21 22 @BeforeClass 23 public void beforeClass() { 24 System.out.println("Before Class"); 25 } 26 27 @AfterClass 28 public void afterClass() { 29 System.out.println("After Class"); 30 } 31 }
运行结果:testMethod2设置了强制等待20毫秒,而注解中设置的超时时间为10秒,因此testMethod2执行失败。
五、维持测试用例的执行顺序
1、案例场景:我们有2个测试类都需要执行,分别取名为TestNG_Preserve1和TestNG_Preserve2,但是TestNG_Preserve2要在TestNG_Preserve1执行前先执行。
2、TestNG_Preserve1的代码为:
1 package testclasses; 2 3 import org.testng.annotations.Test; 4 5 public class TestNG_Preserve1 { 6 7 @Test 8 public void testMethod1() { 9 System.out.println("TestNG_Preserve1----->testMethod1"); 10 } 11 12 @Test 13 public void testMethod2() throws InterruptedException { 14 System.out.println("TestNG_Preserve1----->testMethod2"); 15 } 16 }
3、TestNG_Preserve2的代码为:
1 package testclasses; 2 3 import org.testng.annotations.Test; 4 5 public class TestNG_Preserve2 { 6 7 @Test 8 public void testMethod1() { 9 System.out.println("TestNG_Preserve2----->testMethod1"); 10 } 11 12 @Test 13 public void testMethod2() throws InterruptedException { 14 System.out.println("TestNG_Preserve2----->testMethod2"); 15 } 16 }
4、控制test类的执行顺序我们需要用到XML文件,配置如下:
1 <!-- 没有此行配置运行时会报错 --> 2 <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" > 3 <suite name="Preserve Enable TestSuite"> 4 <!-- preserve-order用于设置test中的class是否按照顺序执行,如果为false测按照类名字母的原有顺序执行,为true就按照书写的顺序执行 --> 5 <test 6 name="Test 1" 7 preserve-order="true"> 8 <classes> 9 <class name="testclasses.TestNG_Preserve2"></class> 10 <class name="testclasses.TestNG_Preserve1"></class> 11 </classes> 12 </test> 13 <!-- enabled用于控制该test是否执行,默认为true执行,为false不执行 --> 14 <test 15 name="Test 2" 16 enabled="false"> 17 <classes> 18 <class name="testclasses.TestNG_Preserve2"></class> 19 <class name="testclasses.TestNG_Preserve1"></class> 20 </classes> 21 </test> 22 </suite> 23 <!-- 预期执行结果:Test1中TestNG_Preserve2先执行,然后执行TestNG_Preserve2,Test2中的代码因为被设置为enable为false,因此不会执行 -->
执行结果:预期结果和实际结果一致
如果有不明白的小伙伴可以加群“555191854”问我,群里都是软件行业的小伙伴相互一起学习。
内容具有连惯性,未标注的地方可以看前面的博客,这是一整套关于ava+selenium自动化的内容,从java基础开始。
欢迎关注,转载请注明来源。