输入字符串为二叉树的先根遍历(Pre-order string),可采用堆栈或者递归。
一般pre-order 字符串计算使用递归, post-order 字符串计算使用堆栈
Description
WFF 'N PROOF is a logic game played with dice. Each die has six faces representing some subset of the possible symbols K, A, N, C, E, p, q, r, s, t. A Well-formed formula (WFF) is any string of these symbols obeying the following rules:
p, q, r, s, and t are WFFs
if w is a WFF, Nw is a WFF
if w and x are WFFs, Kwx, Awx, Cwx, and Ewx are WFFs.
The meaning of a WFF is defined as follows:
p, q, r, s, and t are logical variables that may take on the value 0 (false) or 1 (true).
K, A, N, C, E mean and, or, not, implies, and equals as defined in the truth table below. Definitions of K, A, N, C, and E
w x Kwx Awx Nw Cwx Ewx
1 1 1 1 0 1 1
1 0 0 1 0 0 0
0 1 0 1 1 1 0
0 0 0 0 1 1 1
A tautology is a WFF that has value 1 (true) regardless of the values of its variables. For example, ApNp is a tautology because it is true regardless of the value of p. On the other hand, ApNq is not, because it has the value 0 for p=0, q=1.
You must determine whether or not a WFF is a tautology.
Input
Input consists of several test cases. Each test case is a single line containing a WFF with no more than 100 symbols. A line containing 0 follows the last case.
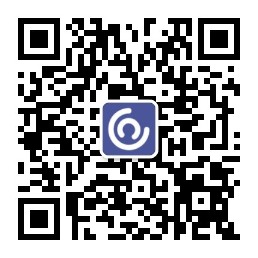
Output
For each test case, output a line containing tautology or not as appropriate.
Sample Input
ApNp
ApNq
0
Sample Output
tautology
not
Source
Waterloo Local Contest, 2006.9.30
//
#include < iostream >
#include < string >
using namespace std;
static int pos = - 1 ;
bool WFF( const string & formula, int i)
{
++ pos;
switch (formula[pos])
{
case ' p ' :
return i & 1 ;
case ' q ' :
return (i >> 1 ) & 1 ;
case ' r ' :
return (i >> 2 ) & 1 ;
case ' s ' :
return (i >> 3 ) & 1 ;
case ' t ' :
return (i >> 4 ) & 1 ;
case ' N ' :
return ! WFF(formula, i);
case ' K ' :
return WFF(formula, i) & WFF(formula, i);
case ' A ' :
return WFF(formula, i) | WFF(formula, i);
case ' C ' :
return ! WFF(formula, i) | WFF(formula, i);
case ' E ' :
return WFF(formula, i) == WFF(formula, i);
}
return false ;
};
bool isTautology( string formula)
{
for ( int i = 0 ; i < 32 ; ++ i)
{
pos = - 1 ;
if (WFF(formula, i) == false ) return false ;;
}
return true ;
};
int main( int argc, char * argv[])
{
string ln;
while (cin >> ln && ln[ 0 ] != ' 0 ' )
{
if (isTautology(ln)) cout << " tautology\n " ;
else cout << " not\n " ;
}
return 0 ;
}
转载于:https://www.cnblogs.com/asuran/archive/2009/09/28/1575379.html