//二叉树的先序遍历(递归)
void PreOrder(BtNode *ptr)
{
if(ptr != NULL)
{
printf("%c ",ptr->data);
PreOrder(ptr->leftchild);
PreOrder(ptr->rightchild);
}
}
//二叉树中序遍历(递归)
void InOrder(BtNode *ptr){
if(ptr != NULL)
{
InOrder(ptr->leftchild);
printf("%c ",ptr->data);
InOrder(ptr->rightchild);
}
}
//二叉树后序遍历(递归)
void PastOrder(BtNode *ptr){
if(ptr != NULL)
{
PastOrder(ptr->leftchild);
PastOrder(ptr->rightchild);
printf("%c ",ptr->data);
}
}
//先序遍历二叉树(非递归)
void NicePastOrder(BtNode *ptr)
{
if(ptr == NULL) return ;
Stack st; // BtNode *; //定义了一个栈
Init_Stack(&st); //初始化这个栈
BtNode *tag = NULL;//做一个标记,用来标记已经走过的
while(ptr != NULL || !empty(&st))
{
while(ptr != NULL)
{
push(&st,ptr);
ptr = ptr->leftchild;
}
ptr = top(&st); pop(&st);
if(ptr->rightchild == NULL || ptr->rightchild == tag)
{
printf("%c ",ptr->data);
tag = ptr;
ptr = NULL;
}
else
{
push(&st,ptr);
ptr = ptr->rightchild;
}
}
}
//利用前序和中序创建树
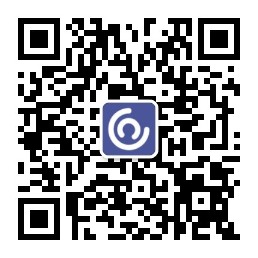
//1.前序的第一个字母为树的根节点
//2.然后查看中序序列中这个字母的位置,它之前的为左子树,之后的为右子树
//3.然后分别对这两个子树的前序和中序序列做同样的步骤即可。
BtNode *Create1(char *ps,char *is,int n)
{
BtNode *s=NULL;
if(n>0)
{
s=Buynode();
s->data =ps[0];
int pos=FindIs(is,n,ps[0]);
if(pos==-1)
{
exit(1);
}
s->leftchild =Create1(ps+1,is,pos);
s->rightchild =Create1(ps+pos+1,is+pos+1,n-pos-1);
}
return s;
}
BtNode * CreatePI(char *ps,char *is)
{
if(ps==NULL||is==NULL)
{
return NULL;
}
else
{
int n=strlen(ps);
return Create(ps,is,n);
}
}
//利用中序和后序,创建树
//1.后序的最后一个字母为树的根节点
//2.然后查看中序序列中这个字母的位置,它之前的为左子树,之后的为右子树
//3.然后分别对这两个子树的中序和后序序列做同样的步骤即可。
BtNode *Create2(char *is,char *ls,int n)
{
BtNode *p=NULL;
if(n>0)
{
p=Buynode();
p->data =ls[n-1];
int pos = FindIs(is,n,ls[n-1]);
if(pos==-1)
{
exit(1);
}
p->leftchild =Create2(is,ls,pos);
p->rightchild =Create2(is+pos+1,ls+pos,n-pos-1);
}
return p;
}
BtNode * CreateIL(char *is,char *ls)
{
if(is==NULL||ls==NULL||n<1)
{
return NULL;
}
int n=strlen(ls);
return Create2(is,ls,n);
}
//在二叉树中查找值x
BtNode * FindValue(BtNode *ptr,ElemType x)
{
ptr=root;
if(ptr==NULL ||ptr==x)
{
return ptr;
}
else
{
BtNode *p=FindValue(ptr->leftchild ,x);
if(p==NULL)
{
p=FindValue(ptr->rightchild ,x);
}
return p;
}
}
//知道孩子结点,找到母亲结点
BtNode * Parent(BtNode *ptr,BtNode *child)
{
if(ptr==NULLL||ptr->leftchild ==NULL||ptr->rightchild ==NULL)
{
return ptr;
}
else
{
BtNode *p=Parent(p->leftchild ,child);
if(p==NULL)
{
p=Parent(p->rightchild ,child);
}
return ptr;
}
}
BtNode *FindParent(char *ptr,char *child)
{
if(ptr==NULL||ptr==child||child==NULL)
{
return NULL;
}
else
{
return Parent(ptr,child);
}
}
//求二叉树的大小
int Size(BtNode *ptr)
{
if(ptr==NULL)
{
return 0;
}
else
{
return Size(ptr->leftchild )+Size(ptr->rightchild )+1;
}
}
//求二叉树的深度
int Max(int x,int y)
{
return x>y? x:y ;
}
int Depth(BtNode *ptr)
{
if(ptr==NULL)
{
return 0;
}
else
{
return Max(Depth(ptr->leftchild ),Depth(ptr->rightchild ))+1;
}
}
//求叶子结点的个数
int SizeLeaf(BtNode *ptr)
{
if(ptr==NULL)
{
return 0;
}
else if(ptr->leftchild ==NULL && ptr->rightchild ==NULL)
{
return 1;
}
else
{
return SizeLeaf(ptr->leftchild )+SizeLeaf(ptr->rightchild );
}
}
//二叉树的先序遍历(递归)
void PreOrder(BtNode *ptr)
{
if(ptr != NULL)
{
printf("%c ",ptr->data);
PreOrder(ptr->leftchild);
PreOrder(ptr->rightchild);
}
}
//二叉树中序遍历(递归)
void InOrder(BtNode *ptr){
if(ptr != NULL)
{
InOrder(ptr->leftchild);
printf("%c ",ptr->data);
InOrder(ptr->rightchild);
}
}
//二叉树后序遍历(递归)
void PastOrder(BtNode *ptr){
if(ptr != NULL)
{
PastOrder(ptr->leftchild);
PastOrder(ptr->rightchild);
printf("%c ",ptr->data);
}
}
//先序遍历二叉树(非递归)
void NicePastOrder(BtNode *ptr)
{
if(ptr == NULL) return ;
Stack st; // BtNode *; //定义了一个栈
Init_Stack(&st); //初始化这个栈
BtNode *tag = NULL;//做一个标记,用来标记已经走过的
while(ptr != NULL || !empty(&st))
{
while(ptr != NULL)
{
push(&st,ptr);
ptr = ptr->leftchild;
}
ptr = top(&st); pop(&st);
if(ptr->rightchild == NULL || ptr->rightchild == tag)
{
printf("%c ",ptr->data);
tag = ptr;
ptr = NULL;
}
else
{
push(&st,ptr);
ptr = ptr->rightchild;
}
}
}
//利用前序和中序创建树
//1.前序的第一个字母为树的根节点
//2.然后查看中序序列中这个字母的位置,它之前的为左子树,之后的为右子树
//3.然后分别对这两个子树的前序和中序序列做同样的步骤即可。
BtNode *Create1(char *ps,char *is,int n)
{
BtNode *s=NULL;
if(n>0)
{
s=Buynode();
s->data =ps[0];
int pos=FindIs(is,n,ps[0]);
if(pos==-1)
{
exit(1);
}
s->leftchild =Create1(ps+1,is,pos);
s->rightchild =Create1(ps+pos+1,is+pos+1,n-pos-1);
}
return s;
}
BtNode * CreatePI(char *ps,char *is)
{
if(ps==NULL||is==NULL)
{
return NULL;
}
else
{
int n=strlen(ps);
return Create(ps,is,n);
}
}
//利用中序和后序,创建树
//1.后序的最后一个字母为树的根节点
//2.然后查看中序序列中这个字母的位置,它之前的为左子树,之后的为右子树
//3.然后分别对这两个子树的中序和后序序列做同样的步骤即可。
BtNode *Create2(char *is,char *ls,int n)
{
BtNode *p=NULL;
if(n>0)
{
p=Buynode();
p->data =ls[n-1];
int pos = FindIs(is,n,ls[n-1]);
if(pos==-1)
{
exit(1);
}
p->leftchild =Create2(is,ls,pos);
p->rightchild =Create2(is+pos+1,ls+pos,n-pos-1);
}
return p;
}
BtNode * CreateIL(char *is,char *ls)
{
if(is==NULL||ls==NULL||n<1)
{
return NULL;
}
int n=strlen(ls);
return Create2(is,ls,n);
}
//在二叉树中查找值x
BtNode * FindValue(BtNode *ptr,ElemType x)
{
ptr=root;
if(ptr==NULL ||ptr==x)
{
return ptr;
}
else
{
BtNode *p=FindValue(ptr->leftchild ,x);
if(p==NULL)
{
p=FindValue(ptr->rightchild ,x);
}
return p;
}
}
//知道孩子结点,找到母亲结点
BtNode * Parent(BtNode *ptr,BtNode *child)
{
if(ptr==NULLL||ptr->leftchild ==NULL||ptr->rightchild ==NULL)
{
return ptr;
}
else
{
BtNode *p=Parent(p->leftchild ,child);
if(p==NULL)
{
p=Parent(p->rightchild ,child);
}
return ptr;
}
}
BtNode *FindParent(char *ptr,char *child)
{
if(ptr==NULL||ptr==child||child==NULL)
{
return NULL;
}
else
{
return Parent(ptr,child);
}
}
//求二叉树的大小
int Size(BtNode *ptr)
{
if(ptr==NULL)
{
return 0;
}
else
{
return Size(ptr->leftchild )+Size(ptr->rightchild )+1;
}
}
//求二叉树的深度
int Max(int x,int y)
{
return x>y? x:y ;
}
int Depth(BtNode *ptr)
{
if(ptr==NULL)
{
return 0;
}
else
{
return Max(Depth(ptr->leftchild ),Depth(ptr->rightchild ))+1;
}
}
//求叶子结点的个数
int SizeLeaf(BtNode *ptr)
{
if(ptr==NULL)
{
return 0;
}
else if(ptr->leftchild ==NULL && ptr->rightchild ==NULL)
{
return 1;
}
else
{
return SizeLeaf(ptr->leftchild )+SizeLeaf(ptr->rightchild );
}
}