Given two rectangles, find if the given two rectangles overlap or not.
Example
Example 1:
Input : l1 = [0, 8], r1 = [8, 0], l2 = [6, 6], r2 = [10, 0]
Output : true
Example 2:
Input : l1 = [0, 8], r1 = [8, 0], l2 = [9, 6], r2 = [10, 0]
Output : false
Notice
l1: Top Left coordinate of first rectangle.
r1: Bottom Right coordinate of first rectangle.
l2: Top Left coordinate of second rectangle.
r2: Bottom Right coordinate of second rectangle.
l1 != r2 and l2 != r2
分析:一开始想的是让其中一个rectangle的某个顶点放在另一个rectangle里。这里忽略了一个下图所示case。所以这题的关键点是求重叠部分,然后看所求出的重叠部分是否valid。
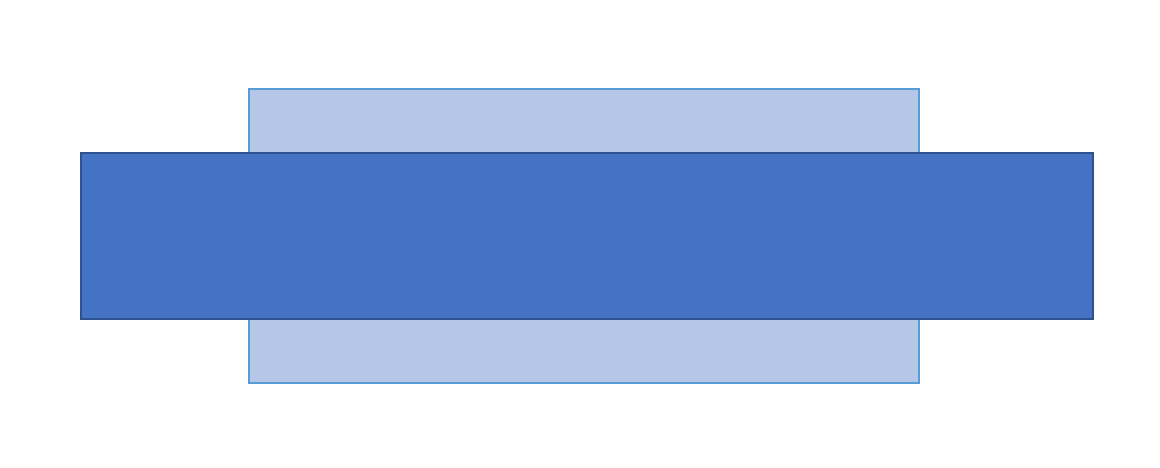
⚠️注意edge case!!!
public class Solution { /** * @param l1: top-left coordinate of first rectangle * @param r1: bottom-right coordinate of first rectangle * @param l2: top-left coordinate of second rectangle * @param r2: bottom-right coordinate of second rectangle * @return: true if they are overlap or false */ public boolean doOverlap(Point l1, Point r1, Point l2, Point r2) { int x1 = Math.max(l1.x, l2.x); int x2 = Math.min(r1.x, r2.x); int y1 = Math.max(r1.y, r2.y); int y2 = Math.min(l1.y, l2.y); if (x2 >= x1 && y2 >= y1) { return true; } else { return false; } } }