https://start.spring.io/ 生成SpringBoot项目
pom文件应该是我这样的:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.20.RELEASE</version> <relativePath /> <!-- lookup parent from repository --> </parent> <groupId>org.dreamtech</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>demo</name> <description>Demo project for Spring Security</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- 在IDEA中如果项目运行失败,注释掉这一项即可 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Controller:
package org.dreamtech.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.EnableAutoConfiguration; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.security.access.prepost.PreAuthorize; import org.springframework.security.config.annotation.method.configuration.EnableGlobalMethodSecurity; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; /** * Spring Boot启动类 * @author Xu Yiqing * */ @SpringBootApplication @RestController @EnableAutoConfiguration @EnableGlobalMethodSecurity(prePostEnabled = true) public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } /** * 根目录,所有人都可以访问 * @return */ @RequestMapping("/") public String helloSpringBoot() { return "hello spring boot"; } /** * 只有经过身份认证后才可以访问 * @return */ @RequestMapping("/hello") public String helloWorld() { return "hello world"; } /** * 经过身份认证且身份必须是ADMIN才可以访问,并且是在方法执行前进行验证 * @return */ @PreAuthorize("hasRole('ROLE_ADMIN')") @RequestMapping("/role") public String role() { return "admin auth"; } }
Spring Security配置文件:
package org.dreamtech.demo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.builders.WebSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; /** * Spring Security配置文件 * @author Xu Yiqing * */ @Configuration @EnableWebSecurity public class SpringSecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private MyUserService myUserService; @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { /* 可以将用户名密码存在内存中,也可以采用自定义Service从数据库中取 auth.inMemoryAuthentication().withUser("admin").password("12345").roles("ADMIN"); auth.inMemoryAuthentication().withUser("test").password("test").roles("USER"); */ auth.userDetailsService(myUserService).passwordEncoder(new MyPasswordEncoder()); } @Override protected void configure(HttpSecurity http) throws Exception { // 配置对根路径放行,其他请求拦截,对logout放行,允许表单校验,禁用CSRF http.authorizeRequests().antMatchers("/").permitAll().anyRequest().authenticated().and().logout().permitAll() .and().formLogin(); http.csrf().disable(); } @Override public void configure(WebSecurity web) throws Exception { // 配置忽略js、css、images静态文件 web.ignoring().antMatchers("/js/**", "/css/**", "/images/**"); } }
自定义密码加密器:
package org.dreamtech.demo; import org.springframework.security.authentication.encoding.Md5PasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; /** * 自定义密码加密器 * * @author Xu Yiqing * */ @SuppressWarnings("deprecation") public class MyPasswordEncoder implements PasswordEncoder { // 加密需要的盐 private static final String SALT = "666"; /** * 加密 */ @Override public String encode(CharSequence rawPassword) { Md5PasswordEncoder encoder = new Md5PasswordEncoder(); return encoder.encodePassword(rawPassword.toString(), SALT); } /** * 匹配 */ @Override public boolean matches(CharSequence rawPassword, String encodedPassword) { Md5PasswordEncoder encoder = new Md5PasswordEncoder(); return encoder.isPasswordValid(encodedPassword, rawPassword.toString(), SALT); } }
扫描二维码关注公众号,回复:
5982420 查看本文章
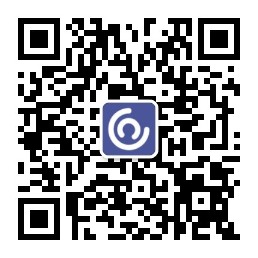
Service:
package org.dreamtech.demo; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.core.userdetails.UsernameNotFoundException; import org.springframework.stereotype.Component; /** * 自定义服务 * @author Xu Yiqing * */ @Component public class MyUserService implements UserDetailsService { /** * 从DAO层根据用户名查询 */ @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { UserDetails userDetails = null; // DAO操作 ...... return userDetails; } }
这里的Service层只是做一个示例
如果想看具体的效果,应该使用configure中注释掉的那两行进行测试
总结:
Spring Security优点:功能较齐全,高度兼容Spring
Spring Security缺点:体系庞大,配置繁琐,不够直观
所以,在实际开发中,人们通常选用Apache Shiro代替Spring Security