这个是SMS Cat设备:
需要插入SIM卡,所以是需要付短信费的。
插好USB和电源后,会安装好驱动,可以在设备管理可以查看到端口号
也有一种软件可以测试端口是否可连接:secureCRT
新建好connection后,输入AT测试,如果OK就OK。
扫描二维码关注公众号,回复:
5970693 查看本文章
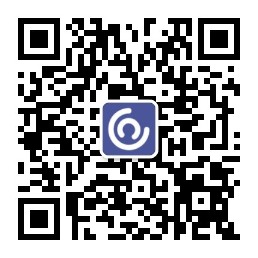
好了,端口确定没问题了。
接着,在你的JDK的bin路径下放一个win32com.dll
在JDK的lib中放一个comm.jar和javax.comm.properties
需要的文件都在附件SMSCat.rar中。
OK,所有都准备完了,现在开始代码测试。
demo测试需要用到的jar
sms.properties#sms properties
- Message.comId=modem.com19
- Message.com=COM19
- Message.baudRate=9600
- Message.manufacturer=wavecom
- Message.model=
- Message.simPin=0000
注意:这里的端口COM19必须和你上面的端口对应。
SMSService.java
- /**
- * Copyright(C) 2012 GZ ISCAS ALL Rights Reserved
- */
- package com.royal.SMSCat;
- import java.util.Properties;
- import org.smslib.Message.MessageEncodings;
- import org.smslib.OutboundMessage;
- import org.smslib.Service;
- import org.smslib.modem.SerialModemGateway;
- import com.royal.utils.PropertiesUtil;
- /**
- * 描述:SMS Cat服务类
- */
- public class SMSService {
- /**
- * 私有静态实例
- */
- private static SMSService instance = null;
- /**
- * 是否开启服务
- */
- private boolean isStartService = false;
- /**
- * 私有构造方法
- */
- private SMSService() {
- }
- /**
- * 获取实例(单例模式)
- *
- * @return
- */
- public static SMSService getInstance() {
- if (instance == null) {
- instance = new SMSService();
- }
- return instance;
- }
- /**
- * 开启短信服务
- *
- * @param path
- * 配置文件路径
- */
- public void startService(String path) {
- System.out.println("开始初始化SMS服务!");
- // 加载文件属性
- Properties p = null;
- try {
- p = PropertiesUtil.getProperties(path);
- } catch (Exception e) {
- System.out.println("加载属性文件出错:" + e.getMessage());
- return;
- }
- // 初始化网关,参数信息依次为:COMID,COM号,比特率,制造商,Modem模式
- SerialModemGateway gateway = new SerialModemGateway(p.getProperty("Message.comId"), p.getProperty("Message.com"), Integer.parseInt(p.getProperty("Message.baudRate")), p.getProperty("Message.manufacturer"), p.getProperty("Message.model"));
- gateway.setInbound(true);
- gateway.setOutbound(true);
- gateway.setSimPin(p.getProperty("Message.simPin"));
- OutboundNotification outboundNotification = new OutboundNotification();
- Service service = Service.getInstance();
- if (service == null) {
- System.out.println("初始化SMS服务失败!");
- return;
- }
- service.setOutboundMessageNotification(outboundNotification);
- try {
- service.addGateway(gateway);
- // 开启服务
- service.startService();
- System.out.println("初始化SMS服务成功!");
- isStartService = true;
- } catch (Exception e) {
- System.out.println("开启SMS服务异常:" + e.getMessage());
- }
- }
- /**
- * 停止SMS服务
- */
- public void stopService() {
- try {
- Service.getInstance().stopService();
- } catch (Exception e) {
- System.out.println("关闭SMS服务异常:" + e.getMessage());
- }
- isStartService = false;
- }
- /**
- * 发送短信
- *
- * @param toNumber
- * 手机号码
- * @param message
- * 短信内容
- */
- public void sendMessage(String toNumber, String message) {
- if (!isStartService) {
- System.out.println("尚未开启SMS服务!");
- return;
- }
- // 封装信息
- OutboundMessage msg = new OutboundMessage(toNumber, message);
- msg.setEncoding(MessageEncodings.ENCUCS2);
- try {
- // 发送信息
- Service.getInstance().sendMessage(msg);
- } catch (Exception e) {
- System.out.println("SMS服务发送信息发生异常:" + e.getMessage());
- isStartService = false;
- }
- }
- }
OutboundNotification.java
- package com.royal.SMSCat;
- import org.smslib.AGateway;
- import org.smslib.IOutboundMessageNotification;
- import org.smslib.OutboundMessage;
- /**
- * 封装发送短信类
- */
- public class OutboundNotification implements IOutboundMessageNotification {
- public void process(AGateway gateway, OutboundMessage msg) {
- System.out.println("Outbound handler called from Gateway: " + gateway.getGatewayId());
- }
- }
SMSCatClient.java
- package com.royal.SMSCat;
- public class SMSCatClient {
- /**
- * 测试
- *
- * @param args
- */
- public static void main(String[] args) {
- String path = "D:\\sms.properties";
- SMSService.getInstance().startService(path);
- SMSService.getInstance().sendMessage("13800138000", "测试 Test!");
- //没必要的时候没停止服务,因为端口占用着
- SMSService.getInstance().stopService();
- }
- }
测试结果自己找个手机号测吧
看见了吗?控制台中的服务(红色标识)还在跑着,也就是端口还在占用着;服务没断,可以不用重新初始化。